当我们进行项目开发的时候,往往是需要应用程序的各组件、组件与后台线程间进行通信,比如在子线程中进行请求数据,当数据请求完毕后通过Handler或者是广播通知UI,而两个Fragment之家可以通过Listener进行通信等等。当我们的项目越来越复杂,使用Intent、Handler、Broadcast进行模块间通信、模块与后台线程进行通信时,代码量大,而且高度耦合。现在就让我们来学习一下EventBus ;
EventBus能够简化各组件间的通信,让我们的代码书写变得简单,能有效的分离事件发送方和接收方(也就是解耦的意思),能避免复杂和容易出错的依赖性和生命周期问题。
具体开发步骤
/**
- 1.搭建环境
- 1.1:依赖:01.okHttp , 02:RecyclerView, 03.Fresco ,04.EventBus,
- 1.2:网络权限
- 2.完成布局
- 3.创建适配器
- 4.完成RecycleView数据展示
- 5.使用Fresco定义图片形状,自定义MyApplication
- 6.在清单文件注册MyApplication
- 7.使用自定义接口回调实现条目点击事件
- 8.使用EventBus的黏性事件完成数据传递
*/
1.搭建环境:
依赖:
implementation 'com.squareup.okhttp3:okhttp:3.4.2'
implementation 'com.android.support:recyclerview-v7:28.0.0'
implementation 'com.facebook.fresco:fresco:0.14.1'
implementation 'org.greenrobot:eventbus:3.0.0'
implementation 'com.google.code.gson:gson:2.8.5'
implementation 'com.jakewharton:butterknife:8.8.1'
annotationProcessor 'com.jakewharton:butterknife-compiler:8.8.1'
网络权限:
<uses-permission android:name="android.permission.INTERNET " />
期间我们需要用到Fresco,我们需要自定义MyApplication类,现在来清单文件(AndroidManifest)注册一下
<application
android:name=".MyApplication"
</application>
MyApplication:
package com.example.dsjob;
import android.app.Application;
import com.facebook.drawee.backends.pipeline.Fresco;
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
Fresco.initialize(this);
}
}
2.MainActivity:
package com.example.dsjob;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.OrientationHelper;
import android.support.v7.widget.RecyclerView;
import com.google.gson.Gson;
import org.greenrobot.eventbus.EventBus;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private RecyclerView rlv;
private MyAdapter myAdapter;
private String path = "http://172.17.8.100/movieApi/movie/v1/findHotMovieList?page=1&count=10";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//初始化控件
initView();
//创建适配器
myAdapter = new MyAdapter(MainActivity.this);
//加载网络数据
initData();
rlv.setAdapter(myAdapter);
//设置布局管理器
rlv.setLayoutManager(new LinearLayoutManager(this,OrientationHelper.VERTICAL,false));
myAdapter.setOnClickListener(new MyAdapter.OnClickListener() {
@Override
public void onClick(String name) {
EventBus.getDefault().postSticky(name);
Intent intent = new Intent(MainActivity.this,EventBusActivity.class);
startActivity(intent);
}
});
}
private void initData() {
OkHttpUtils.getInstance().doGet(path, new OkHttpUtils.OkCallback() {
@Override
public void success(String json) {
DataItem dataItem = new Gson().fromJson(json, DataItem.class);
List<DataItem.ResultBean> result = dataItem.getResult();
myAdapter.setData(result);
}
@Override
public void failtrue(Exception e) {
}
});
}
private void initView() {
rlv = (RecyclerView) findViewById(R.id.rlv);
}
}
3.EventBusActivity:
package com.example.dsjob;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import org.greenrobot.eventbus.EventBus;
import org.greenrobot.eventbus.Subscribe;
import org.greenrobot.eventbus.ThreadMode;
/**
* 接收主页面传递过来的数据
*/
public class EventBusActivity extends AppCompatActivity implements View.OnClickListener {
private Button btn_eventbus;
private TextView tv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_event_bus);
initView();
//注册EventBus
EventBus.getDefault().register(this);
}
private void initView() {
btn_eventbus = (Button) findViewById(R.id.btn_eventbus);
btn_eventbus.setOnClickListener(this);
tv = (TextView) findViewById(R.id.tv);
tv.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_eventbus:
finish();
break;
}
}
@Subscribe(threadMode = ThreadMode.MAIN,sticky = true)
public void MessageEventBus(String name) {
tv.setText(name+"");
}
@Override
protected void onDestroy() {
super.onDestroy();
//解除注册EventBus
EventBus.getDefault().unregister(this);
}
}
4.EventBus消息类:
package com.example.dsjob;
/**
* date:2018/11/30
* author:李壮(大壮)
* function:EventBus消息类
*/
public class EventBusMessage {
private String name;
public EventBusMessage(String name) {
this.name = name;
}
}
5.适配器:
package com.example.dsjob;
import android.content.Context;
import android.net.Uri;
import android.support.annotation.NonNull;
import android.support.v7.widget.RecyclerView;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import com.facebook.drawee.generic.GenericDraweeHierarchy;
import com.facebook.drawee.generic.GenericDraweeHierarchyBuilder;
import com.facebook.drawee.generic.RoundingParams;
import com.facebook.drawee.view.SimpleDraweeView;
import java.util.ArrayList;
import java.util.List;
/**
* date:2018/11/30
* author:李壮(大壮)
* function: RecyclerView适配器
* 1.创建Xml布局
* 2.完成展示数据
* 3.自定义接口回调实现条目点击事件
*/
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.ViewHolder> {
private Context mContext;
private List<DataItem.ResultBean> mResultBeans;
public MyAdapter(Context context) {
this.mContext = context;
mResultBeans = new ArrayList<>();
}
public void setData(List<DataItem.ResultBean> result) {
mResultBeans.clear();
if (result != null){
mResultBeans.addAll(result);
}
notifyDataSetChanged();
}
@NonNull
@Override//创建Viewolder
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = View.inflate(mContext,R.layout.rlv_item,null);
ViewHolder viewHolder = new ViewHolder(view);
return viewHolder;
}
@Override//绑定视图
public void onBindViewHolder(@NonNull ViewHolder viewHolder, final int i) {
viewHolder.rlv_title.setText(mResultBeans.get(i).getName());
//获取图片地址
Uri parse = Uri.parse(mResultBeans.get(i).getImageUrl());
GenericDraweeHierarchyBuilder genericDraweeHierarchyBuilder = new GenericDraweeHierarchyBuilder(mContext.getResources());
//设置圆形
RoundingParams circle = RoundingParams.asCircle();
GenericDraweeHierarchy build = genericDraweeHierarchyBuilder.setRoundingParams(circle).build();
viewHolder.rlv_icon.setHierarchy(build);
viewHolder.rlv_icon.setImageURI(parse);
//条目点击事件
viewHolder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mOnClickListener != null){
mOnClickListener.onClick(mResultBeans.get(i).getName());
}
}
});
}
@Override
public int getItemCount() {
return mResultBeans.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
private SimpleDraweeView rlv_icon;
private TextView rlv_title;
public ViewHolder(@NonNull View itemView) {
super(itemView);
rlv_icon = itemView.findViewById(R.id.rlv_icon);
rlv_title = itemView.findViewById(R.id.rlv_title);
}
}
//接口回调 点击事件
public interface OnClickListener{
void onClick(String name);
}
OnClickListener mOnClickListener;
public void setOnClickListener(OnClickListener onClickListener) {
mOnClickListener = onClickListener;
}
}
6.OkHttp二次封装:
package com.example.dsjob;
import android.os.Handler;
import android.os.Looper;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
/**
* date:2018/11/30
* author:李壮(大壮)
* function:OkHttp的二次封装,这里用的是doGet请求
* 相关知识点:单例模式,接口回调 ,handler
*/
public class OkHttpUtils {
private Handler mHandler;
private OkHttpClient mOkHttpClient;
private static OkHttpUtils mOkHttpUtils;
private OkHttpUtils(){
mHandler = new Handler(Looper.getMainLooper());
mOkHttpClient = new OkHttpClient.Builder()
.writeTimeout(10,TimeUnit.SECONDS)
.readTimeout(10,TimeUnit.SECONDS)
.connectTimeout(10,TimeUnit.SECONDS)
.build();
}
//使用单例模式
public static OkHttpUtils getInstance(){
if (mOkHttpUtils == null){
synchronized (OkHttpUtils.class){
if (mOkHttpUtils == null){
return mOkHttpUtils = new OkHttpUtils();
}
}
}
return mOkHttpUtils;
}
//接口回调
public interface OkCallback{
//成功
void success(String json);
//失败
void failtrue(Exception e);
}
//封装doGet请求
public void doGet(String url, final OkCallback okCallback){
//创建Request对象
Request request = new Request.Builder()
.get()
.url(url)
.build();
//创建call对象
Call call = mOkHttpClient.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, final IOException e) {
mHandler.post(new Runnable() {
@Override
public void run() {
if (okCallback != null){
okCallback.failtrue(e);
}
}
});
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
if (response != null && response.isSuccessful()){
final String json = response.body().string();
mHandler.post(new Runnable() {
@Override
public void run() {
if (okCallback != null){
okCallback.success(json);
}
}
});
}
}catch (Exception e){
e.printStackTrace();
}
}
});
}
}
7.Bean类:
package com.example.dsjob;
import java.util.List;
public class DataItem {
private String message;
private String status;
private List<ResultBean> result;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public List<ResultBean> getResult() {
return result;
}
public void setResult(List<ResultBean> result) {
this.result = result;
}
public static class ResultBean {
private boolean followMovie;
private int id;
private String imageUrl;
private String name;
private int rank;
private String summary;
public boolean isFollowMovie() {
return followMovie;
}
public void setFollowMovie(boolean followMovie) {
this.followMovie = followMovie;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getImageUrl() {
return imageUrl;
}
public void setImageUrl(String imageUrl) {
this.imageUrl = imageUrl;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getRank() {
return rank;
}
public void setRank(int rank) {
this.rank = rank;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
}
}
8.XML布局文件
扫描二维码关注公众号,回复:
4486976 查看本文章
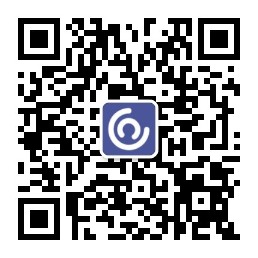
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/rlv"
android:layout_width="match_parent"
android:layout_height="match_parent"></android.support.v7.widget.RecyclerView>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".EventBusActivity">
<TextView
android:id="@+id/tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="接收数值"/>
<Button
android:id="@+id/btn_eventbus"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="返回"/>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.facebook.drawee.view.SimpleDraweeView
android:id="@+id/rlv_icon"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_below="@+id/rlv_title"
android:layout_marginTop="-25dp"
android:src="@mipmap/ic_launcher" />
<TextView
android:id="@+id/rlv_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginStart="127dp"
android:layout_marginLeft="127dp"
android:layout_marginTop="28dp"
android:text="标题" />
</RelativeLayout>
这次就与大家分享这么多,希望能够为大家带来帮助