Given a linked list, swap every two adjacent nodes and return its head.
Example:
Given1->2->3->4
, you should return the list as2->1->4->3
Note:
- Your algorithm should use only constant extra space.
- You may not modify the values in the list's nodes, only nodes itself may be changed
给定一个单链表,依次交换每两个节点。
思路一利用三指针pre pcur pnext来依次操作:
每次遍历以两个节点为单位 pre---pcur--pnext
执行操作 交换两个指针
pcur.next = pnext.next
pnext.next = pcur
pre.next = pcur
依次移动指针,进行下一次循环,但是要主要空指针的验证
pre = pcur
扫描二维码关注公众号,回复:
4217935 查看本文章
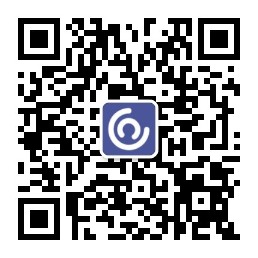
pcur = pcur.next
pnext = pcur.next
class Solution:
def swapPairs(self, head):
"""
:type head: ListNode
:rtype: ListNode
"""
if head==None or head.next==None:
return head
first = head
second = head.next
first.next = second.next
second.next = first
head = second
if first.next==None:
return head
pcur = first.next
if pcur==None or pcur.next==None:
return head
pnext = pcur.next
pre = first
while pcur!= None and pnext!=None:
pcur.next = pnext.next
pnext.next = pcur
pre.next = pnext
pre = pcur
pcur = pcur.next
if pcur!=None and pcur.next!=None:
pnext = pcur.next
return head
思路二利用递归思想来实现:
head--head.next---head.next.next----
假定head.next以后节点已经交换完成且头指针为newhead
head--head.next---newhead
指针交换,完成交换
next = head.next
head.next = newhead
next.next = head
return next
递归的基准条件是head==None 或者head.next==None 此时说明没有节点可以交换,返回即可
class Solution:
def swapPairs(self, head):
"""
:type head: ListNode
:rtype: ListNode
"""
if head!=None and head.next!=None:
newhead = self.swapPairs(head.next.next)
next = head.next
head.next = newhead
next.next = head
return next
else:
return head