rxjava+retrofit
1.添加依赖.
//Retrofit2的依赖
implementation ‘com.squareup.retrofit2:converter-gson:2.0.0-beta4’
compile ‘com.squareup.retrofit2:converter-gson:2.1.0’
//Rxjava依赖
implementation ‘io.reactivex.rxjava2:rxjava:2.1.16’
implementation ‘io.reactivex.rxjava2:rxandroid:2.0.2’
implementation ‘com.squareup.retrofit2:adapter-rxjava2:2.4.0’
2.新建callback接口
import retrofit2.Call;
ic interface CallBack {
void onSuccess(String result);
void onFailer(Call call,Throwable t);
}
import java.util.Map;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.scalars.ScalarsConverterFactory;
public class HttpManager {
public void getMethod(String url, final CallBack callback){
Retrofit retrofit = new Retrofit.Builder().baseUrl("http://api.tianapi.com/nba/")
.addConverterFactory(ScalarsConverterFactory.create())
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build();
ProjectApi projectApi = retrofit.create(ProjectApi.class);
Call<String> call = projectApi.getMethod(url);
call.enqueue(new Callback<String>() {
@Override
public void onResponse(Call<String> call, Response<String> response) {
String body = response.body();
callback.onSuccess(body);
}
@Override
public void onFailure(Call<String> call, Throwable t) {
callback.onFailer(call,t);
}
});
}
public void postMethod(String baseUrl, String url, Map<String,String> map, final CallBack callback){
Retrofit retrofit = new Retrofit.Builder()
.baseUrl(baseUrl)
.addConverterFactory(ScalarsConverterFactory.create())
.build();
ProjectApi projectApi = retrofit.create(ProjectApi.class);
Call<String> call = projectApi.postMethod(url, map);
call.enqueue(new Callback<String>() {
@Override
public void onResponse(Call<String> call, Response<String> response) {
String body = response.body();
callback.onSuccess(body);
}
@Override
public void onFailure(Call<String> call, Throwable t) {
callback.onFailer(call,t);
}
});
}
}
import java.util.Map;
import retrofit2.Call;
import retrofit2.http.FieldMap;
import retrofit2.http.GET;
import retrofit2.http.POST;
import retrofit2.http.Url;
public interface ProjectApi {
@GET
Call getMethod(@Url String url);
@POST
Call<String> postMethod(@Url String url, @FieldMap Map<String,String> map);
}
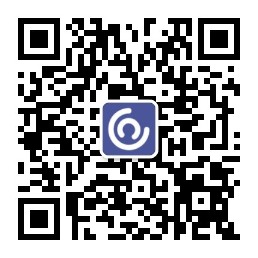
public interface UrlUtil {
public String PATH = “http://api.tianapi.com/nba/”;
public String AA = “?key=71e58b5b2f930eaf1f937407acde08fe&num=10”;
}
public interface RecycleViewV {
void onSuccess(String result);
void onFailer(Call call, Throwable t);
}
public class RecyclePresenter {
private RecycleViewV mRecyclerView;
private HttpManager mHttpManager;
public RecyclePresenter(RecycleViewV recyclerView) {
mRecyclerView = recyclerView;
mHttpManager = new HttpManager();
}
public void urlUtil(String url, Map<String,String> map){
mHttpManager.getMethod(url, new CallBack() {
@Override
public void onSuccess(String result) {
mRecyclerView.onSuccess(result);
}
@Override
public void onFailer(Call<String> call, Throwable t) {
mRecyclerView.onFailer(call,t);
}
});
}
}
public class MainActivity extends AppCompatActivity implements RecycleViewV {
private RecyclerView recycle;
private RecyclePresenter mRecyclePresenter;
private List<User.NewslistBean> list;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recycle = findViewById(R.id.recycle);
Map<String,String> map = new HashMap<>();
mRecyclePresenter = new RecyclePresenter(this);
mRecyclePresenter.urlUtil(UrlUtil.PATH+UrlUtil.AA,map);
}
@Override
public void onSuccess(String result) {
Gson gson = new Gson();
User user = gson.fromJson(result, User.class);
list = user.getNewslist();
recycle.setLayoutManager(new LinearLayoutManager(this));
RecycleAdapter adapter = new RecycleAdapter(getApplicationContext(),list);
recycle.setAdapter(adapter);
}
@Override
public void onFailer(Call<String> call, Throwable t) {
}
}