今天来复习一下IO流的api,
在java中用io流来进行文件的输出和输出操作,那么首先类讲解一下什么是输入和输出:
所有往内存中送数据都是输入
所有从内存出数据都是输出
能用java.io包中的api操作的输入输出:
内存–>外存(硬盘,光盘,U盘) 本地流输出
内存<—外存 本地流输入
内存–>网络上 网络流输出
内存<—网络上 网络流输入
一般的网络流的应用场景就是文件的上传的下载
上传文件:先是本地流输入(从硬盘把数据读入到内存)
然后是网络流输出(把内存的数据存储到内存)
远端计算机网络流输入(把网络的数据存储到内存)
远端计算机本地流输出(把内存的数据存储到硬盘)
下载文件:跟上传文件的顺序相反
不能用java.io操作如下:
内存–>显示器
内存–>CPU
内存<–CPU
说到这儿就不得不讲一下数据的持久化的问题了.
数据的持久化:
-数据长时间保留在硬盘上
-数据长时间保存在数据库上,其实数据库的本质就是数据库文件存储在硬盘上
在硬盘中实际体现出来的是文件和目录
File类:专门用来操作目录和文件,但是不能操作文件内容
一批类专门用来操作文件的内容
根据文件内容的操作:
字节流:对文件的读写内容都是用字节方式操作
字符流:对文件的读写内容都是用字符(ascill)的方式操作
File类:用于表示文件和目录,跟内容无关
有关文件和目录间的分隔符的问题:
window方式: C:\aa\bb\cc.txt
Linux 方式: /home/soft/aa/bb/cc.txt
java中对于路径中的分隔符的表示:
window方式: C:\aa\bb\c.txt
Linux方式: /home/soft/aa/bb/cc.txt
如果想兼容:(二者都可以,萝卜青菜各有所爱)
“aa”+File.separator+”bb”+File.separator+”cc.txt”
File的api:
使用一个类之前首先要看它的构造方法
-构建File类对象:File(String filePath);
File(File parent,String child);
File(String parentName,String child);
它的常用api有:
-isFile():用于判断当前的File对象所表示的是否是一个标准的文件
-isDirectory():用于判断当前File对象所表示的是否是一个目录
-length():用于返回文件的长度,如果是目录,window返回结果是0,不会报异常
-exists():用于测试文件或目录是否存在
-createNewFile():用于在当前的目录中创建一个新的空文件,返回结果是Boolean值,如果指定的文件不存在则创建文件返回true;如果指定的文件存在,则返回false
-delete():用于删除指定的目录或文件,若此File对象表示一个目录时,要保证此目录为空才可删除
-mkdir():用于创建目录
-mkdirs():用于创建多层目录
-listFIles():用于返回指定目录中的所有子目录和子文件
-listFIle(FileFilter):用于返回指定目录中的信息,这些信息由FileFilter来过滤
-listFile(FilenameFileFilter):用于返回指定目录中的信息,这些信息由FilenameFileFilter来过滤
-list():用于返回指定目录中的所有信息,信息中不包括子目录和子文件的路径信息
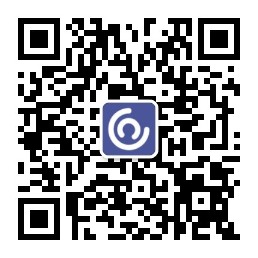
File类中有一个很重要的类,就是RandomAccessFile类:随机读写类
random:随机
access:访问/读写/存储
file:文件
RandomAccessFile类可以对文件做随机读写操作,可以通过seek方法设置指针的位置,然后从指针的位置开始读取字节
seek:搜索/探索/寻找/寻求
RandomAccessFile类文件的随机访问有两种模式:mode
**-只读模式:r
-读写模式:rw**
创建对象:RandomAccessFile(File file,String mode);//创建从中读取和向其中写入的随机访问流,文件指定由File类指定
RandomAccessFile(String name,String mode)//创建从中读取和向其中写入的随机访问流,文件指定由String 类指定
mode的取值: r 只读模式 read
rw 读写模式 read write
-写入操作:
void write(int d);此方法会根据当前指针所在的位置处写入一个字节,是将int的低8位
void write(byte[] d);此方法可以给文件中写入一组字节,根据当前指针的位置连续写出给定数据中的所有字节
byte[] b=”字符串”.getBytes();
void write(byte[] b,int offset,int len);将len个字节从指定byte数组写入文件,并从偏移量offset处开始
-读取操作:
int read();此方法会从文件中读取一个byte字节来填充int的低8位,返回值是0-255;
如果返回-1,则表示读取到文件末尾,每次读取之后自动移动文件指针到下一个字节,准备下次读取
int read(byte[] b);此方法从指针的位置处尝试最多读取给定数组长度的字节量,
并从给定的字节数组的第一个位置开始,将读取到的字节顺序
存放在数组的对应位置,返回的是实际读到的字节数,如果读
到文件末尾,则返回值为-1
int read(byte[] b,int offset,int len);将最多len个数据字节从文件中读入byte数据,并从偏移量offset开始存储
-void getFilePointer();返回此文件中的当前偏移量
-void seek(long position);设置到此文件开头测量到文件指针偏移量,在该位置发生下一个读取或写入的操作
-int skipBytes(int n);此方法可能跳过一些较少量的字节
总结:RandomAccessFile类,此类很特殊,首先是按照字节操作,其次可以对文件中的任意位置可以通过seek方法做文件指针定位
由于File类的方法的使用都比较简单,就不做例子了,下面把RandomAccessFile类的常用api的使用演示一下:
public class TestRandomAccessFileAPIClass {
/**
* 构建RandomAccessFile类对象
* @throws FileNotFoundException
*/
@Test
public void testMethod1() throws FileNotFoundException{
File file1=new File("C:\\Users\\李旭康\\Desktop\\test\\TEST.txt");
File file2=new File("C:\\Users\\李旭康\\Desktop\\test\\TEST.txt");
RandomAccessFile raf1=new RandomAccessFile(file1,"r");
RandomAccessFile raf2=new RandomAccessFile(file2,"rw");
}
/**
* write(int)
* @throws FileNotFoundException
*/
@Test
public void testMethod2() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"rw");
raf.write(55);
raf.close();
}
/**
* int Read()
* @throws Exception
*/
@Test
public void testMethod3() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"r");
int d=raf.read();
System.out.println(d);
}
/**
* write(byte[] b)
* @throws Exception
*/
@Test
public void testMethod4() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"rw");
byte[] b="hello word!".getBytes();
raf.write(b);
raf.close();
}
/**
* int read(byte[] b)
* @throws Exception
*/
@Test
public void testMethod5() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"r");
byte[] b1=new byte[20];
byte[] b2=new byte[16];
int d1=raf.read(b1);//从文件中读取内容放在字节数组中,返回值是读取的字节数
System.out.println(d1);
System.out.println(new String(b1));
/*int d2=raf.read(b2);//从文件中读取内容放在字节数组中,返回值是读取的字节数
System.out.println(d2);
System.out.println(new String(b2));*/
}
/**
* write(byte[] b,int offset,int len)
* @throws Exception
*/
@Test
public void testMethod6() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"rw");
byte[] b="hello word!".getBytes();
raf.write(b,0,6);
raf.close();
}
/**
* int read(byte[] b,int offset,int len)
* @throws Exception
*/
@Test
public void testMethod7() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"r");
byte[] b1=new byte[10];
int d1=raf.read(b1,0,3);
System.out.println(d1);
System.out.println(new String(b1));
}
@Test
public void testMethod8() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"rw");
System.out.println(raf.getFilePointer());
raf.write('a');
System.out.println(raf.getFilePointer());
raf.write(1000);
System.out.println(raf.getFilePointer());
raf.close();
}
/**
* seek(long)
* @throws Exception
*/
@Test
public void testMethod9() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"rw");
raf.seek(2);
System.out.println(raf.getFilePointer());
int k=raf.read();
System.out.println(k);
System.out.println(raf.getFilePointer());
raf.seek(2);//重新移回2
System.out.println(raf.getFilePointer());
raf.seek(raf.length()-3);
System.out.println(raf.getFilePointer());
k=raf.read();
System.out.println("k="+k);
System.out.println(raf.getFilePointer());
raf.close();
}
/**
* int skipBytes(int)
* @throws Exception
*/
@Test
public void testMethod10() throws Exception{
File file=new File("C:\\Users\\李旭康\\Desktop\\test\\test.txt");
RandomAccessFile raf=new RandomAccessFile(file,"rw");
raf.skipBytes(3);
System.out.println(raf.getFilePointer());
int k=raf.read();
System.out.println(k);
System.out.println(raf.getFilePointer());
raf.skipBytes(3);
System.out.println(raf.getFilePointer());
k=raf.read();
System.out.println(k);
System.out.println(raf.getFilePointer());
raf.close();
}
}
FIle的IO的方法还有FileOutputStream类和FileOutputStream类,由于这两个类很重要,本文就先不做讲解,我将会在下篇文章中详细讲解.