IO编程-记事本开发
fis.read(bytes)
字节数组:
通过fis把文件里面的1800个字符,尽可能的读,
最多读1024个。记录一下,0-1024记录到bytes;
再去读的首,fis.read试图1024个字节,不够了,
第二次读了776个,n=776;(0,776);
第三次,又准备读1024,这次一个读不到,返回-1;
退出循环;
JMenuBar

菜单条是一个JMenuBar;
JMenu

File是一个JMenu;
JMenuItem
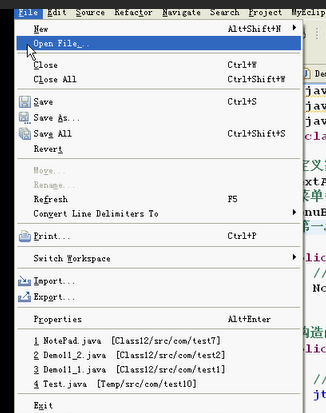
下面这些选项是JMenuItem;
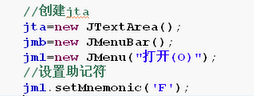
一层放一层的:
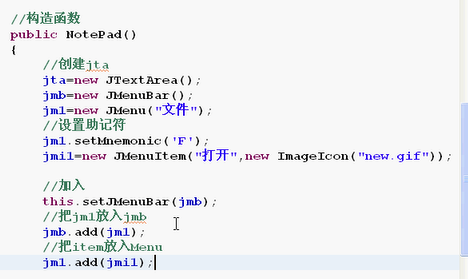
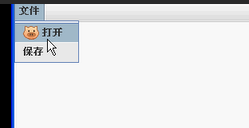
//点击打开,ActionListener,重写方法
actionPerformed
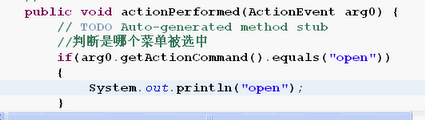
//创建文件选择组件
//swing组件---JFileChooser
setDialogTitle("请选择文件...")
对话框:
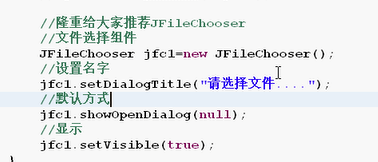
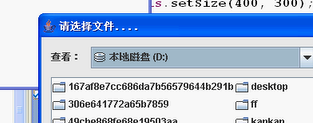
跳出来的组件JAVA设计者已经做好了;
//得到相关文件的路径:
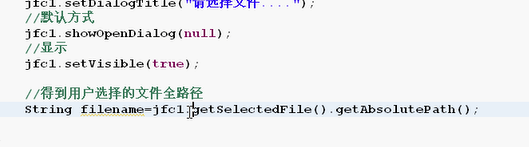
getSelectedFile().getAbsolutePath();
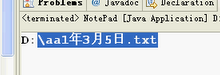
记事本读取:
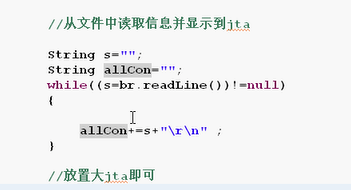
br.readLine( )
//保存-save
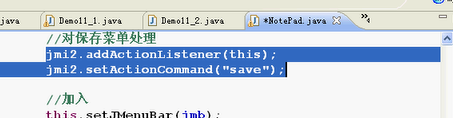
addActionListener(this);
setActionCommand("save");
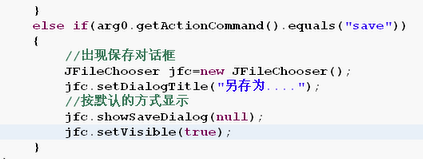
//保存文件到何处:

//准备写入到指定文件即可-write出去:
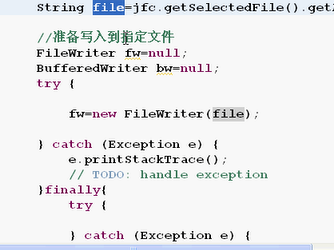
//关闭文件流
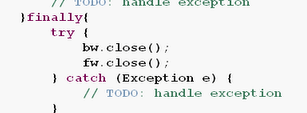
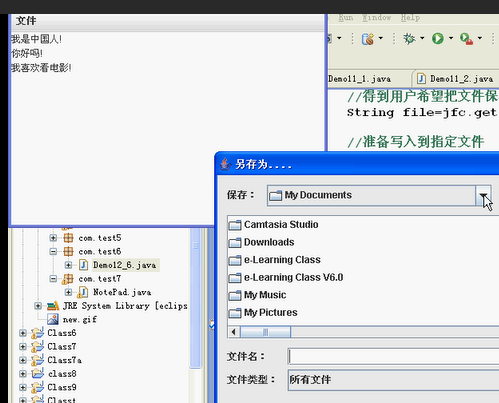
---------------------------------------
java文件编程--常用io流
常用io流--文件字符流
案例[Io05.java](文件字符输入、输出流,目的:FileReader、FileWriter类)读取一个文件并写入到另一个文件中char[]来中转
40
1
/**
2
* File类的基本用法
3
* io流--文件字符流,只能用于完全为字符的文件
4
* TXT文件拷贝--FileReader类与 FileWriter类
5
*/
6
import java.io.*;
7
public class Io05 {
8
public static void main(String[] args) {
9
//文件取出字符流对象(输入流)
10
FileReader fr=null;
11
//写入到文件(输出流)
12
FileWriter fw=null;
13
try {
14
//创建fr对象
15
fr=new FileReader("e:\\ff\\hsp.txt");
16
//创建输出对象
17
fw=new FileWriter("e:\\hsp.txt");
18
//创建字符数组
19
char c[]=new char[1024];
20
int n=0;
21
//读入到内存
22
while((n=fr.read(c))!=-1){
23
//控制台输出TXT文件内容
24
String s=new String(c,0,n);
25
System.out.println(s);
26
fw.write(c, 0, n);
27
}
28
} catch (Exception e) {
29
e.printStackTrace();
30
}finally{
31
try {
32
fr.close();
33
fw.close();
34
} catch (Exception e) {
35
e.printStackTrace();
36
}
37
}
38
}
39
}
40
----------------------------------------------
常用io流--缓冲字符流
为了提高效率引入了缓冲字符流
案例[Io06.java](文件缓冲字符流,目的:BufferedReader和BufferedWriter类介绍,直接操作String)
1
/**
2
* File类的基本用法
3
* io流--缓冲字符流
4
* BufferedReader类与BufferedWriter类
5
*/
6
import java.io.*;
7
public class Io06 {
8
public static void main(String[] args) {
9
BufferedReader br=null;
10
BufferedWriter bw=null;
11
try {
12
//先创建FileReader对象
13
FileReader fr=new FileReader("e:\\ff\\hsp.txt");
14
br=new BufferedReader(fr);
15
16
//创建FileWriter对象
17
FileWriter fw=new FileWriter("e:\\hsp1.txt");
18
bw=new BufferedWriter(fw);
19
20
//循环读取
21
String s="";
22
while((s=br.readLine())!=null){
23
//输出到磁盘
24
bw.write(s+"\r\n");
25
}
26
} catch (Exception e) {
27
e.printStackTrace();
28
}finally{
29
try {
30
br.close();
31
bw.close();
32
} catch (Exception e) {
33
e.printStackTrace();
34
}
35
}
36
}
37
}
38
-----------------------------------------
常用io流--打印输出流PrintWriter
打印输出流PrintWriter可以使用print/println及writer方法。但不换行。需在文本内容中加入\r\n通配符才可以做到。
PrintWriter的使用方法与FileReader、FileWriter/BufferedReader、BufferedWriter基本相同
记事本实例[Io07.java]
x
1
/**
2
* 我的记事本(界面+功能)
3
*/
4
import java.awt.*;
5
import javax.swing.*;
6
import java.io.*;
7
import java.awt.event.*;
8
public class Io07 extends JFrame implements ActionListener{
9
//定义组件
10
JTextArea jta=null;//文本框
11
//菜单条
12
JMenuBar jmb=null;
13
//定义第一个JMenu
14
JMenu jm1=null;
15
//定义JMenuItem
16
JMenuItem jmi1=null;
17
JMenuItem jmi2=null;
18
JMenuItem jmi3=null;
19
20
public static void main(String[] args) {
21
Io07 io=new Io07();
22
}
23
//构造函数
24
public Io07(){
25
//创建组件
26
jta=new JTextArea();
27
jmb=new JMenuBar();
28
jm1=new JMenu("文件(F)");
29
//设置助记符
30
jm1.setMnemonic('F');
31
jmi1=new JMenuItem("打开(O)");
32
//open打开注册监听
33
jmi1.addActionListener(this);
34
jmi1.setActionCommand("open");
35
36
jmi2=new JMenuItem("保存(S)");
37
//save保存注册监听
38
jmi2.addActionListener(this);
39
jmi2.setActionCommand("save");
40
41
jmi3=new JMenuItem("退出(X)");
42
//exit退出注册监听
43
jmi3.addActionListener(this);
44
jmi3.setActionCommand("exit");
45
46
//加入到菜单
47
this.setJMenuBar(jmb);
48
//把jm1放到jmb
49
jmb.add(jm1);
50
//把 jmi1放入jm1
51
jm1.add(jmi1);
52
jm1.add(jmi2);
53
jm1.add(jmi3);
54
55
//设置界面管理器(默认BorderLayout边界布局管理器)
56
57
//加入组件
58
this.add(jta);
59
60
//设置JFrame面板
61
this.setTitle("记事本界面与功能");
62
this.setSize(500, 400);
63
this.setLocationRelativeTo(null);
64
this.setVisible(true);
65
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
66
}
67
68
public void actionPerformed(ActionEvent e) {
69
//判断"打开"菜单被选中
70
if(e.getActionCommand().equals("open")){
71
/**
72
* 隆重推荐JFileChooser组件
73
*/
74
//创建一个文件选择组件
75
JFileChooser jfc=new JFileChooser();
76
//设置名字
77
jfc.setDialogTitle("请选择要打开的文件...");
78
//使用默认方式
79
jfc.showOpenDialog(null);
80
//显示
81
jfc.setVisible(true);
82
83
//得到用户选择的文件全(绝对)路径
84
String filename=jfc.getSelectedFile().getAbsolutePath();
85
86
FileReader fr=null;
87
BufferedReader br=null;
88
try {
89
fr=new FileReader(filename);
90
br=new BufferedReader(fr);
91
//从文件中读取信息并显示到jta(JTextArea)中
92
String s="";
93
String allCon="";
94
while((s=br.readLine())!=null){
95
allCon+=s+"\r\n";//"\r\n"显示文本时将文件中原有的格式显示到jta中
96
}
97
//放置到jta即可
98
jta.setText(allCon);
99
} catch (Exception e2) {
100
e2.printStackTrace();
101
}finally{
102
try {
103
br.close();
104
fr.close();
105
} catch (Exception e1) {
106
e1.printStackTrace();
107
}
108
}//判断"保存"菜单被选中
109
}else if(e.getActionCommand().equals("save")){
110
//创建保存对话框
111
JFileChooser jfc=new JFileChooser();
112
//设置名字
113
jfc.setDialogTitle("将文件保存到...");
114
//使用默认方式
115
jfc.showSaveDialog(null);
116
//显示
117
jfc.setVisible(true);
118
119
//得到用户希望把文件保存到何处,文件全路径
120
String file=jfc.getSelectedFile().getAbsolutePath();
121
122
//准备写入到指定文件
123
FileWriter fw=null;
124
BufferedWriter bw=null;
125
try {
126
fw=new FileWriter(file);
127
bw=new BufferedWriter(fw);
128
//将JtextArea中的内容输出到指定文件中
129
bw.write(this.jta.getText());
130
} catch (Exception e2) {
131
e2.printStackTrace();
132
}finally{
133
try {
134
bw.close();
135
fw.close();
136
} catch (Exception e1) {
137
e1.printStackTrace();
138
}
139
}
140
141
}else if(e.getActionCommand().equals("exit")){
142
System.exit(0);
143
}
144
}
145
}
146
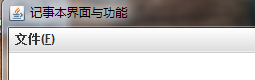
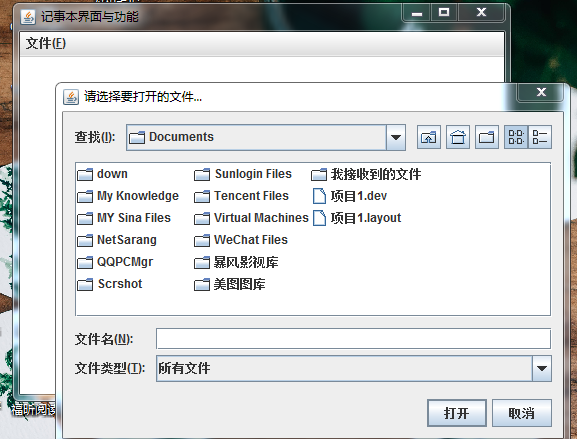
JFileChooser:
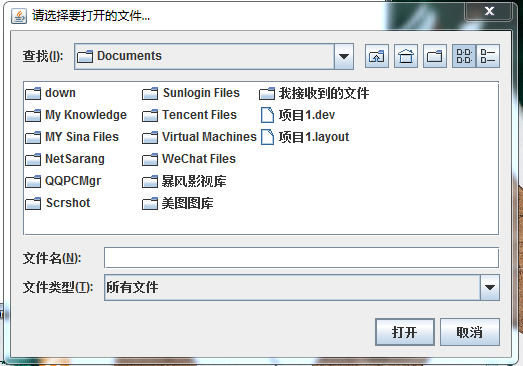
setDialogTitle("请选择要打开的文件")
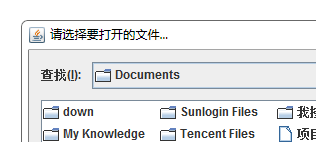
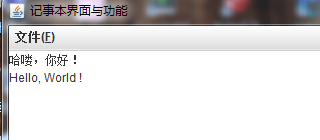
---------------------------------------------
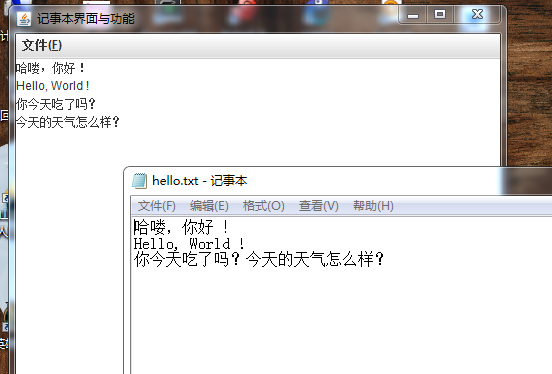