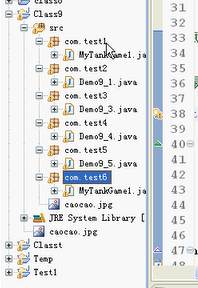
新建一个包:新建一个类,MyTankGame02.java
}
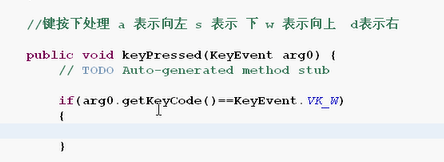
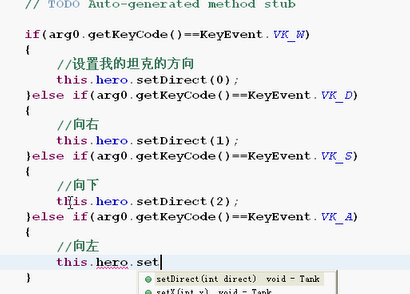
坦克的移动:
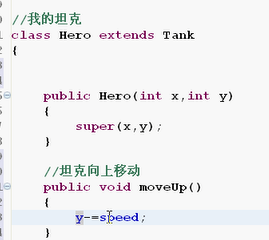
重新绘制repaint才能动起来;
最后,需要注册监听;
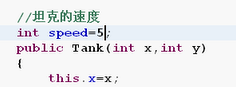
这样,改起来速度很灵活;
改变坦克的方向:
Outline:大纲:
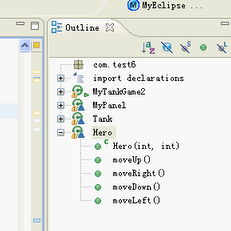
类-方法:
根据通包的访问机制,Members和MyTankGame
是可以相互访问的。
根据方向对函数进行扩展:(坦克炮筒方向)
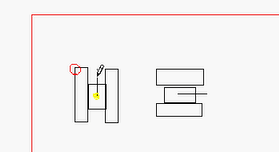
参照点需要从左上角,移动到中心点;
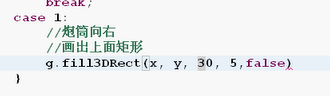
横着放,矩形,宽度、高度需要变化;
敌人的 坦克:
加一个颜色color:
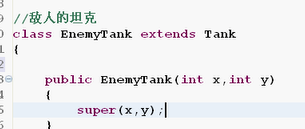
在面板上定义敌人的坦克:
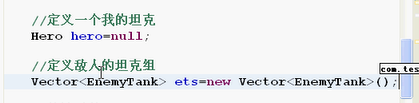
在构造函数里面进行初始化:
每次横坐标间隔50,纵坐标为0:
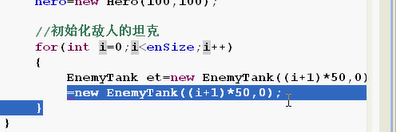
创建一辆敌人坦克对象,加入到集合中;
画出敌人坦克:
用向量算出敌人坦克数量;
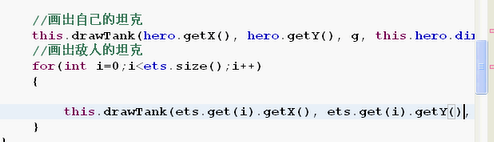
g,direct,type 画笔,方向,坦克颜色
初始化的时候换个方向:
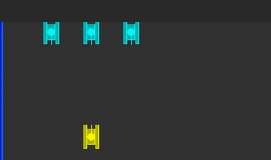
x
1
/**
2
* 功能:坦克游戏的1.01
3
* 1、画出坦克
4
* 2、坦克动起来
5
*/
6
import java.awt.*;
7
import java.awt.event.KeyEvent;
8
import java.awt.event.KeyListener;
9
10
import javax.swing.*;
11
public class MyTank02 extends JFrame{
12
//定义组件
13
MyPanel mp=null;
14
public static void main(String[] args) {
15
MyTank02 mt=new MyTank02();
16
}
17
//构造函数
18
public MyTank02(){
19
//构建组件
20
mp=new MyPanel();
21
22
//监听
23
this.addKeyListener(mp);
24
25
//加入组件
26
this.add(mp);
27
28
//设置JFrame窗体
29
this.setTitle("坦克大战");//JFrame标题
30
this.setSize(400, 300);//JFrame窗体大小
31
this.setLocationRelativeTo(null);//在屏幕中心显示
32
this.setVisible(true);//显示
33
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//退出并关闭JFrame
34
35
}
36
}
37
38
//我的面板Panel
39
class MyPanel extends JPanel implements KeyListener{
40
//定义一个我的坦克
41
Hero hero=null;
42
43
int x=10,y=10,z=0;//初始坦克在MyPanel的位置
44
45
//构造函数
46
public MyPanel(){
47
hero=new Hero(x, y, z);//我的坦克初始位置
48
}
49
50
//重写paint函数
51
public void paint(Graphics g){
52
super.paint(g);//调用父类paint方法
53
//设置Panel底色
54
g.fillRect(0, 0, 400, 300);//fillRect(0,0,X?,Y?)中X?/Y?为活动区域
55
//调用坦克
56
this.drawTank(hero.getX(), hero.getY(), g, 1, hero.getZ());
57
}
58
59
//画出坦克的函数
60
public void drawTank(int x,int y,Graphics g,int type,int direct){
61
//判断是什么类型的坦克
62
switch(type){
63
case 0:
64
g.setColor(Color.cyan);//我的坦克颜色
65
break;
66
case 1:
67
g.setColor(Color.yellow);//敌人坦克颜色
68
break;
69
case 2:
70
g.setColor(Color.red);
71
break;
72
}
73
74
//判断坦克的方向
75
switch(direct){
76
//向上走的坦克
77
case 0:
78
//画出我的坦克(到时再封装成一个函数)
79
//1、画出左边的矩形
80
g.fill3DRect(x, y, 5, 30, false);
81
//2、画出右边的矩形
82
g.fill3DRect(x+15, y, 5, 30, false);
83
//3、画出中间矩形
84
g.fill3DRect(x+5, y+5, 10, 20, false);
85
//4、画出中间圆形
86
g.fillOval(x+5, y+10, 10, 10);
87
//5、画出线(炮筒)
88
g.drawLine(x+10, y+15, x+10, y);
89
break;
90
//向下走的坦克
91
case 1:
92
//1、画出左边的矩形
93
g.fill3DRect(x, y, 5, 30, false);
94
//2、画出右边的矩形
95
g.fill3DRect(x+15, y, 5, 30, false);
96
//3、画出中间矩形
97
g.fill3DRect(x+5, y+5, 10, 20, false);
98
//4、画出中间圆形
99
g.fillOval(x+5, y+10, 10, 10);
100
//5、画出线(炮筒)
101
g.drawLine(x+10, y+15, x+10, y+29);
102
break;
103
//向左走的坦克
104
case 2:
105
//1、画出上边的矩形
106
g.fill3DRect(x, y, 30, 5, false);
107
//2、画出下边的矩形
108
g.fill3DRect(x, y+15, 30, 5, false);
109
//3、画出中间矩形
110
g.fill3DRect(x+5, y+5, 20, 10, false);
111
//4、画出中间圆形
112
g.fillOval(x+10, y+5, 10, 10);
113
//5、画出线(炮筒)
114
g.drawLine(x+15, y+10, x, y+10);
115
break;
116
//向右走的坦克
117
case 3:
118
//1、画出左边的矩形
119
g.fill3DRect(x, y, 30, 5, false);
120
//2、画出右边的矩形
121
g.fill3DRect(x, y+15, 30, 5, false);
122
//3、画出中间矩形
123
g.fill3DRect(x+5, y+5, 20, 10, false);
124
//4、画出中间圆形
125
g.fillOval(x+10, y+5, 10, 10);
126
//5、画出线(炮筒)
127
g.drawLine(x+15, y+10, x+29, y+10);
128
break;
129
}
130
}
131
132
public void keyPressed(KeyEvent e) {//按下键事件
133
if(e.getKeyCode()==KeyEvent.VK_DOWN){
134
hero.setX(x);
135
hero.setY(y++);
136
hero.setZ(z+1);
137
}else if(e.getKeyCode()==KeyEvent.VK_UP){
138
hero.setX(x);
139
hero.setY(y--);
140
hero.setZ(z+0);
141
}else if(e.getKeyCode()==KeyEvent.VK_LEFT){
142
hero.setX(x--);
143
hero.setY(y);
144
hero.setZ(z+2);
145
}else if(e.getKeyCode()==KeyEvent.VK_RIGHT){
146
hero.setX(x++);
147
hero.setY(y);
148
hero.setZ(z+3);
149
}
150
//调用repaint()函数,来重绘界面
151
this.repaint();
152
153
}
154
155
public void keyReleased(KeyEvent e) {//弹起键事件
156
157
158
}
159
160
public void keyTyped(KeyEvent e) {//按键输出值
161
162
163
}
164
165
}
166
167
//定义坦克类
168
class Tank{
169
//表示坦克的X横坐标Y纵坐标Z坦克方向
170
int x=0,y=0,z=0;
171
172
public Tank(int x,int y,int z){
173
this.x=x;
174
this.y=y;
175
this.z=z;
176
}
177
178
public int getX() {
179
return x;
180
}
181
182
public void setX(int x) {
183
this.x = x;
184
}
185
186
public int getY() {
187
return y;
188
}
189
190
public void setY(int y) {
191
this.y = y;
192
}
193
194
public int getZ() {
195
return z;
196
}
197
198
public void setZ(int z) {
199
this.z = z;
200
}
201
}
202
203
//我的坦克
204
class Hero extends Tank{
205
public Hero(int x,int y,int z){
206
super(x,y,z);
207
}
208
}
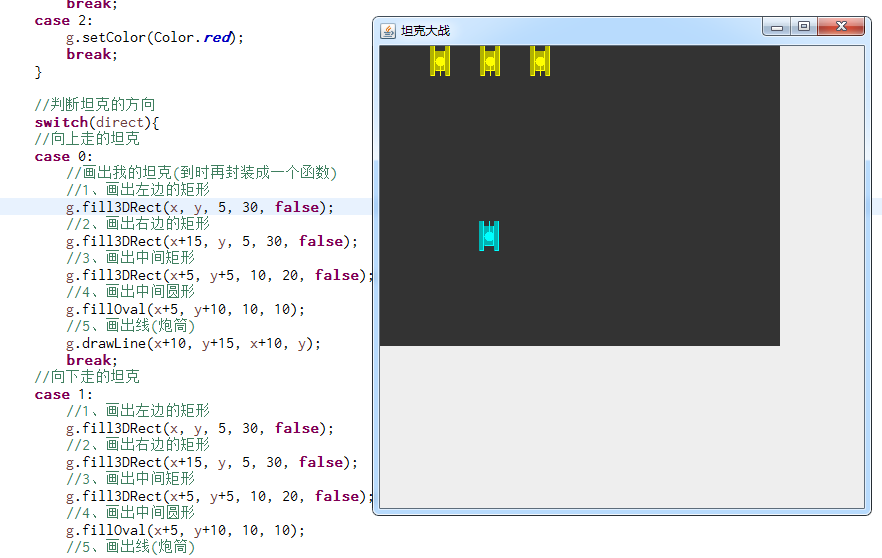