一、手机触屏事件
1.常见的触屏事件:
touchstart:触摸开始时候触发
touchmove:手指在屏幕上滑动的时候触发
touchend:触摸结束的时候触发
touchcancel:当一些更高级别的事件发生的时候(如电话接入或者弹出信息)会取消当前的touch操作,即触发ontouchcancel。一般会在ontouchcancel时暂停游戏、存档等操作。
2.每个触摸事件包含三个触摸列表,每个列表里包含了对应的一系列触摸点:
touches:当前位于屏幕上的所有手指的列表;//touches[0]、touches[1]....
targetTouches:位于当前DOM元素上手机的列表;
changedTouches:涉及当前事件手指的列表;
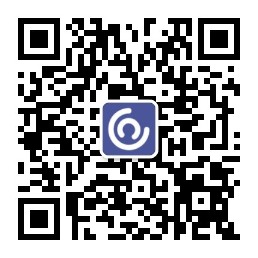
3.每个触摸点(touch对象)包含的触摸信息:
clientX/clientY:触摸目标在可视窗口中的X/Y坐标;
pageX/pageY:触摸目标在页面中的x/y坐标;
screenX/screenY:触摸目标在屏幕中的x/y坐标;
identifier:表示触摸的唯一ID;
target:触摸的DOM节点坐标;
注意:手机事件添加只能使用监听添加事件;
例子:触屏滑动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title></title>
<style>
*{margin: 0;padding: 0;}
.block{
width: 100%;
height: 300px;
border: 1px solid red;
overflow: hidden;
}
</style>
</head>
<body>
<div class="block"></div>
<script>
//定义坐标
var startx=0;
var starty=0;
var endx=0;
var endy=0;
var block=document.getElementsByClassName("block")[0];
block.addEventListener("touchstart",function(e){
startx=e.touches[0].pageX;
starty=e.touches[0].pageY;
});
block.addEventListener("touchmove",function(e){
endx=e.touches[0].pageX;
endy=e.touches[0].pageY;
});
block.addEventListener("touchend",function(){
if(endx==undefined){
endx=startx;
}else{
if(endx-startx>0){
console.log("右")
}else{
console.log("左")
}
}
endx=0;
})
</script>
</body>
</html>
二、手势事件
手势是指利用多点触控进行旋转、拉伸等操作,例如图片、网页的放大、旋转。需要两个或以上的手指同时触摸时才会触发手势事件。
gesturestart:当一个手指按在屏幕上,另一个手指有触发屏幕时触发;
gestureend:当任何一个手指从屏幕上移开的时候触发;
gesturechange:当触摸屏幕的任何一个手指发生变化的时候触发;
rotation:手指变化引起的旋转角度,值区间[0,360],顺时针为正,逆时针为负;
scale:两个手指之间的距离变化(小于1是缩小,大于1是放大,原始为1 );
Eg:
<script>
var block=document.getElementsByClassName("block")[0];
block.addEventListener("gesturechang",function(e){
var scale=e.scale;
var angle=e.rotation;
})
</script>
三、利用第三方封装重力感应事件之旋转事件
1.orientationchange事件是在用户水平或垂直翻转设备(即每次屏幕方向在横竖屏间切换后)时触发的事件;
在此事件中由window.orientation属性得到代表当前手机方向的数值。
window.orientation值的列表:
0:与页面首次加载时的方向一致;
-90:相对原始方向顺时针旋转了90度;
180:转了180度;
90:逆时针转90度;
Eg:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta id="viewport" name="viewport" content="width=device-width,initial-scale=1.0;">
<title></title>
<style>
*{margin: 0;padding: 0;}
body[orient=landscape]{
background-color: #ff0000;
}
body[orient=portrait]{
background-color: #00ffff;
}
</style>
</head>
<body orient="landspace">
<div>内容</div>
<script>
(function(){
if(window.orient==0){//未旋转
document.body.setAttribute("orient","portrait");
}else{
document.body.setAttribute("orient","lanscape");
}
})();
window.onorientationchange=function(){
var body=document.body;//获取body
var viewport=document.getElementById("viewport");
if(body.getAttribute("orient")=="landscape"){
body.setAttribute("orient","portrait");
}else{
body.setAttribute("orient","landscape")
}
};
</script>
</body>
</html>
注释:
setAttribute() 方法添加指定的属性,并为其赋指定的值。
如果这个指定的属性已存在,则仅设置/更改值。
四、重力感应事件 H5自带事件
1.DeviceMotionEvent事件:提供设备加速的信息,表示为定义在设备上的坐标系中的卡尔迪坐标。其还提供了设备在坐标系中的自转速率;
deviceorientation事件:提供设备物理方向信息,表示为一系列本地坐标系的旋角;
compassneedscalibration 用于通知Web站点使用罗盘信息校准上述事件。
2.用法
(1)注册一个devicemotion事件的接收器:
window.addEventListener("devicemotion", function(event) {
// 处理event.acceleration、event.accelerationIncludingGravity、
// event.rotationRate和event.interval
}, true);
将设备放置在水平表面,屏幕向上,acceleration为零,则其accelerationIncludingGravity信息:{x:0,y:0,z:9.81};
设备做自由落体,屏幕水平向上,accelerationIncludingGravity为零,则其acceleration信息:{x:0,y:0,z:-9,81}
将设备安置于车辆上,屏幕处于一个垂直平面,顶端向上,面向车辆后部。车辆行驶速度为v,向右侧进行半径为r的转弯。设备记录acceleration和accelerationIncludingGravity在位置x处的情况,同时设备还会记录rotationRate.gamma的负值:
{acceleration: {x: v^2/r, y: 0, z: 0},
accelerationIncludingGravity: {x: v^2/r, y: 0, z: 9.81},
rotationRate: {alpha: 0, beta: 0, gamma: -v/r*180/pi} };
3.应用案例:手机简单摇一摇
<script>
if (window.DeviceMotionEvent) {
//添加重力感应处理事件
window.addEventListener('devicemotion',deviceMotionHandler, false);
}
var speed = 30;//speed
var x = y = z = lastX = lastY = lastZ = 0;
//封装重力感应方法
function deviceMotionHandler(event) {
var acceleration =event.accelerationIncludingGravity;
x = acceleration.x;
y = acceleration.y;
z = acceleration.z;
if(Math.abs(x-lastX) > speed || Math.abs(y-lastY) > speed || Math.abs(z-lastZ) > speed) {//Math.abs()取绝对值
//简单的摇一摇触发代码
alert(1);
}
////覆盖上一次摇晃
lastX = x;
lastY = y;
lastZ = z;
}
</script>