JAVA 布局由于考虑跨平台所以是以相对位置控制的。在JavaFX中,成为Layout Pane(配置面板)
JavaFX提供一个类别,均集成自javafx.scene.layout.Pane
- javafx.scene.layout.AnchorPane
- javafx.scene.layout.BorderPane
- javafx.scene.layout.FlowPane
- javafx.scene.layout.GridPane
- javafx.scene.layout.HBox
- javafx.scene.layout.StackPane
- javafx.scene.layout.TilePane
- javafx.scene.layout.VBox
AnchorPane
AnchorPane的用法和名字Anchor(锚点)的意思很接近。
用下列方法设置AnchorPane各区域与组件之间的距离。
//上方与组件的距离
setTopAnchor()
//上方与组件的距离
setBottomAnchor()
//左方与组件的距离
setLeftAnchor()
//右方与组件的距离
setRightAnchor()
下面以topAnchor,bottomAnchor,leftAnchor,rightAnchor表示方位距离。无论窗体如何变化,组件所定的相对位置不会变化。

image.png
如上图开发程序。
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
/**
* 描述:
* Anchor 美 /'æŋkɚ/
* n. 锚;抛锚停泊;靠山;新闻节目主播
* vt. 抛锚;使固定;主持节目
* vi. 抛锚
* adj. 末棒的;最后一棒的
* @author Felix.Wang
* @create 2018-09-23 14:14
*/
public class AnchorPaneDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
primaryStage.setTitle("AnchorPane Demo");
//定义btn1
Button btn1 = new Button("Button01");
btn1.setPrefSize(100, 30);
//设置间隔
AnchorPane.setTopAnchor(btn1, 50.0);
AnchorPane.setLeftAnchor(btn1, 50.0);
//定义btn2
Button btn2 = new Button("Button02");
btn2.setPrefSize(100, 30);
//设置间隔
AnchorPane.setBottomAnchor(btn2, 50.0);
AnchorPane.setRightAnchor(btn2, 50.0);
//定义AnchorPane,添加node
AnchorPane anchorPane = new AnchorPane();
anchorPane.getChildren().addAll(btn1, btn2);
//定义scene
Scene scene = new Scene(anchorPane);
//设置主界面
primaryStage.setScene(scene);
primaryStage.setWidth(500);
primaryStage.setHeight(500);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}

效果图
BorderPane
BorderPane分为上,下,左,右,中央五个区域,
在JavaFx中用一下方法设置对应位置。
setTop()
setBottom()
setLeft()
setRight()
setCenter()
组件大小不会因为视图变化而变化。
BorderPane有以下几种构造方法:
/**
* Creates a BorderPane layout.
*/
public BorderPane() {
super();
}
/**
* Creates an BorderPane layout with the given Node as the center of the BorderPane.
* @param center The node to set as the center of the BorderPane.
* @since JavaFX 8.0
*/
public BorderPane(Node center) {
super();
setCenter(center);
}
/**
* Creates an BorderPane layout with the given Nodes to use for each of the main
* layout areas of the Border Pane. The top, right, bottom, and left nodes are listed
* in clockwise order.
* @param center The node to set as the center of the BorderPane.
* @param top The node to set as the top of the BorderPane.
* @param right The node to set as the right of the BorderPane.
* @param bottom The node to set as the bottom of the BorderPane.
* @param left The node to set as the left of the BorderPane.
* @since JavaFX 8.0
*/
public BorderPane(Node center, Node top, Node right, Node bottom, Node left) {
super();
setCenter(center);
setTop(top);
setRight(right);
setBottom(bottom);
setLeft(left);
}
BorderPane主要方法如下:
/**
* Returns the child's alignment constraint if set.
* @param child the child node of a border pane
* @return the alignment position for the child or null if no alignment was set
*/
public static Pos getAlignment(Node child) {
return (Pos)getConstraint(child, ALIGNMENT);
}
/**
* Sets the alignment for the child when contained by a border pane.
* If set, will override the border pane's default alignment for the child's position.
* Setting the value to null will remove the constraint.
* @param child the child node of a border pane
* @param value the alignment position for the child
*/
public static void setAlignment(Node child, Pos value) {
setConstraint(child, ALIGNMENT, value);
}
/**
* The node placed on the top edge of this border pane.
* If resizable, it will be resized to its preferred height and it's width
* will span the width of the border pane. If the node cannot be
* resized to fill the top space (it's not resizable or its max size prevents
* it) then it will be aligned top-left within the space unless the child's
* alignment constraint has been set.
*/
public final ObjectProperty<Node> topProperty() {
if (top == null) {
top = new BorderPositionProperty("top");
}
return top;
}
private ObjectProperty<Node> top;
public final void setTop(Node value) { topProperty().set(value); }
public final Node getTop() { return top == null ? null : top.get(); }
/**
* The node placed on the bottom edge of this border pane.
* If resizable, it will be resized to its preferred height and it's width
* will span the width of the border pane. If the node cannot be
* resized to fill the bottom space (it's not resizable or its max size prevents
* it) then it will be aligned bottom-left within the space unless the child's
* alignment constraint has been set.
*/
public final ObjectProperty<Node> bottomProperty() {
if (bottom == null) {
bottom = new BorderPositionProperty("bottom");
}
return bottom;
}
private ObjectProperty<Node> bottom;
public final void setBottom(Node value) { bottomProperty().set(value); }
public final Node getBottom() { return bottom == null ? null : bottom.get(); }
/**
* The node placed on the left edge of this border pane.
* If resizable, it will be resized to its preferred width and it's height
* will span the height of the border pane between the top and bottom nodes.
* If the node cannot be resized to fill the left space (it's not resizable
* or its max size prevents it) then it will be aligned top-left within the space
* unless the child's alignment constraint has been set.
*/
public final ObjectProperty<Node> leftProperty() {
if (left == null) {
left = new BorderPositionProperty("left");
}
return left;
}
private ObjectProperty<Node> left;
public final void setLeft(Node value) { leftProperty().set(value); }
public final Node getLeft() { return left == null ? null : left.get(); }
/**
* The node placed on the right edge of this border pane.
* If resizable, it will be resized to its preferred width and it's height
* will span the height of the border pane between the top and bottom nodes.
* If the node cannot be resized to fill the right space (it's not resizable
* or its max size prevents it) then it will be aligned top-right within the space
* unless the child's alignment constraint has been set.
*/
public final ObjectProperty<Node> rightProperty() {
if (right == null) {
right = new BorderPositionProperty("right");
}
return right;
}
private ObjectProperty<Node> right;
public final void setRight(Node value) { rightProperty().set(value); }
public final Node getRight() { return right == null ? null : right.get(); }
/**
* The node placed in the center of this border pane.
* If resizable, it will be resized fill the center of the border pane
* between the top, bottom, left, and right nodes. If the node cannot be
* resized to fill the center space (it's not resizable or its max size prevents
* it) then it will be center aligned unless the child's alignment constraint
* has been set.
*/
public final ObjectProperty<Node> centerProperty() {
if (center == null) {
center = new BorderPositionProperty("center");
}
return center;
}
private ObjectProperty<Node> center;
public final void setCenter(Node value) { centerProperty().set(value); }
public final Node getCenter() { return center == null ? null : center.get(); }
对齐方式有如下种类:
package javafx.geometry;
import static javafx.geometry.HPos.LEFT;
import static javafx.geometry.HPos.RIGHT;
import static javafx.geometry.VPos.BASELINE;
import static javafx.geometry.VPos.BOTTOM;
import static javafx.geometry.VPos.TOP;
/**
* A set of values for describing vertical and horizontal positioning and
* alignment.
*
* @since JavaFX 2.0
*/
public enum Pos {
/**
* Represents positioning on the top vertically and on the left horizontally.
*/
TOP_LEFT(TOP, LEFT),
/**
* Represents positioning on the top vertically and on the center horizontally.
*/
TOP_CENTER(TOP, HPos.CENTER),
/**
* Represents positioning on the top vertically and on the right horizontally.
*/
TOP_RIGHT(TOP, RIGHT),
/**
* Represents positioning on the center vertically and on the left horizontally.
*/
CENTER_LEFT(VPos.CENTER, LEFT),
/**
* Represents positioning on the center both vertically and horizontally.
*/
CENTER(VPos.CENTER, HPos.CENTER),
/**
* Represents positioning on the center vertically and on the right horizontally.
*/
CENTER_RIGHT(VPos.CENTER, RIGHT),
/**
* Represents positioning on the bottom vertically and on the left horizontally.
*/
BOTTOM_LEFT(BOTTOM, LEFT),
/**
* Represents positioning on the bottom vertically and on the center horizontally.
*/
BOTTOM_CENTER(BOTTOM, HPos.CENTER),
/**
* Represents positioning on the bottom vertically and on the right horizontally.
*/
BOTTOM_RIGHT(BOTTOM, RIGHT),
/**
* Represents positioning on the baseline vertically and on the left horizontally.
*/
BASELINE_LEFT(BASELINE, LEFT),
/**
* Represents positioning on the baseline vertically and on the center horizontally.
*/
BASELINE_CENTER(BASELINE, HPos.CENTER),
/**
* Represents positioning on the baseline vertically and on the right horizontally.
*/
BASELINE_RIGHT(BASELINE, RIGHT);
private final VPos vpos;
private final HPos hpos;
private Pos(VPos vpos, HPos hpos) {
this.vpos = vpos;
this.hpos = hpos;
}
/**
* Returns the vertical positioning/alignment.
* @return the vertical positioning/alignment.
*/
public VPos getVpos() {
return vpos;
}
/**
* Returns the horizontal positioning/alignment.
* @return the horizontal positioning/alignment.
*/
public HPos getHpos() {
return hpos;
}
}

效果图
例子如下:
扫描二维码关注公众号,回复:
4296209 查看本文章
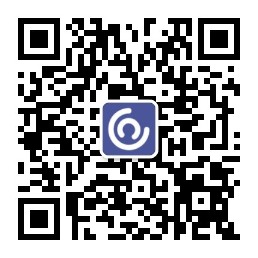
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
/**
* 描述:
*
* @author Felix.Wang
* @create 2018-09-23 14:33
*/
public class BorderPaneDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
//定义按钮
Button topBtn = new Button("TopButton");
topBtn.setPrefSize(100, 30);
Button bottomBtn = new Button("BottomButton");
bottomBtn.setPrefSize(100, 30);
Button leftBtn = new Button("LeftButton");
leftBtn.setPrefSize(100, 30);
Button rightBtn = new Button("RightButton");
rightBtn.setPrefSize(100, 30);
Button centerBtn = new Button("CenterButton");
centerBtn.setPrefSize(100, 30);
BorderPane borderPane = new BorderPane();
//設置區域位置歸屬
borderPane.setTop(topBtn);
borderPane.setBottom(bottomBtn);
borderPane.setLeft(leftBtn);
borderPane.setRight(rightBtn);
borderPane.setCenter(centerBtn);
//设置在每个区域中间位置
BorderPane.setAlignment(topBtn, Pos.CENTER);
BorderPane.setAlignment(bottomBtn, Pos.CENTER);
BorderPane.setAlignment(leftBtn, Pos.CENTER);
BorderPane.setAlignment(rightBtn, Pos.CENTER);
BorderPane.setAlignment(centerBtn, Pos.CENTER);
Scene scene = new Scene(borderPane);
primaryStage.setScene(scene);
primaryStage.setHeight(600);
primaryStage.setWidth(600);
primaryStage.setResizable(true);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
FlowPane
FlowPane有两种方向,默认水平。达到最大宽度或者最大高度会自动换行。
构造方法如下几种:
/**
* Creates a horizontal FlowPane layout with hgap/vgap = 0.
*/
public FlowPane() {
super();
}
/**
* Creates a FlowPane layout with the specified orientation and hgap/vgap = 0.
* @param orientation the direction the tiles should flow & wrap
*/
public FlowPane(Orientation orientation) {
this();
setOrientation(orientation);
}
/**
* Creates a horizontal FlowPane layout with the specified hgap/vgap.
* @param hgap the amount of horizontal space between each tile
* @param vgap the amount of vertical space between each tile
*/
public FlowPane(double hgap, double vgap) {
this();
setHgap(hgap);
setVgap(vgap);
}
/**
* Creates a FlowPane layout with the specified orientation and hgap/vgap.
* @param orientation the direction the tiles should flow & wrap
* @param hgap the amount of horizontal space between each tile
* @param vgap the amount of vertical space between each tile
*/
public FlowPane(Orientation orientation, double hgap, double vgap) {
this();
setOrientation(orientation);
setHgap(hgap);
setVgap(vgap);
}
/**
* Creates a horizontal FlowPane layout with hgap/vgap = 0.
* @param children The initial set of children for this pane.
* @since JavaFX 8.0
*/
public FlowPane(Node... children) {
super();
getChildren().addAll(children);
}
/**
* Creates a FlowPane layout with the specified orientation and hgap/vgap = 0.
* @param orientation the direction the tiles should flow & wrap
* @param children The initial set of children for this pane.
* @since JavaFX 8.0
*/
public FlowPane(Orientation orientation, Node... children) {
this();
setOrientation(orientation);
getChildren().addAll(children);
}
/**
* Creates a horizontal FlowPane layout with the specified hgap/vgap.
* @param hgap the amount of horizontal space between each tile
* @param vgap the amount of vertical space between each tile
* @param children The initial set of children for this pane.
* @since JavaFX 8.0
*/
public FlowPane(double hgap, double vgap, Node... children) {
this();
setHgap(hgap);
setVgap(vgap);
getChildren().addAll(children);
}
/**
* Creates a FlowPane layout with the specified orientation and hgap/vgap.
* @param orientation the direction the tiles should flow & wrap
* @param hgap the amount of horizontal space between each tile
* @param vgap the amount of vertical space between each tile
* @param children The initial set of children for this pane.
* @since JavaFX 8.0
*/
public FlowPane(Orientation orientation, double hgap, double vgap, Node... children) {
this();
setOrientation(orientation);
setHgap(hgap);
setVgap(vgap);
getChildren().addAll(children);
}
参数说明:
package javafx.geometry;
/**
* Orientation
* @since JavaFX 2.0
*/
public enum Orientation {
/**
* The horizontal (right <-> left) orientation
*/
HORIZONTAL,
/**
* The vertical (top <-> bottom) orientation
*/
VERTICAL
}
主要方法:
private ObjectProperty<Pos> alignment;
public final void setAlignment(Pos value) {
alignmentProperty().set(value);
}
public final Pos getAlignment() {
return alignment == null ? Pos.TOP_LEFT : alignment.get();
}
private Pos getAlignmentInternal() {
Pos localPos = getAlignment();
return localPos == null ? Pos.TOP_LEFT : localPos;
}
private DoubleProperty hgap;
public final void setHgap(double value) {
hgapProperty().set(value);
}
public final double getHgap() {
return hgap == null ? 0 : hgap.get();
}
private ObjectProperty<Orientation> orientation;
public final void setOrientation(Orientation value) {
orientationProperty().set(value);
}
public final Orientation getOrientation() {
return orientation == null ? HORIZONTAL : orientation.get();
}
例子:
import javafx.application.Application;
import javafx.geometry.Orientation;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
/**
* 描述:FlowPaneDemo
*
* @author Felix.Wang
* @create 2018-09-23 15:11
*/
public class FlowPaneDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Button btn1 = new Button("btn1");
btn1.setPrefSize(100, 30);
Button btn2 = new Button("btn2");
btn2.setPrefSize(100, 30);
Button btn3 = new Button("btn3");
btn3.setPrefSize(100, 30);
Button btn4 = new Button("btn4");
btn4.setPrefSize(100, 30);
FlowPane flowPane = new FlowPane();
flowPane.getChildren().addAll(btn1, btn2, btn3, btn4);
flowPane.setOrientation(Orientation.VERTICAL);
Scene scene = new Scene(flowPane);
primaryStage.setScene(scene);
primaryStage.setWidth(500);
primaryStage.setHeight(500);
primaryStage.setTitle("LayoutPane");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}

效果图1

效果图2
GridPane
布局方式和html中<table> <tr> <td> </ td> </ tr> </ table>方式比较相似。
</div>
</div>