之前可以先了解一下另一个模块,看本博客的ethereumjs/ethereumjs-common部分内容
通过tests测试文件能够帮助更好了解API的使用
ethereumjs-block/tests/header.js
const tape = require('tape') const Common = require('ethereumjs-common') const utils = require('ethereumjs-util') const rlp = utils.rlp const Header = require('../header.js') const Block = require('../index.js') tape('[Block]: Header functions', function (t) { t.test('should create with default constructor', function (st) { function compareDefaultHeader (st, header) { st.deepEqual(header.parentHash, utils.zeros(32)) st.equal(header.uncleHash.toString('hex'), utils.SHA3_RLP_ARRAY_S) st.deepEqual(header.coinbase, utils.zeros(20)) st.deepEqual(header.stateRoot, utils.zeros(32)) st.equal(header.transactionsTrie.toString('hex'), utils.SHA3_RLP_S) st.equal(header.receiptTrie.toString('hex'), utils.SHA3_RLP_S) st.deepEqual(header.bloom, utils.zeros(256)) st.deepEqual(header.difficulty, Buffer.from([])) st.deepEqual(header.number, utils.intToBuffer(1150000)) st.deepEqual(header.gasLimit, Buffer.from('ffffffffffffff', 'hex')) st.deepEqual(header.gasUsed, Buffer.from([])) st.deepEqual(header.timestamp, Buffer.from([])) st.deepEqual(header.extraData, Buffer.from([])) st.deepEqual(header.mixHash, utils.zeros(32)) st.deepEqual(header.nonce, utils.zeros(8)) } var header = new Header() compareDefaultHeader(st, header) var block = new Block() header = block.header compareDefaultHeader(st, header) st.end() }) t.test('should test header initialization', function (st) { const header1 = new Header(null, { 'chain': 'ropsten' }) //初始化,一种是提供chain/hardfork const common = new Common('ropsten') const header2 = new Header(null, { 'common': common })//另一种则是使用common,common和chain不能同时提供,会报错 header1.setGenesisParams()//就是将该区块头设置为规范初始区块头 header2.setGenesisParams() st.strictEqual(header1.hash().toString('hex'), header2.hash().toString('hex'), 'header hashes match')//因为两个都设置为规范初始区块头,所以相应的值是相同的 st.throws(function () { new Header(null, { 'chain': 'ropsten', 'common': common }) }, /not allowed!$/, 'should throw on initialization with chain and common parameter') // eslint-disable-line st.end() }) t.test('should test validateGasLimit', function (st) {//对gasLimit进行验证 const testData = require('./bcBlockGasLimitTest.json').tests const bcBlockGasLimigTestData = testData.BlockGasLimit2p63m1 Object.keys(bcBlockGasLimigTestData).forEach(key => { var parentBlock = new Block(rlp.decode(bcBlockGasLimigTestData[key].genesisRLP)) //使用genesisRLP初始化block得到的是父区块 var block = new Block(rlp.decode(bcBlockGasLimigTestData[key].blocks[0].rlp)) //使用blocks[0].rlp初始化block得到的是本区块 st.equal(block.header.validateGasLimit(parentBlock), true) }) st.end() }) t.test('should test isGenesis', function (st) { var header = new Header() //查看该区块头是否为初始区块 st.equal(header.isGenesis(), false) header.number = Buffer.from([]) //通过设置header.number为空buffer数组就能得到是初始区块的结果 st.equal(header.isGenesis(), true) st.end() }) const testDataGenesis = require('./genesishashestest.json').test t.test('should test genesis hashes (mainnet default)', function (st) { var header = new Header() header.setGenesisParams() st.strictEqual(header.hash().toString('hex'), testDataGenesis.genesis_hash, 'genesis hash match') st.end() }) t.test('should test genesis parameters (ropsten)', function (st) { var genesisHeader = new Header(null, { 'chain': 'ropsten' }) genesisHeader.setGenesisParams() let ropstenStateRoot = '217b0bbcfb72e2d57e28f33cb361b9983513177755dc3f33ce3e7022ed62b77b' st.strictEqual(genesisHeader.stateRoot.toString('hex'), ropstenStateRoot, 'genesis stateRoot match') st.end() }) })
ethereumjs-block/tests/block.js
const tape = require('tape') const Common = require('ethereumjs-common') const rlp = require('ethereumjs-util').rlp const Block = require('../index.js') tape('[Block]: block functions', function (t) { t.test('should test block initialization', function (st) { const block1 = new Block(null, { 'chain': 'ropsten' }) //初始化,一种是提供chain/hardfork const common = new Common('ropsten') const block2 = new Block(null, { 'common': common })//另一种则是使用common,common和chain不能同时提供,会报错 block1.setGenesisParams()//就是将该区块设置为规范初始区块 block2.setGenesisParams() st.strictEqual(block1.hash().toString('hex'), block2.hash().toString('hex'), 'block hashes match')//因为两个都设置为规范初始区块,所以相应的值是相同的 st.throws(function () { new Block(null, { 'chain': 'ropsten', 'common': common }) }, /not allowed!$/, 'should throw on initialization with chain and common parameter') // eslint-disable-line st.end() }) const testData = require('./testdata.json') function testTransactionValidation (st, block) { st.equal(block.validateTransactions(), true) //验证区块中的交易 block.genTxTrie(function () {//必须要先生成了前缀树后才能调用验证前缀树的操作 st.equal(block.validateTransactionsTrie(), true) st.end() }) } t.test('should test transaction validation', function (st) { var block = new Block(rlp.decode(testData.blocks[0].rlp)) st.plan(2) testTransactionValidation(st, block) }) t.test('should test transaction validation with empty transaction list', function (st) { var block = new Block() st.plan(2) testTransactionValidation(st, block) }) const testData2 = require('./testdata2.json') t.test('should test uncles hash validation', function (st) { var block = new Block(rlp.decode(testData2.blocks[2].rlp))//从区块信息文件生成相同区块 st.equal(block.validateUnclesHash(), true)//验证该区块的叔块hash st.end() }) t.test('should test isGenesis (mainnet default)', function (st) { var block = new Block() st.notEqual(block.isGenesis(), true) //查看是否为初始区块,为false block.header.number = Buffer.from([])//决定因素是block.header.number,设置为空数组buffer即可 st.equal(block.isGenesis(), true) st.end() }) t.test('should test isGenesis (ropsten)', function (st) { var block = new Block(null, { 'chain': 'ropsten' }) st.notEqual(block.isGenesis(), true) block.header.number = Buffer.from([]) st.equal(block.isGenesis(), true) st.end() }) const testDataGenesis = require('./genesishashestest.json').test//(初始区块信息) t.test('should test genesis hashes (mainnet default)', function (st) { var genesisBlock = new Block() genesisBlock.setGenesisParams()//设置为初始区块 var rlp = genesisBlock.serialize() //序列化,就是将其生产rlp格式 st.strictEqual(rlp.toString('hex'), testDataGenesis.genesis_rlp_hex, 'rlp hex match') st.strictEqual(genesisBlock.hash().toString('hex'), testDataGenesis.genesis_hash, 'genesis hash match') st.end() }) t.test('should test genesis hashes (ropsten)', function (st) { var common = new Common('ropsten') var genesisBlock = new Block(null, { common: common }) genesisBlock.setGenesisParams() st.strictEqual(genesisBlock.hash().toString('hex'), common.genesis().hash.slice(2), 'genesis hash match') st.end() }) t.test('should test genesis hashes (rinkeby)', function (st) { var common = new Common('rinkeby') var genesisBlock = new Block(null, { common: common }) genesisBlock.setGenesisParams() st.strictEqual(genesisBlock.hash().toString('hex'), common.genesis().hash.slice(2), 'genesis hash match') st.end() }) t.test('should test genesis parameters (ropsten)', function (st) { var genesisBlock = new Block(null, { 'chain': 'ropsten' }) genesisBlock.setGenesisParams() let ropstenStateRoot = '217b0bbcfb72e2d57e28f33cb361b9983513177755dc3f33ce3e7022ed62b77b' st.strictEqual(genesisBlock.header.stateRoot.toString('hex'), ropstenStateRoot, 'genesis stateRoot match') st.end() }) t.test('should test toJSON', function (st) { var block = new Block(rlp.decode(testData2.blocks[2].rlp)) st.equal(typeof (block.toJSON()), 'object') //返回值为object类型 st.equal(typeof (block.toJSON(true)), 'object') st.end() }) })
ethereumjs-block/tests/difficulty.js
const utils = require('ethereumjs-util') const tape = require('tape') const Block = require('../') const BN = utils.BN function normalize (data) { Object.keys(data).map(function (i) { if (i !== 'homestead' && typeof (data[i]) === 'string') { data[i] = utils.isHexPrefixed(data[i]) ? new BN(utils.toBuffer(data[i])) : new BN(data[i]) } }) } tape('[Header]: difficulty tests', t => { function runDifficultyTests (test, parentBlock, block, msg) { normalize(test) var dif = block.header.canonicalDifficulty(parentBlock)//返回区块的规范困难度 t.equal(dif.toString(), test.currentDifficulty.toString(), `test canonicalDifficulty (${msg})`) //从父区块得到的规范困难度与当前区块的困难度应该是相等的 t.assert(block.header.validateDifficulty(parentBlock), `test validateDifficulty (${msg})`) //查看区块头是否符合规范困难度的区块困难度 } const hardforkTestData = { 'chainstart': require('./difficultyFrontier.json').tests, 'homestead': require('./difficultyHomestead.json').tests, 'byzantium': require('./difficultyByzantium.json').tests, 'constantinople': require('./difficultyConstantinople.json').tests } for (let hardfork in hardforkTestData) { const testData = hardforkTestData[hardfork] for (let testName in testData) { let test = testData[testName] let parentBlock = new Block(null, { 'chain': 'mainnet', 'hardfork': hardfork }) parentBlock.header.timestamp = test.parentTimestamp parentBlock.header.difficulty = test.parentDifficulty parentBlock.header.uncleHash = test.parentUncles let block = new Block(null, { 'chain': 'mainnet', 'hardfork': hardfork }) block.header.timestamp = test.currentTimestamp block.header.difficulty = test.currentDifficulty block.header.number = test.currentBlockNumber runDifficultyTests(test, parentBlock, block, 'fork determination by hardfork param') } } const chainTestData = { 'mainnet': require('./difficultyMainNetwork.json').tests, 'ropsten': require('./difficultyRopstenByzantium.json').tests } for (let chain in chainTestData) { const testData = chainTestData[chain] for (let testName in testData) { let test = testData[testName] let parentBlock = new Block(null, { 'chain': chain }) parentBlock.header.timestamp = test.parentTimestamp parentBlock.header.difficulty = test.parentDifficulty parentBlock.header.uncleHash = test.parentUncles let block = new Block(null, { 'chain': chain }) block.header.timestamp = test.currentTimestamp block.header.difficulty = test.currentDifficulty block.header.number = test.currentBlockNumber runDifficultyTests(test, parentBlock, block, 'fork determination by block number') } } t.end()
扫描二维码关注公众号,回复:
4475133 查看本文章
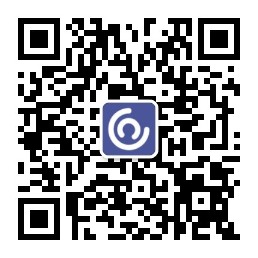