翻蛮牛看到一篇ugui实现摇杆的文章,我在它的基础上做了扩展,能够像EasyTouch一样派发 begin,moving,end 事件,
并且 在按住摇杆的情况下也能持续调用moving 事件。
参考:http://www.manew.com/thread-98879-1-1.html
JoyStick.cs代码:
using UnityEngine;
using System.Collections;
using System;
using UnityEngine.EventSystems;
public class JoyStickArgs : EventArgs
{
public Vector3 dir;
public JoyStickArgs(Vector3 _dir)
{
dir = _dir;
}
}
public delegate void JoyStickHandler(object o, JoyStickArgs e);
public class JoyStick : MonoBehaviour ,IDragHandler , IBeginDragHandler , IEndDragHandler{
public event JoyStickHandler moveHandler;
public event JoyStickHandler beginHandler;
public event JoyStickHandler endHandler;
private static JoyStick mIns;
public RectTransform viewPivot;
public RectTransform pot;
private float radius;
private Vector3 offset = Vector3.zero;
private bool hasMoving = false;
void Awake()
{
mIns = this;
}
void OnEnable()
{
radius = viewPivot.sizeDelta.x * 0.5f;
hasMoving = false;
offset = Vector3.zero;
}
void OnDisable()
{
// todo:
}
void Update()
{
if(hasMoving)
{
if (moveHandler != null)
moveHandler(this, new JoyStickArgs(offset.normalized));
}
}
// get instance
public static JoyStick Inst()
{
return mIns;
}
// rigister listener
public void RigisterJoyStickHandler(JoyStickHandler _onBeginHandler = null, JoyStickHandler _onMoveHandler = null, JoyStickHandler _onEndHandler = null)
{
if (_onBeginHandler != null)
beginHandler += _onBeginHandler;
if (_onMoveHandler != null)
moveHandler += _onMoveHandler;
if (_onEndHandler != null)
endHandler += _onEndHandler;
}
public void UnRigisterJoyStickHandler(JoyStickHandler _onBeginHandler = null, JoyStickHandler _onMoveHandler = null, JoyStickHandler _onEndHandler = null)
{
if (_onBeginHandler != null)
beginHandler -= _onBeginHandler;
if (_onMoveHandler != null)
moveHandler -= _onMoveHandler;
if (_onEndHandler != null)
endHandler -= _onEndHandler;
}
public void OnDrag(PointerEventData data)
{
//limit radius
if (pot.anchoredPosition.magnitude > radius)
pot.anchoredPosition = pot.anchoredPosition.normalized * radius;
offset = pot.anchoredPosition3D;
if (offset.x < 1.0f && offset.x > -1.0f)
offset.x = 0.0f;
if (offset.y < 1.0f && offset.y > -1.0f)
offset.y = 0.0f;
}
public void OnBeginDrag(PointerEventData data)
{
hasMoving = true;
if (beginHandler != null)
beginHandler(this,new JoyStickArgs(offset.normalized));
}
public void OnEndDrag(PointerEventData data)
{
hasMoving = false;
if (endHandler != null)
endHandler(this, new JoyStickArgs(offset.normalized));
}
}
JoyStick 继承了 3个DragHandler 用于判断操作状态.
自定义了 一个 delegate 用于调用注册的物体事件.
摇杆UI的动作 都是用的ScrollRect 本身的特性,因此实现摇杆的代码非常简短,无需专门的代码控制摇杆的图片运动。
gif:
扫描二维码关注公众号,回复:
4098597 查看本文章
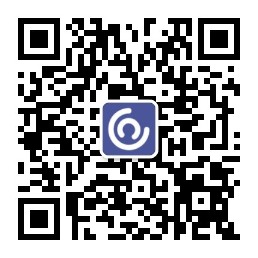