实验目的与要求
(1) 掌握事件处理的基本原理,理解其用途;
(2) 掌握AWT事件模型的工作机制;
(3) 掌握事件处理的基本编程模型;
(4) 了解GUI界面组件观感设置方法;
(5) 掌握WindowAdapter类、AbstractAction类的用法;
(6) 掌握GUI程序中鼠标事件处理技术。
实验内容和步骤
实验1: 导入第11章示例程序,测试程序并进行代码注释。
测试程序1:
l 在elipse IDE中调试运行教材443页-444页程序11-1,结合程序运行结果理解程序;
l 在事件处理相关代码处添加注释;
l 用lambda表达式简化程序;
l 掌握JButton组件的基本API;
l 掌握Java中事件处理的基本编程模型。
package button;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* 带按钮面板的框架
*/
public class ButtonFrame extends JFrame
{
private JPanel buttonPanel;//定义JPanel属性
private static final int DEFAULT_WIDTH = 600;
private static final int DEFAULT_HEIGHT = 400;
public ButtonFrame()
{
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);//通过setSize更改了宽度和高度的属性值
//创建按钮 生成了三个按钮对象,显示在窗口对象上的title文本
JButton yellowButton = new JButton("Yellow");
JButton blueButton = new JButton("Blue");
JButton redButton = new JButton("Red");
buttonPanel = new JPanel();//使new JPanel指向对象buttonPanel
//向面板添加按钮 通过add方法添加三个按钮组件
buttonPanel.add(yellowButton);
buttonPanel.add(blueButton);
buttonPanel.add(redButton);
// 将面板添加到帧
add(buttonPanel);
// 创建按钮动作
ColorAction yellowAction = new ColorAction(Color.YELLOW);
ColorAction blueAction = new ColorAction(Color.BLUE);
ColorAction redAction = new ColorAction(Color.RED);
//用按钮关联动作 监听器对象与组件之间的注册机制
yellowButton.addActionListener(yellowAction);
blueButton.addActionListener(blueAction);
redButton.addActionListener(redAction);
}
/**
* 设置面板背景颜色的动作侦听器。
*/
private class ColorAction implements ActionListener
//ColorAction类后面实现了一个监听器接口类ActionListener
{
private Color backgroundColor;
public ColorAction(Color c)
{
backgroundColor = c;
}
public void actionPerformed(ActionEvent event)
{
buttonPanel.setBackground(backgroundColor);
}
}
}
package button;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public class ButtonTest
{
public static void main(String[] args)//String类代表字符串。Java 程序中的所有字符串字面值(如 "abc" )都作为此类的实例实现。
{
EventQueue.invokeLater(() -> {
JFrame frame = new ButtonFrame();//生成ButtonFrame对象
frame.setTitle("ButtonTest");//设置组建的自定义标题测试按钮
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//设置默认的关闭操作,参数在关闭动作时退出
frame.setVisible(true);//一个图形界面默认都是不可见的,setVisible把图形界面设置为可见
});
}
}
ButtonTest
package button;
import java.awt.*;
import java.awt.event.*;
import java.nio.charset.CodingErrorAction;
import java.util.Collection;
import javax.swing.*;
/**
* 带按钮面板的框架
*/
@SuppressWarnings("serial")
public class ButtonFrame extends JFrame {
private JPanel buttonPanel;// 定义JPanel属性
private static final int DEFAULT_WIDTH = 600;
private static final int DEFAULT_HEIGHT = 400;
public ButtonFrame() {
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);// 通过setSize更改了宽度和高度的属性值
buttonPanel = new JPanel();// 使new JPanel指向对象buttonPanel
// 将面板添加到帧
add(buttonPanel);
makeButton1("Yellow", Color.yellow);
makeButton1("White", Color.white);
makeButton1("Black", Color.black);
makeButton1("Green", Color.green);
makeButton1("Pink", Color.pink);
makeButton1("Blue", Color.blue);
makeButton1("Gray", Color.gray);
makeButton1("Red", Color.red);
}
public void makeButton1(String name, Color backgroundColor) {
JButton button = new JButton(name);
buttonPanel.add(button);
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
buttonPanel.setBackground(backgroundColor);
}
});
}
}
测试程序2:
l 在elipse IDE中调试运行教材449页程序11-2,结合程序运行结果理解程序;
l 在组件观感设置代码处添加注释;
l 了解GUI程序中观感的设置方法
package
plaf;
import
javax.swing.JButton;
import
javax.swing.JFrame;
import
javax.swing.JPanel;
import
javax.swing.SwingUtilities;
import
javax.swing.UIManager;
/**带有按钮面板的框架,用于改变外观
* A frame with a button panel for changing look-and-feel
*/
public
class
PlafFrame
extends
JFrame
{
private
JPanel buttonPanel;
public
PlafFrame()
{
buttonPanel =
new
JPanel();
//获得一个用于描述已安装的观感的对象数组
UIManager.LookAndFeelInfo[] infos = UIManager.getInstalledLookAndFeels();
for
(UIManager.LookAndFeelInfo info : infos)
//返回观感的显示名称
makeButton(info.getName(), info.getClassName());
//返回观感实现类的名称
add(buttonPanel);
pack();
//调整此窗口的大小,以适合其子组件的首选大小和布局。
}
/**
* Makes a button to change the pluggable look-and-feel.
* @param name the button name
* @param className the name of the look-and-feel class
*/
private
void
makeButton(String name, String className)
{
// 向面板添加按钮
JButton button =
new
JButton(name);
buttonPanel.add(button);
// 设定按钮动作
button.addActionListener(event -> {
// 按钮动作:切换到新的外观
try
{
UIManager.setLookAndFeel(className);
//className - 指定实现外观的类名称的字符串
// Swing方法的集合
SwingUtilities.updateComponentTreeUI(
this
);
//简单的外观更改:将树结构中的每个节点转到 updateUI() -- 也就是说,通过当前外观初始化其 UI 属性。
pack();
}
catch
(Exception e)
//抛出异常
//Exception 类及其子类是 Throwable 的一种形式,它指出了合理的应用程序想要捕获的条件。
{
e.printStackTrace();
//Exception 类及其子类是 Throwable 的一种形式,它指出了合理的应用程序想要捕获的条件。
}
});
}
}
package
plaf;
import
java.awt.*;
import
javax.swing.*;
/**
* @version 1.32 2015-06-12
* @author Cay Horstmann
*/
public
class
PlafTest
{
public
static
void
main(String[] args)
{
//lambda表达式
EventQueue.invokeLater(() -> {
JFrame frame =
new
PlafFrame();
frame.setTitle(
"PlafTest"
);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//退出应用程序默认窗口关闭操作
frame.setVisible(
true
);
});
}
}
package
button;
import
java.awt.*;
import
javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public
class
ButtonTest
{
public
static
void
main(String[] args)
{
//lambda表达式
EventQueue.invokeLater(() -> {
JFrame frame =
new
ButtonFrame();
frame.setTitle(
"ButtonTest"
);
//setTitle表示将此窗体的标题设置为制定的字符串
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//根据参数的值显示或隐藏窗口
frame.setVisible(
true
);
});
}
}
测试程序3:
l 在elipse IDE中调试运行教材457页-458页程序11-3,结合程序运行结果理解程序;
l 掌握AbstractAction类及其动作对象;
l 掌握GUI程序中按钮、键盘动作映射到动作对象的方法。
package action;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/**
* 具有显示颜色变化动作的面板的框架
*/
public class ActionFrame extends JFrame
{
private JPanel buttonPanel;
private static final int DEFAULT_WIDTH = 300;
private static final int DEFAULT_HEIGHT = 200;
public ActionFrame()
{
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
// 设置默认宽度和高度
buttonPanel = new JPanel();
// 将类的实例域中的JPanel面板对象实例化
Action yellowAction = new ColorAction("Yellow", new ImageIcon("yellow-ball.gif"),
Color.YELLOW);
// 创建一个自己定义的ColorAction对象yellowAction
Action blueAction = new ColorAction("Blue", new ImageIcon("blue-ball.gif"), Color.BLUE);
Action redAction = new ColorAction("Red", new ImageIcon("red-ball.gif"), Color.RED);
//创建一个按钮,其属性从所提供的 Action中获取
buttonPanel.add(new JButton(yellowAction));
buttonPanel.add(new JButton(blueAction));
buttonPanel.add(new JButton(redAction));
//我们将这个添加好按钮的面板添加到原框架中
add(buttonPanel);
//我们将JPanel对象的InputMap设置为第二种输入映射,并创建该对象
InputMap imap = buttonPanel.getInputMap(JComponent.WHEN_ANCESTOR_OF_FOCUSED_COMPONENT);
imap.put(KeyStroke.getKeyStroke("ctrl Y"), "panel.yellow");
imap.put(KeyStroke.getKeyStroke("ctrl B"), "panel.blue");
imap.put(KeyStroke.getKeyStroke("ctrl R"), "panel.red");
// 在imap中通过调用击键类KeyStroke的静态方法设置击键输入ctrl+Y的组合
// 第二个参数是一个标志参数,将这对参数用键值对的形式存入imap
// 将imap中标记参数对应的击键组合和相应的Action组合起来
ActionMap amap = buttonPanel.getActionMap();
amap.put("panel.yellow", yellowAction);
amap.put("panel.blue", blueAction);
amap.put("panel.red", redAction);
}
public class ColorAction extends AbstractAction
{
/**
* 构造颜色动作。
* @param name the name to show on the button
* @param icon the icon to display on the button
* @param c the background color
*/
public ColorAction(String name, Icon icon, Color c)
{
putValue(Action.NAME, name);
putValue(Action.SMALL_ICON, icon);
putValue(Action.SHORT_DESCRIPTION, "Set panel color to " + name.toLowerCase());
putValue("color", c);
//在构造器中设置一些键值对映射,这些设置的属性将会被JPanel读取
}
/**
* 当按钮点击或击键的时候,会自动的调用actionPerformed方法
*/
public void actionPerformed(ActionEvent event)
{
Color c = (Color) getValue("color");
buttonPanel.setBackground(c);
// 调用setBackground方法,设置背景颜色
}
}
}
package action;
import java.awt.*;
import javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
import plaf.PlafFrame;
public class ActionTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame = new ActionFrame();//生成ActionFrame对象
frame.setTitle("ActionTest");//设置组建的自定义标题测试按钮
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//设置默认的关闭操作,参数在关闭动作时退出
frame.setVisible(true);//一个图形界面默认都是不可见的,setVisible把图形界面设置为可见
});
}
}
ActionTest
测试程序4:
l 在elipse IDE中调试运行教材462页程序11-4、11-5,结合程序运行结果理解程序;
l 掌握GUI程序中鼠标事件处理技术。
package
mouse;
import
java.awt.*;
import
java.awt.event.*;
import
java.awt.geom.*;
import
java.util.*;
import
javax.swing.*;
/**
* A component with mouse operations for adding and removing squares.
*/
public
class
MouseComponent
extends
JComponent
{
private
static
final
int
DEFAULT_WIDTH =
300
;
private
static
final
int
DEFAULT_HEIGHT =
200
;
private
static
final
int
SIDELENGTH =
10
;
private
ArrayList<Rectangle2D> squares;
private
Rectangle2D current;
// 包含鼠标光标的正方形
public
MouseComponent()
{
squares =
new
ArrayList<>();
current =
null
;
addMouseListener(
new
MouseHandler());
addMouseMotionListener(
new
MouseMotionHandler());
}
public
Dimension getPreferredSize() {
return
new
Dimension(DEFAULT_WIDTH, DEFAULT_HEIGHT); }
public
void
paintComponent(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
// 画所有正方形
for
(Rectangle2D r : squares)
g2.draw(r);
}
/**
* Finds the first square containing a point.
* @param p a point
* @return the first square that contains p
*/
public
Rectangle2D find(Point2D p)
{
for
(Rectangle2D r : squares)
{
if
(r.contains(p))
return
r;
}
return
null
;
}
/**
* Adds a square to the collection.
* @param p the center of the square
*/
public
void
add(Point2D p)
//Point2D 类定义表示 (x,y) 坐标空间中位置的点。
{
double
x = p.getX();
//以 double 精度返回此 Point2D 的 X 坐标
double
y = p.getY();
//以 double 精度返回此 Point2D 的 Y 坐标。
current =
new
Rectangle2D.Double(x - SIDELENGTH /
2
, y - SIDELENGTH /
2
, SIDELENGTH,
SIDELENGTH);
//Rectangle2D 类描述通过位置 (x,y) 和尺寸 (w x h) 定义的矩形。
squares.add(current);
repaint();
//重绘此组件。
}
/**
* 从集合中移除正方形
* @param s the square to remove
*/
public
void
remove(Rectangle2D s)
{
if
(s ==
null
)
return
;
if
(s == current) current =
null
;
squares.remove(s);
repaint();
}
private
class
MouseHandler
extends
MouseAdapter
//接收鼠标事件的抽象适配器类
{
//鼠标按键在组件上按下时调用。
public
void
mousePressed(MouseEvent event)
{
// 如果光标不在正方形内添加一个新的正方形
current = find(event.getPoint());
if
(current ==
null
) add(event.getPoint());
}
//鼠标按键在组件上单击(按下并释放)时调用。
public
void
mouseClicked(MouseEvent event)
{
// 如果双击,删除当前正方形
current = find(event.getPoint());
if
(current !=
null
&& event.getClickCount() >=
2
) remove(current);
}
}
private
class
MouseMotionHandler
implements
MouseMotionListener
{
public
void
mouseMoved(MouseEvent event)
{
// 如果光标在内部,请将其设置为十字准线
//一个矩形
if
(find(event.getPoint()) ==
null
) setCursor(Cursor.getDefaultCursor());
else
setCursor(Cursor.getPredefinedCursor(Cursor.CROSSHAIR_CURSOR));
}
public
void
mouseDragged(MouseEvent event)
{
if
(current !=
null
)
{
int
x = event.getX();
int
y = event.getY();
// 拖住当前矩形,使其居中于 (x, y)
current.setFrame(x - SIDELENGTH /
2
, y - SIDELENGTH /
2
, SIDELENGTH, SIDELENGTH);
repaint();
}
}
}
}
package
mouse;
import
javax.swing.*;
//提供一组“轻量级”(全部是 Java 语言)组件,尽量让这些组件在所有平台上的工作方式都相同。
/**
* 包含用于测试鼠标操作的面板的框架。
*/
public
class
MouseFrame
extends
JFrame
//继承
{
public
MouseFrame()
{
add(
new
MouseComponent());
//将指定组件追加到此容器的尾部
pack();
//调整此窗口的大小,以适合其子组件的首选大小和布局
}
}
package
mouse;
import
java.awt.*;
import
javax.swing.*;
/**
* @version 1.34 2015-06-12
* @author Cay Horstmann
*/
public
class
MouseTest
{
public
static
void
main(String[] args)
{
EventQueue.invokeLater(() -> {
JFrame frame =
new
MouseFrame();
frame.setTitle(
"MouseTest"
);
//设件自定义的鼠标按钮组建
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(
true
);
});
}
}
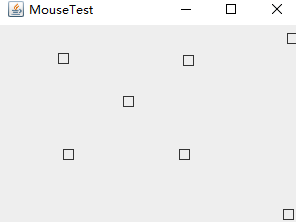
实验2:结对编程练习
利用班级名单文件、文本框和按钮组件,设计一个有如下界面(图1)的点名器,要求用户点击开始按钮后在文本输入框随机显示2017级网络与信息安全班同学姓名,如图2所示,点击停止按钮后,文本输入框不再变换同学姓名,此同学则是被点到的同学姓名。
点名器启动界面
点名器点名界面
代码:
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
public class Fire {
JFrame rFrame=new JFrame("随机点名器");
//名字
String[] stuName={"王之泰","王颖奇","苏浪浪","王斌龙","马兴德","汪慧和","王艳","冯志霞","王志成","张云飞","王海珍","杨野","张燕","唐月晨","李瑞红","李婷华","赵栋","张季跃","孔维滢","穷吉","狄慧","达拉草","杨其菊","马凯军","陈亚茹","常惠琢","马昕璐", "王玉兰", "白玛次仁", "王瑜", "杨蓉庆", "刘志梅", "周强","李清华","李晓菁","徐思","邹丰蔚","罗松","杨玲","王燕","韩腊梅", "东文财", "焦旭超"};
//用于存储名字的标签
JLabel name = new JLabel(); //按钮
JButton btn = new JButton("开始点名"); //采用的是伪随机数,大家也可以不用这个,这个在网上可以找到java随机数的设置 R
Random rd = new Random();
public void init() { //提示标签页面
JLabel jt= new JLabel("随机点名器"); //设置标签居中
jt.setHorizontalAlignment(SwingConstants.CENTER); //设置字体大小
jt.setFont(new java.awt.Font("随机点名器",1,35)); //设置名字显示的标签居中
name.setHorizontalAlignment(SwingConstants.CENTER); //通过匿名类实现Action按钮的监听事件
btn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String n=getRandomName();
//设置name标签的文字
name.setText(n);
//设置字体
name.setFont(new java.awt.Font(n,1,35));
//设置字体颜色
name.setForeground(Color.blue);
}
});
//获取JFrame的面板
Container p = this.rFrame.getContentPane();
//设置布局方式,我采用的BorderLayout布局
p.setLayout(new BorderLayout(3,1));
//添加提示标签在北方
p.add(jt,BorderLayout.NORTH);
//添加姓名标签在中央
p.add(name,BorderLayout.CENTER); //添加按钮控件在南方
p.add(btn,BorderLayout.SOUTH); //调整大小,这个是java中无法设置标签的大小
rFrame.pack(); //设置窗体大小
rFrame.setSize(300, 300);
//设置可以显示
rFrame.setVisible(true);
}
//获取随机的姓名
public String getRandomName() {
int a = 0;
//random类去实现随机数时,只能设置上限,也就是说随机数产生的都是0-stuName.length之间的数字
a = rd.nextInt(stuName.length); //rd.setSeed();
//a = (int)Math.random()*stuName.length;
return stuName[a];
}
public static void main(String[] args) {
Fire rn=new Fire();
rn.init();
}
}
Fire
实验总结:
通过这次实验掌握了事件处理的基本原理、 AWT事件模型的工作机制; 掌握了事件处理的基本编程模型;了解了GUI界面组件观感设置方法;学习了WindowAdapter类、AbstractAction类的用法 以及GUI程序中鼠标事件处理技术。发现自己很多不足之处,还需更加努力。