PHP5中为解决变量的命名冲突和不确定性问题,引入关键字“$this”代表其所在当前对象。
$this在构造函数中指该构造函数所创建的新对象。
在类中使用当前对象的属性和方法,必须使用$this->取值。
方法内的局部变量,不属于对象,不使用$this关键字取值。
局部变量和全局变量与 $this 关键字
使用当前对象的属性必须使用$this关键字。
局部变量的只在当前对象的方法内有效,所以直接使用。
注意:局部变量和属性可以同名,但用法不一样。在使用中,要尽量避免这样使用,以免混淆。
1 <?php 2 class A{ 3 private $a = 99; 4 //这里写一个打印参数的方法. 5 public function printInt($a){ 6 echo "这里的 \$a 是传递的参数 $a "; 7 echo "<br>"; 8 echo "这里的 \$this->a 是属性 $this->a"; 9 } 10 } 11 12 $a = new A(); // 这里的$a 可不是类中的任何一个变量了. 13 $a->printInt(88);
打印结果是
这里的 $a 是传递的参数 88
这里的 $this->a 是属性 99
用$this调用对象中的其它方法
1 <?php 2 //写一个类,让他自动完成最大值的换算 3 class Math{ 4 //两个数值比较大小. 5 public function Max($a,$b){ 6 return $a>$b?$a:$b; 7 } 8 //三个数值比较大小. 9 public function Max3($a,$b,$c){ 10 //调用类中的其它方法. 11 $a = $this->Max($a,$b); 12 return $this->Max($a,$c); 13 } 14 } 15 $math = new Math(); 16 echo "最大值是 ".$math->Max3(99,100,88);
打印结果是:
最大值是 100
扫描二维码关注公众号,回复:
4220872 查看本文章
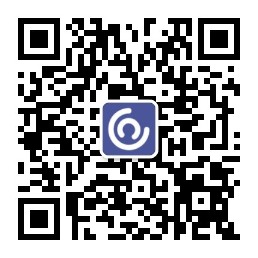
使用$this调用构造函数
调用构造函数和析构函数的方法一致。
1 <?php 2 class A{ 3 private $a = 0; 4 public function __construct(){ 5 $this->a = $this->a + 1 ; 6 } 7 8 public function doSomeThing(){ 9 $this->__construct(); 10 return $this->a; 11 } 12 } 13 $a = new A(); // 这里的$a 可不是类中的任何一个变量了. 14 echo "现在 \$a 的值是" . $a->doSomeThing();
打印结果是:
现在 $a 的值是2
$this 到底指的什么?
$this 就是指当前对象,我们甚至可以返回这个对象使用 $this
1 <?php 2 class A{ 3 public function getASelf(){ 4 return $this; 5 } 6 public function __toString(){ 7 return "这是类A的实例."; 8 } 9 } 10 $a = new A(); // 创建A的实例; 11 $b = $a->getASelf(); //调用方法返回当前实例. 12 echo $a; //打印对象会调用它的__toString方法.
打印结果是:
这是类A的实例.
通过 $this 传递对象
1 <!-- 通过$this 传递对象 2 在这个例子中,我们写一个根据不同的年龄发不同工资的类. 3 我们设置处理年龄和工资的业务模型为一个独立的类. 4 --> 5 <?php 6 class User{ 7 private $age ; 8 private $sal ; 9 private $payoff ; //声明全局属性. 10 11 //构造函数,中创建Payoff的对象. 12 public function __construct(){ 13 $this->payoff = new Payoff(); 14 } 15 public function getAge(){ 16 return $this->age; 17 } 18 public function setAge($age){ 19 $this->age = $age; 20 } 21 // 获得工资. 22 public function getSal(){ 23 $this->sal = $this->payoff->figure($this); 24 return $this->sal; 25 } 26 } 27 //这是对应工资与年龄关系的类. 28 class Payoff{ 29 public function figure($a){ 30 $sal =0; 31 $age = $a->getAge(); 32 if($age >80 || $age <16 ){ 33 $sal = 0; 34 }elseif ($age > 50){ 35 $sal = 1000; 36 }else{ 37 $sal = 800; 38 } 39 return $sal; 40 } 41 } 42 //实例化User 43 $user = new User(); 44 45 $user->setAge(55); 46 echo $user->getAge()."age ,his sal is " . $user->getSal(); 47 echo "<br>"; 48 49 $user->setAge(20); 50 echo $user->getAge()."age , his sal is " . $user->getSal(); 51 echo "<br>"; 52 53 $user->setAge(-20); 54 echo $user->getAge()."age , his sal is " . $user->getSal(); 55 echo "<br>"; 56 57 $user->setAge(150); 58 echo $user->getAge()."age , his sal is " . $user->getSal();
打印结果是:
55age ,his sal is 1000
20age , his sal is 800
-20age , his sal is 0
150age , his sal is 0
转载:http://www.nowamagic.net/php/php_KeywordThis.php