1、典型问题一
下面的程序输出什么为什么?
- #include <stdio.h>
- int main()
- {
- char buf[10] = {0};
- char src[] = "hello %s";
- snprintf(buf, sizeof(buf), src);
- printf("buf = %s\n", buf);
- return 0;
- }
分析
snprintf函数本身是可变参数函数,原型如下:
int snprintf(char* buffer, int buf_size, const char*fomart, ...)当函数只有3个参数时,如果第三个参数没有包含格式化信
息,函数调用没有问题;相反,如果第三个参数包含了格式
化信息,但缺少后续对应参数,则程序行为不确定。
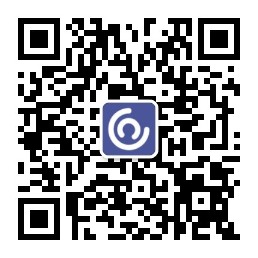
2、典型问题二
下面的程序输出什么为什么?
- #include <stdio.h>
- #include <string.h>
- int main()
- {
- #define STR "Hello, \0D.T.Software\0"
- char* src = STR;
- char buf[255] = {0};
- snprintf(buf, sizeof(buf), src);
- printf("strlen(STR) = %d\n", strlen(STR));
- printf("sizeof(STR) = %d\n", sizeof(STR));
- printf("strlen(src) = %d\n", strlen(src));
- printf("sizeof(src) = %d\n", sizeof(src));
- printf("strlen(buf) = %d\n", strlen(buf));
- printf("sizeof(buf) = %d\n", sizeof(buf));
- printf("src = %s\n", src);
- printf("buf = %s\n", buf);
- return 0;
- }
分析
字符串相关的函数均以第—个出现的 '\0'作为结束符
编译器总是会在字符串字面量的未尾添加'\0'
字符串字面量的本质为数组
3、典型问题三
下面的程序输出什么为什么?
- #include <stdio.h>
- #include <string.h>
- int main()
- {
- #define S1 "D.T.Software"
- #define S2 "D.T.Software"
- if( S1 == S2 )
- {
- printf("Equal\n");
- }
- else
- {
- printf("Non Equal\n");
- }
- if( strcmp(S1, S2) == 0 )
- {
- printf("Equal\n");
- }
- else
- {
- printf("Non Equal\n");
- }
- return 0;
- }
分析
字符串之间的相等比较需要用strcmp完成
不可直接用==进行字符串直接的比较
完全相同的字符串字面量的==比较结果为false
一些现代编译器能够将相同的字符串字面溢
映射到同—个无名字符数组,因此==比较
结果为true。
4、典型问题四
字符串循环右移
- #include <stdio.h>
- #include <string.h>
- void right_shift_r(const char* src, char* result, unsigned int n)
- {
- const unsigned int LEN = strlen(src);
- int i = 0;
- for(i=0; i < LEN; i++)
- {
- result[(n + i) % LEN] = src[i];
- }
- result[LEN] = '\0';
- }
- int main()
- {
- char result[255] = {0};
- right_shift_r("abcde", result, 2);
- printf("%s\n", result);
- right_shift_r("abcde", result, 5);
- printf("%s\n", result);
- right_shift_r("abcde", result, 8);
- printf("%s\n", result);
- return 0;
- }