ViewPager的作用是让页面滑动。
左右滑动分别显示3个页面
代码
在主布局文件放入一个ViewPager
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
<android.support.v4.view.ViewPager
android:id="@+id/viewpager"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</RelativeLayout>
创建每个可以滑动的页面(创建xml文件)
layout1.xml
扫描二维码关注公众号,回复:
4587440 查看本文章
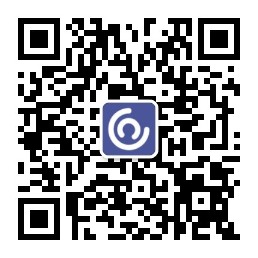
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="第一个页面"
android:layout_centerInParent="true"
android:gravity="center|center_horizontal"
/>
</RelativeLayout>
layout2.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="第二个页面"
android:layout_centerInParent="true"
android:gravity="center|center_horizontal"
/>
</RelativeLayout>
layout3.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="第三个页面"
android:layout_centerInParent="true"
android:gravity="center|center_horizontal"
/>
</RelativeLayout>
MainActivity.java
package com.example.viewpager;
import java.util.ArrayList;
import android.app.Activity;
import android.os.Bundle;
import android.support.v4.view.PagerAdapter;
import android.support.v4.view.ViewPager;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
public class MainActivity extends Activity {
private ViewPager viewPager;
private View view1;
private View view2;
private View view3;
private ArrayList<View> viewList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//获取ViewPager对象
viewPager =(ViewPager)findViewById(R.id.viewpager);
initView();
}
private void initView()
{
//加载布局文件(页卡),并转换为View对象
LayoutInflater lf = getLayoutInflater().from(this);
view1 = lf.inflate(R.layout.layout1, null);
view2 = lf.inflate(R.layout.layout2, null);
view3 = lf.inflate(R.layout.layout3, null);
//将View对象放入数组
viewList = new ArrayList<View>();
viewList.add(view1);
viewList.add(view2);
viewList.add(view3);
//创建适配器对象
ViewPagerAdapter vpa = new ViewPagerAdapter();
//将适配器设置与ViewPager进行绑定
viewPager.setAdapter(vpa);
}
//创建适配器
class ViewPagerAdapter extends PagerAdapter
{
@Override
public void destroyItem(ViewGroup container,int position,Object ibject){
//删除页卡
container.removeView(viewList.get(position));
}
@Override
public Object instantiateItem(ViewGroup container,int position){
//添加页卡
container.addView(viewList.get(position));
return viewList.get(position);
}
@Override
public int getCount() {
//返回页卡的数量
return viewList.size();
}
@Override
public boolean isViewFromObject(View arg0, Object arg1) {
return arg0==arg1;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}