参考资料:
1.雷博博客
2. An ffmpeg and SDL Tutorial
继续FFMPEG学习之路。。。
文章目录
1 综述
本文主要有两份代码,第一份代码就是雷博的《100行实现最简单的FFMPEG视频播放器》,采用的方法就是创建一个线程,定时发出信号,而在主线程中则等待信号进行解码然后显示,整个流程非常清晰,很容易就能看到之间的关系。第二份代码则是参考前面《基于FFMPEG+SDL2播放音频》以及FFMPEG教程《An ffmpeg and SDL Tutorial》将播放线程和解码线程单独分开,每个线程单独做一件事,这样可以更精确的控制播放,但是同时代码复杂程度也同样增大了。。。
2 代码1(基础代码)
此部分代码是基于前面文章《基于FFMPEG将video解码为YUV》以及《基于SDL2播放YUV视频》基础上综合而来,基本思路如下:
初始化复用器和解复用器—>获取输入文件的一些信息—>查找解码器并打开—>初始化SDL—>创建线程定时发出信号—>主线程等待信号解码并显示—>结束,退出。
代码如下:
#include <stdio.h>
#define __STDC_CONSTANT_MACROS
extern "C"
{
#include "libavcodec/avcodec.h"
#include "libavformat/avformat.h"
#include "libswscale/swscale.h"
#include "sdl/SDL.h"
};
//#define debug_msg(fmt, args ...) printf("--->[%s,%d] " fmt "\n\n", __FUNCTION__, __LINE__, ##args)
#define REFRESH_EVENT (SDL_USEREVENT + 1)
#define BREAK_EVENT (SDL_USEREVENT + 2)
int thread_exit=0;
int refresh_video(void *opaque)
{
thread_exit=0;
SDL_Event event;
while (thread_exit==0)
{
event.type = REFRESH_EVENT;
SDL_PushEvent(&event);
SDL_Delay(40);
}
thread_exit=0;
//Break
//SDL_Event event;
event.type = BREAK_EVENT;
SDL_PushEvent(&event);
return 0;
}
int main(int argc, char* argv[])
{
AVFormatContext *pFormatCtx;
AVCodecContext *pCodecCtx;
AVCodec *pCodec;
AVPacket *packet;
AVFrame *pFrame,*pFrameYUV;
int screen_w,screen_h;
SDL_Window *screen;
SDL_Renderer* sdlRenderer;
SDL_Texture* sdlTexture;
SDL_Rect sdlRect;
SDL_Thread *refresh_thread;
SDL_Event event;
struct SwsContext *img_convert_ctx;
uint8_t *out_buffer;
int ret, got_picture;
int i = 0;
int videoindex = -1;
char filepath[]="Titanic.ts";
//char filepath[]="屌丝男士.mov";
av_register_all();
pFormatCtx = avformat_alloc_context();
if(avformat_open_input(&pFormatCtx,filepath,NULL,NULL) != 0)
{
printf("Couldn't open input stream.\n");
return -1;
}
if(avformat_find_stream_info(pFormatCtx,NULL) < 0)
{
printf("Couldn't find stream information.\n");
return -1;
}
for(i = 0; i < pFormatCtx->nb_streams; i++)
{
if(pFormatCtx->streams[i]->codec->codec_type == AVMEDIA_TYPE_VIDEO)
{
videoindex=i;
break;
}
}
if(videoindex == -1)
{
printf("Didn't find a video stream.\n");
return -1;
}
pCodecCtx = pFormatCtx->streams[videoindex]->codec;
pCodec = avcodec_find_decoder(pCodecCtx->codec_id);
if(pCodec == NULL)
{
printf("Codec not found.\n");
return -1;
}
if(avcodec_open2(pCodecCtx, pCodec,NULL)<0)
{
printf("Could not open codec.\n");
return -1;
}
packet=(AVPacket *)av_malloc(sizeof(AVPacket));
pFrame = av_frame_alloc();
pFrameYUV = av_frame_alloc();
out_buffer=(uint8_t *)av_malloc(avpicture_get_size(AV_PIX_FMT_YUV420P, pCodecCtx->width, pCodecCtx->height));
avpicture_fill((AVPicture *)pFrameYUV, out_buffer, AV_PIX_FMT_YUV420P, pCodecCtx->width, pCodecCtx->height);
printf("----------->width is %d, height is %d, size is %d,\n",
pCodecCtx->width, pCodecCtx->height,
avpicture_get_size( pCodecCtx->pix_fmt, pCodecCtx->width, pCodecCtx->height));
//av_dump_format(pFormatCtx,0,filepath,0);
img_convert_ctx = sws_getContext(pCodecCtx->width, pCodecCtx->height, pCodecCtx->pix_fmt,
pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_YUV420P, SWS_BICUBIC, NULL, NULL, NULL);
if(SDL_Init(SDL_INIT_VIDEO | SDL_INIT_TIMER))
{
printf( "Could not initialize SDL - %s\n", SDL_GetError());
return -1;
}
screen_w = pCodecCtx->width;
screen_h = pCodecCtx->height;
screen = SDL_CreateWindow("FFMPEG_SDL2_Play_Video", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED,
screen_w, screen_h,SDL_WINDOW_OPENGL);
if(!screen)
{
printf("SDL: could not create window - exiting:%s\n",SDL_GetError());
return -1;
}
sdlRenderer = SDL_CreateRenderer(screen, -1, 0);
sdlTexture = SDL_CreateTexture(sdlRenderer,SDL_PIXELFORMAT_IYUV, SDL_TEXTUREACCESS_STREAMING,screen_w,screen_h);
refresh_thread = SDL_CreateThread(refresh_video,NULL,NULL);
sdlRect.x = 0;
sdlRect.y = 0;
sdlRect.w = screen_w;
sdlRect.h = screen_h;
while(1)
{
SDL_WaitEvent(&event);
if(event.type==REFRESH_EVENT)
{
if (av_read_frame(pFormatCtx, packet)>=0)
{
if(packet->stream_index==videoindex)
{
ret = avcodec_decode_video2(pCodecCtx, pFrame, &got_picture, packet);
if(ret < 0)
{
printf("Decode Error.\n");
return -1;
}
if(got_picture)
{
sws_scale(img_convert_ctx, (const uint8_t* const*)pFrame->data, pFrame->linesize, 0, pCodecCtx->height,
pFrameYUV->data, pFrameYUV->linesize);
//fwrite(pFrameYUV->data[0],1, (pCodecCtx->width*pCodecCtx->height),fd_yuv);
//fwrite(pFrameYUV->data[1],1, (pCodecCtx->width*pCodecCtx->height)/4,fd_yuv);
//fwrite(pFrameYUV->data[2],1, (pCodecCtx->width*pCodecCtx->height)/4,fd_yuv);
//fwrite(out_buffer,1, (pCodecCtx->width*pCodecCtx->height)*3/2,fd_yuv);
SDL_UpdateTexture( sdlTexture, &sdlRect,out_buffer, pCodecCtx->width);
SDL_RenderClear( sdlRenderer );
SDL_RenderCopy( sdlRenderer, sdlTexture, &sdlRect, &sdlRect );
//SDL_RenderCopy( sdlRenderer, sdlTexture, NULL, NULL);
SDL_RenderPresent( sdlRenderer );
}
}
av_free_packet(packet);
}
else
{
thread_exit=1;
}
}
else if(event.type==SDL_QUIT)
{
thread_exit=1;
}
else if(event.type==BREAK_EVENT)
{
break;
}
}
sws_freeContext(img_convert_ctx);
SDL_Quit();
av_frame_free(&pFrameYUV);
av_frame_free(&pFrame);
avcodec_close(pCodecCtx);
avformat_close_input(&pFormatCtx);
return 0;
}
3 代码2(播放线程和解码线程分开)
将解码线程和播放线程分开,大致思路是:播放主线程通过事件来刷新定时器并且从VideoPicture队列中取出一帧解码后的数据进行显示,在解码线程中从文件中不断读取数据帧并放入AVPacket队列中,然后再创建一个线程(解码线程子线程)从AVPacket队列中不断读出每一帧数据,并将其放入VideoPicture队列中。这样就会形成一个整体循环,一个线程不断的将数据解码并放入队列中,另外一个线程则等待事件来显示并重新刷新事件。
3.1 几个结构体
3.1.1 VideoState
typedef struct _VideoState_
{
AVFormatContext *pFormatCtx;
int videoStreamIndex;
AVStream* pVideoStream;
PacketQueue videoQueue;
VideoPicture pictQueue[VIDEO_PICTURE_QUEUE_SIZE];
int pictSize;
int pictRindex; //取出索引
int pictWindex; //写入索引
SDL_mutex *pictQueueMutex;
SDL_cond *pictQueueCond;
SDL_Thread *videoThrd;
SDL_Thread *parseThrd;
char fileName[128];
int quit;
struct SwsContext *sws_ctx;
}VideoState;
上面的结构体保存了视频的必要信息,videoStreamIndex 为video在文件中的索引,videoQueue队列则是 存放从文件中读取到的AVPacket数据,pictQueue则为存放解码后的YUV数据,pictSize为pictQueue队列中的YUV数据数量,pictRindex为显示视频时候的索引,pictWindex为将YUV数据放到pictQueue队列中的索引,其他参数也是一些必要的信息。
3.1.2 VideoPicture
typedef struct _VideoPicture_
{
AVFrame* pFrame;
int frameWidth;
int frameHeight;
int allocated;
}VideoPicture;
此结构体则为存放解码后的一帧YUV数据,frameWidth和frameHeight则为YUV数据的长和宽,allocated则为一个标志位,用于判断是否需要重新申请AVFrame结构体。
3.2 PacketQueue队列操作
将文件中读取到的每一帧数据,放入到PacketQueue队列中,然后从PacketQueue队列中取出每一帧数据,这一部分相关的操作主要函数有:
void packet_queue_init(PacketQueue *q);
static int packet_queue_put(PacketQueue *q, AVPacket *pkt) ;
static int packet_queue_get(PacketQueue *q, AVPacket *pkt, int block) ;
这一部分的内容在前面文章《基于FFMPEG+SDL2播放音频》中已经做了相关的介绍,这里就不在介绍了。
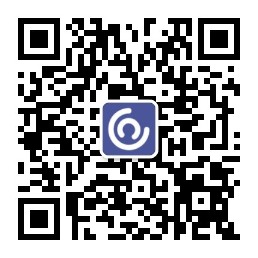
3.3 播放线程
在播放主线程中,我们主要是等待刷新事件进行控制,代码如下:
case FF_REFRESH_EVENT:
if (NULL == pSdlScreen)
{
VideoState* pState = (VideoState *)event.user.data1;
if ((pState->pVideoStream) && (pState->pictSize != 0))
{
sdlWidth = pState->pictQueue[pState->pictRindex].frameWidth;
sdlHeight = pState->pictQueue[pState->pictRindex].frameHeight;
printf("------------>SDL width is %d, height is %d\n", sdlWidth, sdlHeight);
pSdlScreen = SDL_CreateWindow("FFMPEG_SDL2_Play_Video", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED,sdlWidth, sdlHeight,SDL_WINDOW_OPENGL);
if(!pSdlScreen)
{
printf("SDL: could not create window - exiting:%s\n",SDL_GetError());
return -1;
}
pSdlRenderer = SDL_CreateRenderer(pSdlScreen, -1, 0);
pTexTure = SDL_CreateTexture(pSdlRenderer,SDL_PIXELFORMAT_IYUV, SDL_TEXTUREACCESS_STREAMING,sdlWidth,sdlHeight);
}
else
{
schduleRefresh(pState, 1);
break;
}
}
videoRefreshTimer(event.user.data1,pSdlRenderer,pTexTure);
break;
其中videoRefreshTimer函数便是我们的显示函数,在此之前,我们根据YUV数据的长和宽创建了SDL显示相关的窗口、渲染器、纹理。
在介绍videoRefreshTimer之前先介绍一下SDL中的定时器。
3.3.1 SDL中的定时器—SDL_AddTimer
函数原型如下:
SDL_TimerID SDLCALL SDL_AddTimer(Uint32 interval,SDL_TimerCallback callback, void *param);
此函数的作用添加一个新的定时信息,interval单位为ms,添加定时器interval ms后调用回调函数,callback为回调函数,param则为调用回调函数时候携带的参数。
回调函数的原型为:
typedef Uint32 (SDLCALL * SDL_TimerCallback) (Uint32 interval, void *param);
注意,此函数的返回值为下次调用此回调函数的间隔,如果此返回值为0,则不再调用此函数。
代码中的回调相关函数如下:
//定时刷新
void schduleRefresh(VideoState *pState, int delay)
{
SDL_AddTimer(delay, SDLRefreshCB, pState);
}
//定时刷新回调函数
static Uint32 SDLRefreshCB(Uint32 interval, void *opaque)
{
SDL_Event event;
event.type = FF_REFRESH_EVENT;
event.user.data1 = opaque;
SDL_PushEvent(&event);
return 0; /* 0 means stop timer */
}
可以看到,每次调用时候会发出一个刷新事件(FF_REFRESH_EVENT),通知主线程进行解码。同时看到SDLRefreshCB返回值为0,即只调用一次此定时器便失效,然后不再刷新了?不,而是在播放线程中再次添加一个定时器。
3.3.2 播放线程—videoRefreshTimer
先上一下代码:
//刷新定时器并将视频显示到SDL上
void videoRefreshTimer(void *userdata,SDL_Renderer* renderer,SDL_Texture* texture)
{
static int exitCnt = 0;
VideoState* pState = (VideoState *)userdata;
if (pState->pVideoStream)
{
if (pState->pictSize == 0) //当前视频队列中没有视频数据
{
schduleRefresh(pState, 1); // 1ms后再次刷新
if (++exitCnt > 200)
pState->quit = 1;
}
else
{
schduleRefresh(pState, 40);
videoDisplay(pState, renderer, texture); //显示
if(++pState->pictRindex >= VIDEO_PICTURE_QUEUE_SIZE)
{
pState->pictRindex = 0;
}
SDL_LockMutex(pState->pictQueueMutex);
pState->pictSize--;
SDL_CondSignal(pState->pictQueueCond);
SDL_UnlockMutex(pState->pictQueueMutex);
exitCnt = 0;
}
}
else //相关准备还没有做好
{
schduleRefresh(pState, 100);
}
}
播放线程的主要作用便是刷新定时器并且从VideoPicture队列中取出一帧YUV图像进行显示。
在前面我们说过,定时器每次只触发一次,然后在播放线程中再次添加一个定时器,这样做的好处是我们可以精确的控制下次调用播放线程的时间。
播放线程的主要功能便是将一帧YUV图像进行显示。
3.3.2.1 显示画面—videoDisplay
代码如下:
//通过SDL显示画面
void videoDisplay(VideoState *pState,SDL_Renderer* renderer,SDL_Texture* texture)
{
VideoPicture* pVideoPic = &pState->pictQueue[pState->pictRindex];
SDL_Rect sdlRect;
if (pVideoPic->pFrame)
{
sdlRect.x = 0;
sdlRect.y = 0;
sdlRect.w = pVideoPic->frameWidth;
sdlRect.h = pVideoPic->frameHeight;
SDL_UpdateTexture( texture, &sdlRect,pVideoPic->pFrame->data[0], pVideoPic->pFrame->linesize[0]);
SDL_RenderClear( renderer );
//SDL_RenderCopy( sdlRenderer, sdlTexture, &sdlRect, &sdlRect );
SDL_RenderCopy( renderer, texture, &sdlRect , &sdlRect );
SDL_RenderPresent( renderer );
}
}
在上面可以看到,我们直接从队列中取出一帧YUV图像,然后直接通过标准SDL显示流程进行显示。
3.4 解码线程----decordThread
先上代码:
//解码线程
int decordThread(void* arg)
{
VideoState *state = (VideoState *)arg;
AVFormatContext * pFormatCtx = avformat_alloc_context();
AVPacket* packet =(AVPacket *)av_malloc(sizeof(AVPacket));
int i= 0;
int videoIndex = -1;
state->videoStreamIndex = -1;
global_video_state = state;
if(avformat_open_input(&pFormatCtx,state->fileName,NULL,NULL) != 0)
{
printf("Couldn't open input stream.\n");
return -1;
}
state->pFormatCtx = pFormatCtx;
if(avformat_find_stream_info(pFormatCtx,NULL) < 0)
{
printf("Couldn't find stream information.\n");
return -1;
}
for(i = 0; i < pFormatCtx->nb_streams; i++)
{
if(pFormatCtx->streams[i]->codec->codec_type == AVMEDIA_TYPE_VIDEO)
{
videoIndex = i;
break;
}
}
if (videoIndex >= 0)
{
streamComponentOpen(state, videoIndex);
}
if (state->videoStreamIndex < 0)
{
printf("open codec error!\n");
goto fail;
}
while(1)
{
if (state->quit) break;
if (state->videoQueue.size > MAX_VIDEOQ_SIZE) //防止队列过长
{
SDL_Delay(10);
continue;
}
if(av_read_frame(state->pFormatCtx, packet) < 0)
{
if(state->pFormatCtx->pb->error == 0)
{
SDL_Delay(100); /* no error; wait for user input */
continue;
}
else
break;
}
if (packet->stream_index == state->videoStreamIndex)
{
packet_queue_put(&state->videoQueue, packet);
}
else
{
av_free_packet(packet);
}
}
while(state->quit == 0) //等待退出信号
{
SDL_Delay(100);
}
fail:
SDL_Event event;
event.type = FF_QUIT_EVENT;
event.user.data1 = state;
SDL_PushEvent(&event);
return 0;
}
解码线程主要做了以下几个工作:
初始化解码器
从文件中读出每帧数据并放入队列
从队列中取出没帧数据并解码放入VideoPicture队列中
3.4.1 初始化编解码器
初始化编解码器其实包含了FFMPEG一整套的流程,如下:
打开输入文件—>查找文件的一些信息—>查找视频index—>查找解码器—>打开解码器
上面这些流程对应的函数在以前已经说过,这里不再多说。
在查找解码器并打开解码器的时候,另外封装了一个函数streamComponentOpen,这样做减少了代码的复杂性,并且以后音频解码器相关的操作也可以复用此函数。
在初始化解码器后,又创建了一个函数videoThread,此函数的作用便是解码每一帧数据并放入VideoPicture队列中,这一点在下面会说明。
3.4.2 读出每帧数据
从文件中读出每帧数据并将其放入PacketQueue队列中,这部分的操作和前面的文章《基于FFMPEG+SDL2播放音频》中的操作一致,这里不再说明。
另外,由于前面文章《基于FFMPEG+SDL2播放音频》中的音频本身很小,所以会一直读取数据将其放入队列中,但是视频可能很大,几百兆很正常,所以如果由于某种原因导致取数据操作异常或者退出,而将数据放入队列操作缺却一直在进行,这样就会导致内存申请过大,因此在将数据放入队列前,可以做一个判断,当队列中的数据大于某个值的时候,就会暂停放入队列操作,等待队列中的数据被取出,如下:
if (state->videoQueue.size > MAX_VIDEOQ_SIZE) //防止队列过长
{
SDL_Delay(10);
continue;
}
3.4.3 取出每帧数据并解码
从PacketQueue队列中取出每帧数据并解码,这部分的操作和前面的文章《基于FFMPEG+SDL2播放音频》中的操作一致,这里不再说明。
再将解码后的每一帧数据放入VideoPictue队列中去的函数queuePicture中,需要首先判断VideoPictue队列中的AVFrame是否已经初始化,如果没有初始化,则需要发出FF_ALLOC_EVENT事件,等待allocPicture函数初始化好VideoPictue。
最后附上在vs2010上创建好的工程(在vs2010上测试ok):