python入门学习:9.文件和异常
关键点:文件、异常
9.1 从文件中读取数据9.2 写入文件9.3 异常9.4 存储数据
9.1 从文件中读取数据
9.1.1 读取整个文件
首先创建一个pi_digits.txt文件,内容任意填写,保存在当前目录下。
1with open('pi_digits.txt') as file_object: #在当前目录下查找pi_digits.txt,同时返回一个文件对象
2 contents = file_object.read()
3 print(contents)
4
53.1415926535
6 8979323846
7 2643383279
8
9------------------
第一句代码中关键字with,表示在不需要访问文件后将其自动关闭。通过情况下open()一个文件后,需要close()关闭它。这里使用with,在程序结束后自动关闭。
open('pi_digits.txt')返回一个pi_digits.txt的对象,通过该对象可以操作read函数。
可以看到打印结果有很多空格,因为read到末尾时返回一个空字符串,而将空字符串显示出来就是一个空行。要删除末尾空行,可在print语句中使用rstrip():
1with open('pi_digits.txt') as file_object:
2 contents = file_object.read()
3 print(contents.rstrip())
4
53.1415926535
6 8979323846
7 2643383279
8------------------
9.1.2 文件路径
当调用open()函数时,可以指定文件路径,默认在当前目录下查找。例如当前目录/text_files/filename.txt(相对路径)
1#linux or mac
2with open('text_files/filename.txt') as file_object:
3#windows
4with open('text_files\filename.txt') as file_object:
你还可以将文件在计算机中的准确位置告诉python,即全路径或者绝对文件路径
1#linux or OS
2file_path ='/home/book/Learn/python_work/chapter9/text_files/filename.txt'
3with open(file_path) as file_object:
4
5#windows
6file_path ='C:\Users\ywx\Learn\python_work\chapter9\text_files\filename.txt'
7with open(file_path) as file_object:
9.1.3 逐行读取
读取文件时,常常需要检查其中的每一行,要以每次一行的方式检查文件,可对文件对象使用for循环:
1filename = 'pi_digits.txt'
2with open(filename) as file_object:
3 for line in file_object:
4 print(line.rstrip())
9.1.4 创建一个包含文件各行内容的列表
使用关键字with,open()返回的文件对象只在with代码块内使用。如果要在with代码块外访问文件的内容,可在with代码块内将文件的各行存储在一个列表中,并在with代码块外使用列表:
1filename = 'pi_digits.txt'
2with open(filename) as file_object:
3 lines = file_object.readlines() #读取多行存取到列表lines里
4
5for line in lines:
6 print(line.rstrip())
9.1.5 使用文件的内容
将文件的内容读取后就可以使用文件的内容了。
1filename = 'pi_digits.txt'
2with open(filename) as file_object:
3 lines = file_object.readlines()
4
5pi_string = ''
6for line in lines:
7 pi_string += line.rstrip() #计算圆周率rstrip()删除末尾空格
8
9print(pi_string)
10print(len(pi_string))
11
123.1415926535 8979323846 2643383279
1336
在变量pi_string存储的字符串中,包含原来位于左边的空格。为删除这些空格。可使用strip()而不是rstrip()
1filename = 'pi_digits.txt'
2with open(filename) as file_object:
3 lines = file_object.readlines()
4
5pi_string = ''
6for line in lines:
7 pi_string += line.strip() #删除左边空格
8
9print(pi_string)
10print(len(pi_string))
11
123.141592653589793238462643383279
1332
注意,读取文本文件时,python将其中的所有文本都解读为字符串。如果读取的是数字,并要将其作为数值使用,必须使用函数int()将其转化为整数,使用函数float转换为浮点数
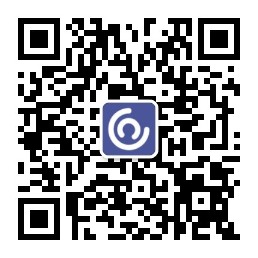
9.2 写入文件
保存数据的最简单方式之一是将其写入到文件中。
9.2.1 写入空文件
要将文本写入文件,在调用open函数时需要提供另一个参数,告诉python你要写入打开的文件。
1filename = 'programming.txt'
2
3with open(filename,'w') as file_object:
4 file_object.write("I love programming")
open第二个参数'w'(可写)。还可以指定'r'(可读)、'a'(附加)或者和可读可写('r+'),如果省略了实参,python默认只读打开。
如果打开的文件不存在,open函数将自动创建它。然而在使用'w'(写)模式打开文件时应注意,如果打开的文件存在,python将在返回文件对象前情况文件。write表示写入文件
9.2.2 写入多行
函数write不会在你写入的文件末尾添加换行,因此如果要写入多行,需要指定换行符。
1filename = 'programming.txt'
2
3with open(filename,'w') as file_object:
4 file_object.write("I love programming\n")
5 file_object.write("I love programming1\n")
9.2.3 附加到文件
如果你要给文件添加内容,而不是覆盖原有内容,可以以附加模式打开。你以附加模式打开文件时,python不会在返回文件对象前清空文件,而是写入到文件的行都将添加到文件末尾。
1filename = 'programming.txt'
2
3with open(filename,'a') as file_object:
4 file_object.write("I also love finding meaning in large datasets.\n")
5 file_object.write("I love creating apps can run n a browser.\n")
9.3 异常
python使用被称为异常的特殊对象来管理程序执行期间发生的错误。每当python不知所措发送错误时,它都会创建一个异常对象。
9.3.1 处理ZerodivisionError异常
下面看一个错误,在Traceback中指出错误ZeroDivisionError是一个异常对象,python无法按照你的要求做时就会创建这种对象,表明0除法异常
1print(5/0)
2Traceback (most recent call last):
3 File "chapter9.py", line 51, in <module>
4 print(5/0)
5ZeroDivisionError: division by zero
9.3.2 使用try-except代码块
当你认为可能发生了错误时,可编写一个try-except代码块来处理可能引发的异常。你让python尝试运行一些代码,并告诉它如果这些代码引发了指定的异常该怎么办。
处理ZeroDivisionError异常的try-except代码块类似于下面这样:
try:
print(5/0)
except ZeroDivisionError:
print("You can't divide by zero!")
9.3.3 使else代码块
通常将可能发生的错误代码放在try-except代码块中可提高这个程序抵御错误的能力,依赖于try代码块成功执行的代码都应放到else代码块中:
1print("Give me two numbers ,and I'll divide them.")
2print("Enter 'q' to quit")
3
4while True:
5 first_number = input("\nFrist number:")
6 if frist_number == 'q':
7 break
8 second_number = input("\nSecond number:")
9 if second_number == 'q':
10 break
11try:
12 answer= int(frist_number )/int(second_number )
13except ZeroDivisionError:
14 print("You can't divide by zero!")
15else:
16 print(answer)
9.3.5 处理FileNotFoundError异常
使用文件时,一种常见的问题是找不到文件:你要查找的文件可能在其他地方、文件名可能不正确或者这个文件根本不存在。对于所有这些情形,都可以使用try-except代码块以直观的方式进行处理。
1filename = 'alice.txt'
2with open(filename) as f_obj:
3 contens = f_obj.read()
4
5Traceback (most recent call last):
6 File "chapter9.py", line 58, in <module>
7 with open(filename) as f_obj:
8FileNotFoundError: [Errno 2] No such file or directory: 'alice.txt'
最后一行报告了FileNotFoundError错误,表示python找不到指定文件,这个错误是调用open函数出现的,所以可以把open函数放在try代码块中:
1filename = 'alice.txt'
2try:
3 with open(filename) as f_obj:
4 contens = f_obj.read()
5except FileNotFoundError:
6 msg = "Sorry,the file "+ filename + " does not exist"
9.3.6 分析文本
split()将字符串以空格符分割,并将结果存储到列表上。下面使用split分割字符串。
1filename = 'alice.txt'
2try:
3 with open(filename) as f_obj:
4 contents = f_obj.read()
5except FileNotFoundError:
6 msg = "Sorry,the file "+ filename + " does not exist"
7 print(msg)
8else:
9 words = contents.split()
10 num_words = len(words)
11 print("The file " + filename + " has about " + str(num_words) + " words")
12
13The file alice.txt has about 29461 words
9.4 存储数据
** 使用json.dump()和json.load()**
模块json让你能够将简单的python数据结构转储到文件中,并在程序再次运行时加载文件中的数据。
- 使用json.dump存储数据
json.dump()接受两个参数,要存储的数据以及可用于存储数据的文件对象。
1import json #导入json模块
2numbers = [2,3,5,7,11,13]
3filename = 'numbers,json' #指定文件为.json格式
4with open(filename,'w') as f_obj:
5 json.dump(numbers,f_obj) #将数据列表存储到文件中
- 使用json.load加载数据
1import json
2filename = 'numbers,json'
3with open(filename) as f_obj:
4 numbers = json.load(f_obj) #从json文件中读取列表
5print(numbers)
6
7[2, 3, 5, 7, 11, 13]