java.lang.Runnable
- 接口
- Runnable中有Public void run();方法
- 供现有Runnable对象创建线程
- 使用Runnable对象创建线程
New Thread(Runnable r).start(); - 静态同步方法是使用class作为锁
- 非静态方法是使用this(当前对象)作为锁
样例
Class car implements Runnable{
…
Static synchronized void xxx(){
//静态同步方法
}
}
New Thread(Runnable r).start();
s.start();
同步(synchronized),格式
synchronized(对象){
需要同步的代码;
}
同步可以解决安全问题的根本原因就在那个对象上,该对象如同锁的功能。
同步的前提条件:
1.同步需要两个或者两个以上的线程。
2.多个线程使用的是同一个锁。
未满足这两个条件不能称为同步。
同步的弊端:
当线程相当多时,因为每个线程都会去判断同步上的锁,这是很耗资源的,无形中会降低程序的运行效率。
停止线程
- 利用循环结束线程
- 使用interrupt(中断)方法
该方法是结束线程的冻结状态,使线程回到运行状态中来。
注:stop方法已经过时,不再使用。
线程类的其他方法
优先级
setPriority(int num) 1<=num<=10
使用方法
xx.setPriority(num);
优先级数越大,则优先运行
setDaemon(boolean b)
join()
自定义线程名称
toString()
线程安全性
synchronized(Object){
…
}
public synchronized void xxx(){
…
}
public static syzchronized void xxx(){
…
}
优先级函数例子
扫描二维码关注公众号,回复:
4531511 查看本文章
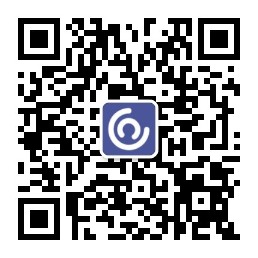
public class t93 {
public static void main(String[] args){
mythread a=new mythread("a");
//使得a的优先级为1
a.setPriority(1);
mythread b=new mythread("b");
//b的优先级为10,打印结果先打印b,后打印a
b.setPriority(10);
a.start();
b.start();
}
}
class mythread extends Thread{
private String name;
public mythread(String name){
this.name=name;
}
public void run(){
System.out.println(name);
}
}
String类
//字符串池中的量
String str=”abc”;
//new String 分配内存空间(堆区)
String str1=new String(“abc”);
字符集编码
//字符串长度,字符的个数
str.length();
//提取指定位置上的字符0<=x<=str.length();
str.charAt(x);
//复制String中的[],产生新的数组,但不会影响原来的数组
char[] arr=str.toCharArray();
//返回子串在母串中的位置(索引值,以0为基址)
int pos =str.indexOf(“world”);
//判断是否以指定的字符开头或者结尾,返回true/false
startsWith(“xxx”);
endsWith(“xxx”);
//切割字符串,形成字符串数组
str.split("xxx");
//调试 debug(抄自ifeng)
F9 resume programe 恢复程序
Alt+F10 show execution point 显示执行断点
F8 Step Over 相当于eclipse的f6 跳到下一步
F7 Step Into 相当于eclipse的f5就是 进入到代码
Alt+shift+F7 Force Step Into 这个是强制进入代码
Shift+F8 Step Out 相当于eclipse的f8跳到下一个断点,也相当于eclipse的f7跳出函数
Atl+F9 Run To Cursor 运行到光标处
ctrl+shift+F9 debug运行java类
ctrl+shift+F10 正常运行java类
alt+F8 debug时选中查看值
样例代码
public class t1 {
public static void main(String[] args) {
String str="hello world";
//串长度,字符的个数
System.out.println(str.length());
//提取指定位置上的字符
System.out.println(str.charAt(10));
//复制String中的[ ],产生新的数组,不会影响原来的数组
char[] arr=str.toCharArray();
System.out.println(arr);
//返回子串在母串中的位置
int pos=str.indexOf("world");
System.out.println(pos);
//判断是否以指定的字符开头或结尾
String s1="syzniubi6";
String s2="ccyniubi7";
String s3="lzxniubi8";
System.out.println(s1.endsWith("6"));
System.out.println(s2.startsWith("lzx"));
//切割字符串,形成字符串组
str="hello world";
String[] strArr=str.split(" ");
System.out.println(strArr.length);
}
}
打印结果