总结
1、注释
单行注释:#
多行注释:''' ''' , """ """
2、数据类型
- Number : 数字类型 (int , float , bool , complex)
eg: 1 , 1.0 , True/False , 1+1j
- String: 字符串
eg:'' , 'abc'
- List: 列表
eg:[] , [1,2,3]
- Tuple: 元组
eg:tuple() , (1,2) ,(1,)
- Set: 集合
eg:set() , {1,2,3,'4'}
- Dictionary:字典
eg:{} , {1:2,'a':5}
3、序列(sequence):String , List , Tuple
序列也是有序序列,序列可以使用序列方法,比如:[1::-1]切片操作,通过下标索引访问
list1 = [1,2,3,4]
print(list1[1:2])
print(list1[1])
tuple1 = (1,2,3,4)
print(tuple1[1::2])
print(tuple[2])
str1 = 'abcdef'
print(str1[1:3])
print(str[1:])
#result:
# [2]
# 2
# (2, 4)
# 3
# bc
# bcdef
4、类型转换( int , float , bool , complex , str , list , tuple , dict )
扫描二维码关注公众号,回复:
4199446 查看本文章
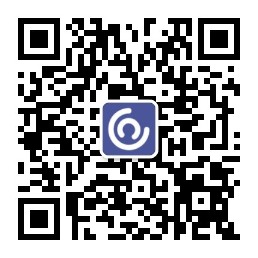
# int , float , bool , complex , str , list , tuple , dict
#-----------类型转换------------------
#all->str
print('==============all->str==================')
print('int-->str:',str(1))
print('complex-->str:',str(1+1j))
print('bool-->str:',str(True))
print('list-->str:',str([1,2,3]))
print('tuple-->str:',str((1,2,3)))
print('set-->str:',str({1,2,3}))
print('dict-->str:',str({1:2,2:3}))
#res:
# int-->str: 1
# complex-->str: (1+1j)
# bool-->str: True
# list-->str: [1, 2, 3]
# tuple-->str: (1, 2, 3)
# set-->str: {1, 2, 3}
# dict-->str: {1: 2, 2: 3}
#all-->list
print('==============all-->list==================')
print('int-->list:转换不了')
print('str-->list:',list('abc'))
print('tuple-->list:',list((1,2,3)))
print('set-->list:',list({1,2,3}))
print('dict-->list:',list({1:2,2:3,'a':'b'})) #只输出key组成的list
# int-->list:转换不了
# str-->list: ['a', 'b', 'c']
# tuple-->list: [1, 2, 3]
# set-->list: [1, 2, 3]
# dict-->list: [1, 2, 'a']
#all-->tuple
print('========all-->tuple:和all-->list一样======')
#all-->set
print('=========all-->set:和all-->list一样========')
#all-->dict
print('===all-->dict:每个元素必须是成对出现的可迭代对象才可转换====')
print('list-->dict:',dict([(1,2),[3,4]]))
print('tuple-->dict:',dict(((1,2),[3,4])))
print(dict(zip([1,2,3],(2,3,5))))
# list-->dict: {1: 2, 3: 4}
# tuple-->dict: {1: 2, 3: 4}
# {1: 2, 2: 3, 3: 5}
#all --> int
print(int(' 5 '))
print(int(' 00500 '))
#error:print(int(' 005 00 ')) #因为方法内部先调用trim(),然后转int,‘5 00’肯定就转不了int
print(int(True))
print(int(False))
# error:print(int(1+2j)) #错误,不能将complex转换为int
# 不能转列表,元组,集合和字典
5、运算符
算数运算符 + , - , * , / , // , % , ** 比较运算符 == , != , > , < , >= , <= 赋值运算符 = , += , -= , *= , /= , %= , **= , //= 位运算符 & , | , ^ , ~ , << , >> 逻辑运算符(返回True/False) and , or , not 成员运算符(返回值为True/False) in , not in
练习:
# ======================算数运算符=========================== # + , - , * , / , // , % , ** print(1 + 2) 3 #序列的拼接,集合和字典没有加法运算符 print([1,2] + ['a',3]) [1, 2, 'a', 3] print('123' + 'abc') 123abc print((1,2,3) + (4,5,6)) (1, 2, 3, 4, 5, 6) #减法运算符 print(1 - 2) -1 print({1,2,3}-{1,5,6}) {2, 3} #集合可以进行-(去掉相同的元素),&(集合的并集) ,|(集合元素的和)运算 #乘法运算符,序列*n print(1 * 5) 5 print('abc'*2) abcabc print((1,2)*2) (1, 2, 1, 2) print([1,2,3]*2) [1, 2, 3, 1, 2, 3] #商值取整数,记住是向下取整 print(1/2) 0.5 print(-1/2) -0.5 print(-1 % 2) 1 print(1 % -2) -1 #取商值 print(5 / 2) 2.5 #取模 print(-5 % 2) 1 #对于负数的值为:除数-被除数*商 #取值的次方 print(5**2) 25 print(16**(1/2)) 4.0 #float类型 # ======================比较运算符=========================== print(1>2) True print(True == 1) True print(False == 0) False print(2 != 2) False print(True > 1j) Error #bool和complex不能进行比较,貌似复数和任何类型都不能进行>,<比较,==可以 print(3>2>2) False print('abc'>'xyz') False print((3,2)>(1,2)) True print(1>'2') Error:报错 TypeError: '>' not supported between instances of 'int' and 'str' print((3,2)>('a','b')) Error:同上 print((3,2)>(2,'b')) True print((3,2)>(3,'b')) Error:同上 #--->所以有没有发现:不同类型之间不能进行比较(Number内部可以进行比较) # ======================赋值运算符=========================== a = 5 a += 1 6 a -= 2 4 a *= 2 8 a /= 2 4 a %= 2 2 a **= 2 4 a //= 2 2 # ======================逻辑运算符=========================== # and , or , not print('=========') print( True and False) False print( 5 > 3 and 3< 5) True print(5>3>5) False #相当于 5>3 and 3>5 >>> 4 and 5 5 >>> 1 or 9 1 >>> not 4 False >>> not 0 True >>> 0 and 4 0 # ======================成员运算符=========================== # in , not in print(1 in [1,2]) True print( 'a' not in (1,2,3)) True
6、
if <条件判断1>:
<执行1>
elif <条件判断2>:
<执行2>
elif <条件判断3>:
<执行3>
else:
<执行4>
age = 20
if age >= 6:
print('teenager')
elif age >= 18:
print('adult')
else:
print('kid')
#这儿条件相当于bool(x)
if x:
print('True')
7、循环语句
Python的循环有两种,一种是for...in循环,依次把list或tuple中的每个元素迭代出来,看例子:
names = ['Michael', 'Bob', 'Tracy']
for name in names:
print(name)
执行这段代码,会依次打印names
的每一个元素:
Michael
Bob
Tracy
Test:
list(range(5))
#res:[0, 1, 2, 3, 4]
另外一种循环就是while循环了
#循环输出1到20的数
count = 0
while count < 20:
count += 1
print(count)
else:
print('ok')