比较懒,直接代码展示吧
如下代码展示C++ 的特性。
#include <string>
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <stdint.h>
#include <stddef.h>
#include <string.h>
#include <ctype.h>
#include <fcntl.h>
#include <unistd.h>
#include <signal.h>
#include <pthread.h>
#include <sys/types.h>
/*C++ 覆盖,隐藏, 重载, 拷贝构造, 赋值函数特性例子*/
class Iclass{
public:
Iclass(){}
Iclass(int a, int b){
ad =a;
bc =b;
}
virtual ~Iclass(){}
virtual int getbc(){
printf("Iclass getbc\n");
return bc;
}
int printff(char *msg){
printf("printff%s\n",msg);
return 0;
}
int printff( char *msg, char *msg1){
printf("printff%s %s\n",msg, msg1);
return 0;
}
int hide(int a, int b){
printf("hide%d %d\n",a,b);
return 0;
}
Iclass(Iclass &other){
ad = other.ad;
bc = other.bc;
}
Iclass& operator=(Iclass &other){
if(this == &other){
return *this;
}
ad = other.ad;
bc = other.bc;
return *this;
}
int getad(){
printf("getad :%d\n", ad);
return ad;
}
private:
int ad;
int bc;
};
class IclassEx : public Iclass{
public:
IclassEx(){}
IclassEx(int a, int b, int c):
Iclass(a,b){
cd = c;
}
~IclassEx(){}
virtual int getbc(){
printf("IclassEx getbc\n");
return Iclass::getbc();
}
int hide(int a, int b){
printf("hide%d %d\n",a,b);
printf("hide%d %d\n",a,b);
return 0;
}
IclassEx(IclassEx & other):
Iclass(other){
cd = other.cd;
}
IclassEx& operator=(IclassEx &other){
if(this == &other){
return *this;
}
Iclass::operator=(other);
cd = other.cd;
return *this;
}
private:
int cd;
};
/*C++ 模板函数*/
template <typename T, typename D>
int templatefun(T a, D b){
return 0;
}
/*C++ 模板类*/
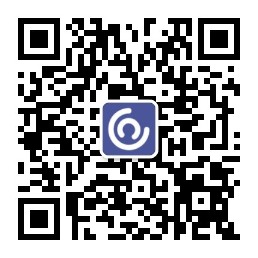
class CDelegateBase {
public:
CDelegateBase(void *pObject, void *pFn) {
m_pObject = pObject;
m_pFn = pFn;
}
CDelegateBase(const CDelegateBase &rhs);
virtual ~CDelegateBase();
bool Equals(const CDelegateBase &rhs) const;
bool operator() (int msg_what, int param1, int param2);
virtual CDelegateBase *Copy() const = 0; // add const for gcc
CDelegateBase &operator=(CDelegateBase ©) {
if (this == ©)return *this;
m_pObject = copy.m_pObject;
m_pFn = copy.m_pFn;
}
protected:
void *GetFn();
void *GetObject();
virtual bool Invoke(int msg_what, int param1, int param2) = 0;
private:
void *m_pObject;
void *m_pFn;
};
template <class O, class T>
class CDelegate : public CDelegateBase {
typedef bool (T::* Fn)(int msg_what, int param1, int param2);
public:
CDelegate(O *pObj, Fn pFn) : CDelegateBase(pObj, &pFn), m_pFn(pFn) { }
CDelegate(const CDelegate &rhs) : CDelegateBase(rhs) {
m_pFn = rhs.m_pFn;
}
virtual CDelegateBase *Copy() const {
return new CDelegate(*this);
}
CDelegate &operator=(CDelegate ©) {
if (this == ©)return *this;
CDelegateBase::operator=(copy);
m_pFn = copy.m_pFn;
}
protected:
virtual bool Invoke(int msg_what, int param1, int param2) {
O *pObject = (O *) GetObject();
return (pObject->*m_pFn)(msg_what, param1, param2);
}
private:
Fn m_pFn;
};
template <class O, class T>
CDelegate<O, T> MakeDelegate(O *pObject, bool (T::* pFn)(int msg_what, int param1, int param2)) {
return CDelegate<O, T>(pObject, pFn);
}
/*C++ 虚拟继承*/
int main(int argc, char** argv)
{
int ret = 0;
Iclass* pthis = NULL;
IclassEx* pthisEx = NULL;
IclassEx a(1,2,3);
pthis = &a;
pthis->getbc(); //覆盖也就是所谓多态
pthis->hide(5,6); //pthis 属于Iclass 类型调用的是基类的hide函数
pthis->printff("hello"); //重载
pthis->printff("hello", "world"); //重载
IclassEx b(a); //拷贝构造
pthis = &b;
pthis->getbc(); //覆盖也就是所谓多态
pthis->hide(5,6); //pthis 属于Iclass 类型调用的是基类的hide函数
pthisEx = &b;
pthisEx->hide(5,6); //隐藏特性, 派生类函数继承了基类的hide函数但是该接口不是virtual的, 派生类直接隐藏基类接口(当做看不见)调用自己的hide函数。
pthis = &b;
pthis->getad();
IclassEx c(7,8,9);
c= b; //赋值操作
pthis = &c;
pthis->getbc();
pthis->hide(5,6);
pthis->getad();
while(1){
sleep(10);
}
return 0;
}
//未完待续, 有时候需要重新看下基础特性知识