目前大大小小的项目中,都不可避免两个系统之间的通讯交互,此处简单整理一下关于HttpClient常用的HttpGet和HttpPost这两个类的编码方式。
HttpClient常用的HttpGet和HttpPost这两个类分别对应Get方式和Post方式。
无论是使用HttpGet,还是使用HttpPost,都必须通过如下3步来访问HTTP资源。
1.创建HttpGet或HttpPost对象,将要请求的URL通过构造方法传入HttpGet或HttpPost对象。
2.使用DefaultHttpClient类的execute方法发送HTTP GET或HTTP POST请求,并返回HttpResponse对象。
3.通过HttpResponse接口的getEntity方法返回响应信息,并进行相应的处理。
如果使用HttpPost方法提交HTTP POST请求,则需要使用HttpPost类的setEntity方法设置请求参数。参数则必须用NameValuePair[]数组存储。
首先介绍HttpGet方式请求:
无参数的Get请求
/**
* 封装HTTP GET方法
* 无参数的Get请求
* @param
* @return
* @throws ClientProtocolException
* @throws java.io.IOException
*/
public static String get(String url) throws ClientProtocolException, IOException {
//首先需要先创建一个DefaultHttpClient的实例
HttpClient httpClient = new DefaultHttpClient();
//先创建一个HttpGet对象,传入目标的网络地址,然后调用HttpClient的execute()方法即可:
HttpGet httpGet = new HttpGet();
httpGet.setURI(URI.create(url));
HttpResponse response = httpClient.execute(httpGet);
String httpEntityContent = getHttpEntityContent(response);
httpGet.abort();
return httpEntityContent;
}
有参数的Get请求
/**
* 封装HTTP GET方法
* 有参数的Get请求
* @param
* @param
* @return
* @throws ClientProtocolException
* @throws java.io.IOException
*/
public static String get(String url, Map<String, String> paramMap) throws ClientProtocolException, IOException {
HttpClient httpClient = new DefaultHttpClient();
HttpGet httpGet = new HttpGet();
List<NameValuePair> formparams = setHttpParams(paramMap);
String param = URLEncodedUtils.format(formparams, "UTF-8");
httpGet.setURI(URI.create(url + "?" + param));
HttpResponse response = httpClient.execute(httpGet);
String httpEntityContent = getHttpEntityContent(response);
httpGet.abort();
return httpEntityContent;
}
构造请求参数的公共方法(setHttpParams)
扫描二维码关注公众号,回复:
4388605 查看本文章
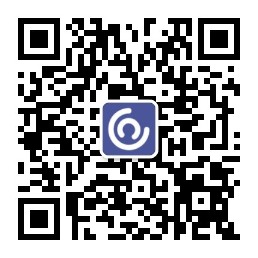
/**
* 设置请求参数
* @param
* @return
*/
private static List<NameValuePair> setHttpParams(Map<String, String> paramMap) {
List<NameValuePair> formparams = new ArrayList<NameValuePair>();
Set<Map.Entry<String, String>> set = paramMap.entrySet();
for (Map.Entry<String, String> entry : set) {
formparams.add(new BasicNameValuePair(entry.getKey(), entry.getValue()));
}
return formparams;
}
获得响应HTTP实体内容(getHttpEntityContent)
/**
* 获得响应HTTP实体内容
* @param response
* @return
* @throws java.io.IOException
* @throws java.io.UnsupportedEncodingException
*/
private static String getHttpEntityContent(HttpResponse response) throws IOException, UnsupportedEncodingException {
//通过HttpResponse 的getEntity()方法获取返回信息
HttpEntity entity = response.getEntity();
if (entity != null) {
InputStream is = entity.getContent();
BufferedReader br = new BufferedReader(new InputStreamReader(is, "UTF-8"));
String line = br.readLine();
StringBuilder sb = new StringBuilder();
while (line != null) {
sb.append(line + "\n");
line = br.readLine();
}
br.close();
is.close();
return sb.toString();
}
return "";
}
POST 请求
/**
* 封装支付HTTP POST方法
* @param
* @param
* @return
* @throws ClientProtocolException
* @throws java.io.IOException
*/
public static String postPayMsg(String url, Map<String, String> paramMap) throws ClientProtocolException, IOException {
HttpClient httpClient = new DefaultHttpClient();
httpClient.getParams().setIntParameter(CoreConnectionPNames.SO_TIMEOUT, 5000);
httpClient.getParams().setIntParameter(CoreConnectionPNames.CONNECTION_TIMEOUT, 3000);
HttpPost httpPost = new HttpPost(url);
List<NameValuePair> formparams = setHttpParams(paramMap);
UrlEncodedFormEntity param = new UrlEncodedFormEntity(formparams, "UTF-8");
//通过setEntity()设置参数给post
httpPost.setEntity(param);
//利用httpClient的execute()方法发送请求并且获取返回参数
HttpResponse response = httpClient.execute(httpPost);
String httpEntityContent = getHttpEntityContent(response);
httpPost.abort();
return httpEntityContent;
}
原博客地址:https://blog.csdn.net/William_Wei007/article/details/75330686?utm_source=copy