代写OpenGL程序作业、OpenGL 引擎作业代写
构建一个渲染引擎
对于此作业,您需要使用OpenGL编写一个渲染引擎。我们将提供一些框架代码。一些框架代码将在即将到来的实验室进一步解释。它包含在Assignment2OptionB目录中。
骨架代码在线记录。
1.从我们提供的骨架程序开始,编写和测试用于呈现模型文件(.obj文件)的例程,满足以下要求。骨架程序创建窗口和OpenGL上下文,使用Open Asset Import Library1为obj和material(mtl)文件提供基本模型和材料加载的方法,并设置一些有用的数据结构。
2.使用我们的示例文件(Skeleton目录中的person.obj和earthobj.obj)和您选择的文件来测试程序。
3.写一个简短的报告,描述你的程序如何工作以及如何测试。描述您程序中的任何已知缺陷。
4.使用submit342脚本为我们提供一个包含以下内容的目录:
?我们可以使用您提供的CMake文件在CS实验室中在MacOS环境下编译和运行代码。为了避免过大的提交,请确保只提交CMakeList.txt,renderApp.cpp所在的公用目录和应用程序目录,例如骨架目录。最好的选择是创建一个干净的目录,并复制CMakeList.txt,common和你的应用程序目录并提交这个目录。不要提交应用程序和外部库的二进制文件。
?我们将在本课程的艺术展示中使用的示例图像文件以及您用于生成它的模型文件(如果您使用的是不同的模型,或者如果您更改了任何提供的模型)。
?作为pdf文件的简短报告。(这通常是关于一个页面的长度,不要超过三页长。)
您的渲染引擎的要求
我们要求您在提供的框架代码的结构中完成以下任务。
1.实现完整的obj模型加载(我们在实验室中提供assimp库的帮助,框架代码包含单个网格的基本加载功能,没有任何阴影)。主要执行文件:common / Objloader.cpp。
2.实现完整的模型渲染(框架代码提供渲染单个网格的功能)。主要文件执行:common / Group.cpp。
3.确保从mtl文件中加载所有材料(通常mtl文件包含多种材料,材料索引允许在不同网格之间重新使用材料)。从加载的mtl文件中实现正确的材料索引。主要执行文件:common/ Objloader.cpp,common / Group.cpp。
目前只能从mtl文件中读取漫反射参数。请阅读附加参数,以支持漫反射颜色,环境色,镜面颜色和镜面指数(mtl文件中的参数Ka,Kd,Ks和Ns)的材料设置。还要读取漫反射纹理贴图(贴图Kd)的纹理设置。确保处理所有网格的所有材料。主要执行文件:common / Objloader.cpp,common /Group.cpp。
5.将这些附加材料参数传递给着色器(主要文件用于实现:common / MTLShader.cpp和mtlShader.vert和mtlShader.frag在Skeleton中)。
6.使用顶点和片段着色器(Skeleton中的主文件:mtlShader.vert和mtlShader.frag)实现Phong阴影和Blinn-Phong反射模型。
7.实现对透明材料的支持(使用mtl文件中的透明度设置 - 参数d)。你的结果应该如图
1,中。主要执行文件:renderApp.cpp以支持在Skeleton目录中的混合和mtlShader.frag。
8.实现您选择的特殊效果着色器(Toon,Sepia,Black-andWhite(BW)) - 使其可以使用输入键进行选择。 BW的结果可能如图1所示。主要文件执行:renderApp.cpp和mtlShader.frag在Skeleton和common / MTLShader.cpp。
Building a render engine
Forthis assignment you are asked to write a render engine using OpenGL. Wewill provide some skeleton code. Some of the skeleton codewill be furtherexplained in the upcoming labs.It is contained within theAssignment2OptionBdirectory.
The skeleton code is documentedonline.
1. Starting with the skeleton program that we haveprovided, write and testroutines that rendermodel files (.obj files), meeting the requirements below. The skeleton programcreates the window and the OpenGL context,providesmethods for basic model and material loading from obj and material (mtl) filesusing the Open Asset Import Library1, and sets someuseful data structures.
2. Test your program with our sample files (person.objand earthobj.objwithin the Skeleton directory) and withfiles of your choice.
3. Write a short report, describing how your programworks and how youtested it. Describe any knownflaws in your program.
4. Use the submit342 script to provideus with a directory that contains:
? Code that we cancompile and run under the MacOS environmentin the CS labs using the CMake file you provide. In order toavoidtoo large submissions, make sure that youonly submit the CMakeList.txt, the common directory and theapplication directory whererenderApp.cppis located e.g. Skeleton directory. The best optionis to create a clean directory and copy CMakeList.txt, common andyour application directory and submit this one. Don’t submitthebinaries for your application and theexternal libraries.
? A sample imagefile, which we will use in an art display for the course,and the model files you used to generate it (if you are usinga differentmodel or if you changed any of the providedmodels).
? A short report as apdf file. (This should usually be about a page inlength, and not be more than three pages long.)
We will e-mail you to confirm that we have received yourassignment within a week of the due date. Keep a copy of your work at leastuntil the assessment has been returned to you.
Requirements for your render engine
We require that you complete the following tasks within thestructure of the skeleton code provided.
1. Implement complete obj model loading (we provide help with assimp library inthe lab and the skeleton code contains basic loading function for a single meshwithout any shading). Main files for implementation: common/Objloader.cpp.
2. Implement complete model rendering (the skeleton code provides functionalityto render a single mesh). Main files for implementation: common/Group.cpp.
3. Make sure all materials are loaded from the mtl file (Usually a mtl file containsmultiple materials, material indexing allows to reuse materials betweendifferent meshes). Implement correct material indexing from the loaded mtlfile. Main files for implementation: common/Objloader.cpp, common/Group.cpp.
4. At the moment only the diffuse parameter is read from the mtl file. Read additionalparameters to support the material setttings for diffuse color, ambient color,specular color and specular exponent (parameters Ka, Kd, Ks, and Ns from themtl file). Also read the texture settings for the diffuse texture map (mapKd). Make sure that all materials for all meshes are processed. Mainfiles for implementation: common/Objloader.cpp, common/Group.cpp.
5. Pass these additional material parameters to the shaders (main files for implementation:common/MTLShader.cpp and mtlShader.vert and mtlShader.frag in Skeleton).
6. Implement Phong shading and Blinn-Phong reflection model using the vertexand the fragment shader (main files: mtlShader.vert and mtlShader.frag inSkeleton).
7. Implement support for transparent material (use transparency setting fromthe mtl file - parameter d). Your result should look like Figure
1, Middle. The main files for implementation: renderApp.cpp tosupport blending and mtlShader.frag in the Skeleton directory.
8. Implement a Special Effect shader of your choice (Toon, Sepia, Black-andWhite(BW)) - make this an option to select using an input key. The result for BWcould like Figure 1, Right. Main files forimplementation: renderApp.cpp and mtlShader.frag in Skeleton andcommon/MTLShader.cpp.
http://www.daixie0.com/contents/21/1264.htm
本团队核心人员组成主要包括硅谷工程师、BAT一线工程师,国内Top5硕士、博士生,精通德英语!我们主要业务范围是代做编程大作业、课程设计等等。
我们的方向领域:window编程 数值算法 AI人工智能 金融统计 计量分析 大数据 网络编程 WEB编程 通讯编程 游戏编程多媒体linux 外挂编程 程序API图像处理 嵌入式/单片机 数据库编程 控制台 进程与线程 网络安全 汇编语言 硬件编程 软件设计 工程标准规等。其中代写代做编程语言或工具包括但不限于以下范围:
C/C++/C#代写
Java代写
IT代写
Python代写
辅导编程作业
Matlab代写
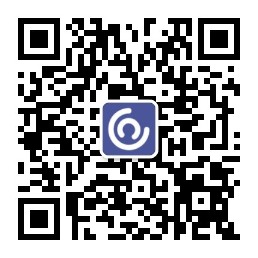
Haskell代写
Processing代写
Linux环境搭建
Rust代写
Data Structure Assginment 数据结构代写
MIPS代写
Machine Learning 作业 代写
Oracle/SQL/PostgreSQL/Pig 数据库代写/代做/辅导
Web开发、网站开发、网站作业
ASP.NET网站开发
Finance Insurace Statistics统计、回归、迭代
Prolog代写
Computer Computational method代做
因为专业,所以值得信赖。如有需要,请加QQ:99515681 或邮箱:[email protected]
微信:codinghelp