话不多说,直接上代码:
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.sofn</groupId> <artifactId>sofn-eletricity_business</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>Sofn-Eletricity_business</name> <description>Demo project for Spring Boot</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.3.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-aop</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jdbc</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-cache</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.3.0</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <!--<dependency>--> <!--<groupId>org.springframework.boot</groupId>--> <!--<artifactId>spring-boot-devtools</artifactId>--> <!--<optional>true</optional>--> <!--</dependency>--> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.0.26</version> </dependency> <dependency> <groupId> mysql</groupId> <artifactId> mysql-connector-java</artifactId> <version> 5.1.30</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.5.0</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.5.0</version> </dependency> <dependency> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-core</artifactId> <version>1.3.2</version> </dependency> <dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper</artifactId> <version>4.1.6</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <version>1.3.2</version> <executions> <execution> <id>generator</id> <goals> <goal>generate</goal> </goals> </execution> </executions> <configuration> <verbose>true</verbose> <overwrite>true</overwrite> <jdbcDriver>com.mysql.jdbc.Driver</jdbcDriver> <jdbcURL>jdbc:mysql://127.0.0.1/sofn_electricity_business</jdbcURL> <jdbcUserId>root</jdbcUserId> <jdbcPassword></jdbcPassword> </configuration> <dependencies> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <!-- 引用依赖库的版本 --> <version>5.1.30</version> </dependency> <dependency> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-core</artifactId> <version>1.3.2</version> </dependency> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <!-- 引用依赖库的版本 --> <version>3.4.2</version> </dependency> </dependencies> </plugin> </plugins> </build> </project>
application.properties
#开发环境 spring.datasource.name = dbName spring.datasource.url = jdbc:mysql://localhost:3306/dbName spring.datasource.username = root spring.datasource.password = spring.datasource.type = com.alibaba.druid.pool.DruidDataSource spring.datasource.driver-class-name = com.mysql.jdbc.Driver spring.datasource.filters = stat spring.datasource.maxActive = 20 spring.datasource.initialSize = 1 spring.datasource.maxWait = 60000 spring.datasource.minIdle = 1 spring.datasource.timeBetweenEvictionRunsMillis = 60000 spring.datasource.minEvictableIdleTimeMillis = 300000 spring.datasource.validationQuery = select 'x' spring.datasource.testWhileIdle = true spring.datasource.testOnBorrow = false spring.datasource.testOnReturn = false spring.datasource.poolPreparedStatements = true spring.datasource.maxOpenPreparedStatements = 20 mybatis.mapperLocations = classpath:mappers/generator/*.xml mybatis.typeAliasesPackage = com.sofn.model
UserMapper.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.sofn.mapper.generator.UserMapper" > <resultMap id="BaseResultMap" type="com.sofn.model.User" > <id column="id" property="id" jdbcType="INTEGER" /> <result column="user_name" property="userName" jdbcType="VARCHAR" /> <result column="passowrd" property="passowrd" jdbcType="VARCHAR" /> <result column="sex" property="sex" jdbcType="VARCHAR" /> <result column="age" property="age" jdbcType="INTEGER" /> <result column="phone" property="phone" jdbcType="VARCHAR" /> <result column="address" property="address" jdbcType="VARCHAR" /> <result column="qq" property="qq" jdbcType="VARCHAR" /> <result column="email" property="email" jdbcType="VARCHAR" /> <result column="createBy" property="createby" jdbcType="VARCHAR" /> <result column="createTime" property="createtime" jdbcType="TIMESTAMP" /> <result column="updateBy" property="updateby" jdbcType="VARCHAR" /> <result column="updateTime" property="updatetime" jdbcType="TIMESTAMP" /> <result column="isDelete" property="isdelete" jdbcType="VARCHAR" /> </resultMap> <delete id="deleteByPrimaryKey" parameterType="java.lang.Integer" > delete from user where id = #{id,jdbcType=INTEGER} </delete> <insert id="insert" parameterType="com.sofn.model.User" > insert into user (id, user_name, passowrd, sex, age, phone, address, qq, email, createBy, createTime, updateBy, updateTime, isDelete) values (#{id,jdbcType=INTEGER}, #{userName,jdbcType=VARCHAR}, #{passowrd,jdbcType=VARCHAR}, #{sex,jdbcType=VARCHAR}, #{age,jdbcType=INTEGER}, #{phone,jdbcType=VARCHAR}, #{address,jdbcType=VARCHAR}, #{qq,jdbcType=VARCHAR}, #{email,jdbcType=VARCHAR}, #{createby,jdbcType=VARCHAR}, #{createtime,jdbcType=TIMESTAMP}, #{updateby,jdbcType=VARCHAR}, #{updatetime,jdbcType=TIMESTAMP}, #{isdelete,jdbcType=VARCHAR}) </insert> <update id="updateByPrimaryKey" parameterType="com.sofn.model.User" > update user set user_name = #{userName,jdbcType=VARCHAR}, passowrd = #{passowrd,jdbcType=VARCHAR}, sex = #{sex,jdbcType=VARCHAR}, age = #{age,jdbcType=INTEGER}, phone = #{phone,jdbcType=VARCHAR}, address = #{address,jdbcType=VARCHAR}, qq = #{qq,jdbcType=VARCHAR}, email = #{email,jdbcType=VARCHAR}, createBy = #{createby,jdbcType=VARCHAR}, createTime = #{createtime,jdbcType=TIMESTAMP}, updateBy = #{updateby,jdbcType=VARCHAR}, updateTime = #{updatetime,jdbcType=TIMESTAMP}, isDelete = #{isdelete,jdbcType=VARCHAR} where id = #{id,jdbcType=INTEGER} </update> <select id="selectByPrimaryKey" resultMap="BaseResultMap" parameterType="java.lang.Integer" > select id, user_name, passowrd, sex, age, phone, address, qq, email, createBy, createTime, updateBy, updateTime, isDelete from user where id = #{id,jdbcType=INTEGER} </select> <select id="selectAll" resultMap="BaseResultMap" > select id, user_name, passowrd, sex, age, phone, address, qq, email, createBy, createTime, updateBy, updateTime, isDelete from user </select> <!-- 根据性别查询 --> <select id="findBySex" resultMap="BaseResultMap" parameterType="java.lang.String"> select id, user_name, passowrd, sex, age, phone, address, qq, email, createBy, createTime, updateBy, updateTime, isDelete from user where sex = #{sex,jdbcType=VARCHAR} </select> </mapper>
application.class
package com.sofn; import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.EnableAutoConfiguration; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.boot.web.servlet.ServletComponentScan; import org.springframework.boot.web.support.SpringBootServletInitializer; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.ImportResource; @SpringBootApplication @MapperScan("com.sofn.mapper") @ServletComponentScan("com.sofn.common") public class SofnEletricityBusinessApplication extends SpringBootServletInitializer { public static void main(String[] args) { SpringApplication.run(SofnEletricityBusinessApplication.class, args); } }
DruidDataSourceConfig
package com.sofn.common; import com.alibaba.druid.support.http.StatViewServlet; import com.alibaba.druid.support.http.WebStatFilter; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.web.servlet.FilterRegistrationBean; import org.springframework.boot.web.servlet.ServletRegistrationBean; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Primary; import org.springframework.transaction.annotation.EnableTransactionManagement; import com.alibaba.druid.pool.DruidDataSource; import javax.sql.DataSource; import java.sql.SQLException; /** Druid的DataResource配置类 * Created by wuye on 2017/4/17. */ @Configuration @EnableTransactionManagement public class DruidDataSourceConfig{ @Configuration public class DruidConfig { @Value("${spring.datasource.url}") private String dbUrl; @Value("${spring.datasource.username}") private String username; @Value("${spring.datasource.password}") private String password; @Value("${spring.datasource.driver-class-name}") private String driverClassName; @Value("${spring.datasource.initialSize}") private int initialSize; @Value("${spring.datasource.minIdle}") private int minIdle; @Value("${spring.datasource.maxActive}") private int maxActive; @Value("${spring.datasource.maxWait}") private int maxWait; @Value("${spring.datasource.timeBetweenEvictionRunsMillis}") private int timeBetweenEvictionRunsMillis; @Value("${spring.datasource.minEvictableIdleTimeMillis}") private int minEvictableIdleTimeMillis; @Value("${spring.datasource.validationQuery}") private String validationQuery; @Value("${spring.datasource.testWhileIdle}") private boolean testWhileIdle; @Value("${spring.datasource.testOnBorrow}") private boolean testOnBorrow; @Value("${spring.datasource.testOnReturn}") private boolean testOnReturn; @Value("${spring.datasource.poolPreparedStatements}") private boolean poolPreparedStatements; @Value("${spring.datasource.filters}") private String filters; @Bean public ServletRegistrationBean druidServlet() { ServletRegistrationBean reg = new ServletRegistrationBean(); reg.setServlet(new StatViewServlet()); reg.addUrlMappings("/druid/*"); reg.addInitParameter("loginUsername", "root"); reg.addInitParameter("loginPassword", "123456"); return reg; } @Bean public FilterRegistrationBean filterRegistrationBean() { FilterRegistrationBean filterRegistrationBean = new FilterRegistrationBean(); filterRegistrationBean.setFilter(new WebStatFilter()); filterRegistrationBean.addUrlPatterns("/*"); filterRegistrationBean.addInitParameter("exclusions", "*.js,*.gif,*.jpg,*.png,*.css,*.ico,/druid/*"); filterRegistrationBean.addInitParameter("profileEnable", "true"); filterRegistrationBean.addInitParameter("principalCookieName", "USER_COOKIE"); filterRegistrationBean.addInitParameter("principalSessionName", "USER_SESSION"); return filterRegistrationBean; } @Bean @Primary public DataSource druidDataSource(){ DruidDataSource datasource = new DruidDataSource(); datasource.setUrl(this.dbUrl); datasource.setUsername(username); datasource.setPassword(password); datasource.setDriverClassName(driverClassName); datasource.setInitialSize(initialSize); datasource.setMinIdle(minIdle); datasource.setMaxActive(maxActive); datasource.setMaxWait(maxWait); datasource.setTimeBetweenEvictionRunsMillis(timeBetweenEvictionRunsMillis); datasource.setMinEvictableIdleTimeMillis(minEvictableIdleTimeMillis); datasource.setValidationQuery(validationQuery); datasource.setTestWhileIdle(testWhileIdle); datasource.setTestOnBorrow(testOnBorrow); datasource.setTestOnReturn(testOnReturn); datasource.setPoolPreparedStatements(poolPreparedStatements); try { datasource.setFilters(filters); } catch (SQLException e) { //logger.error("druid configuration initialization filter", e); } return datasource; } } }
SessionFactoryConfig
package com.sofn.common; import org.apache.ibatis.session.SqlSessionFactory; import org.mybatis.spring.SqlSessionFactoryBean; import org.mybatis.spring.SqlSessionTemplate; import org.mybatis.spring.annotation.MapperScan; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.core.io.ClassPathResource; import org.springframework.core.io.support.PathMatchingResourcePatternResolver; import org.springframework.jdbc.datasource.DataSourceTransactionManager; import org.springframework.transaction.PlatformTransactionManager; import org.springframework.transaction.annotation.EnableTransactionManagement; import org.springframework.transaction.annotation.TransactionManagementConfigurer; import javax.sql.DataSource; /** * Created by wuye on 2017/4/18. */ @Configuration @EnableTransactionManagement @MapperScan("com.sofn.mapper.generator") public class SessionFactoryConfig implements TransactionManagementConfigurer { private static String MYBATIS_CONFIG = "mybatis-config.xml"; @Autowired private DataSource dataSource; private String typeAliasPackage = "com.sofn.model"; /** *创建sqlSessionFactoryBean 实例 * 并且设置configtion 如驼峰命名.等等 * 设置mapper 映射路径 * 设置datasource数据源 * @return * @throws Exception */ @Bean(name = "sqlSessionFactory") public SqlSessionFactoryBean createSqlSessionFactoryBean() throws Exception { PathMatchingResourcePatternResolver resolver = new PathMatchingResourcePatternResolver(); SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean(); /** 设置mybatis configuration 扫描路径 */ sqlSessionFactoryBean.setConfigLocation(new ClassPathResource(MYBATIS_CONFIG)); /** 设置datasource */ sqlSessionFactoryBean.setDataSource(dataSource); /** 设置typeAlias 包扫描路径 */ sqlSessionFactoryBean.setTypeAliasesPackage(typeAliasPackage); /** 设置扫描路径 */ sqlSessionFactoryBean.setMapperLocations(resolver.getResources("classpath:/mappers/generator/*.xml")); return sqlSessionFactoryBean; } @Bean public SqlSessionTemplate sqlSessionTemplate(SqlSessionFactory sqlSessionFactory) { return new SqlSessionTemplate(sqlSessionFactory); } @Bean @Override public PlatformTransactionManager annotationDrivenTransactionManager() { return new DataSourceTransactionManager(dataSource); } }
UserController
package com.sofn.controller; import com.sofn.model.User; import com.sofn.service.UserService; import io.swagger.annotations.ApiImplicitParam; import io.swagger.annotations.ApiImplicitParams; import io.swagger.annotations.ApiOperation; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.*; import java.util.List; /** * Created by wuye on 2017/4/17. */ @RestController @RequestMapping(value = "/user") public class UserController { @Autowired private UserService userService ; /** * 添加一个用户 * @param user * @return */ @ApiOperation(value = "添加一个用户",notes = "添加用户",httpMethod="POST") @ApiImplicitParam(name = "user", value = "用户详细实体user", required = true, dataType = "User") @RequestMapping(value = "/add",method = RequestMethod.POST) public String addUser(@RequestBody User user){ return "hello "+userService.add(user); } /** * 添加一个用户 * @return */ @ApiOperation(value = "添加一个用户",notes = "添加用户",httpMethod="POST") @ApiImplicitParams({ @ApiImplicitParam(name="userName",paramType="query",dataType="string"), @ApiImplicitParam(name="password",paramType="query",dataType="string") }) @RequestMapping(value = "/add2",method = RequestMethod.POST) public String addUser2(String userName,String password){ User user = new User(); user.setUserName(userName); user.setPassowrd(password); return "hello "+userService.add(user); } /** * 查询所有用户 * @return */ @ApiOperation(value = "查询所有用户",notes = "查询所有用户",httpMethod = "POST") @RequestMapping(value = "/all",method = RequestMethod.POST) public List<User> findAll(){ return userService.selectAll(); } /** * 根据ID查询 * @param userId * @return */ @ApiOperation(value = "根据ID查询",notes = "根据ID查询",httpMethod = "POST") @ApiImplicitParam(name="userId",paramType = "query",dataType = "string") @RequestMapping(value = "/findById",method = RequestMethod.POST) public User findById(String userId){ return userService.findUserById(Integer.valueOf(userId)); } /** * 根据ID删除 * @param userId * @return */ @ApiOperation(value = "根据ID删除",notes = "根据ID删除",httpMethod = "POST") @ApiImplicitParam(name="userId",paramType = "query",dataType = "string") @RequestMapping(value = "/delete",method = RequestMethod.POST) public void deleteById(String userId){ userService.deletByUserId(Integer.valueOf(userId)); } /** * 根据ID修改用户 * @param userId * @param sex * @param age * @param address * @return */ @ApiOperation(value = "根据ID修改",notes = "根据ID修改",httpMethod = "POST") @ApiImplicitParams({ @ApiImplicitParam(name = "userId",paramType = "query",dataType = "string"), @ApiImplicitParam(name = "sex",paramType = "query",dataType = "string"), @ApiImplicitParam(name = "age",paramType = "query",dataType = "string"), @ApiImplicitParam(name = "address",paramType = "query",dataType = "string") }) @RequestMapping(value = "/update",method = RequestMethod.POST) public int updateUser(String userId,String sex,String age,String address){ User user = userService.findUserById(Integer.valueOf(userId)); user.setSex(sex); user.setAge(Integer.valueOf(age)); user.setAddress(address); return userService.updateByUserId(user); } @ApiOperation(value = "根据性别查询",notes = "根据性别查询",httpMethod = "POST") @ApiImplicitParam(name = "sex",paramType = "query",dataType = "string") @RequestMapping(value = "/findBySex",method = RequestMethod.POST) public List<User> findBySex(String sex){ return userService.findBySex(sex); } // @ApiOperation(value = "获取用户信息列表",notes = "获取用户信息列表",httpMethod = "POST") // @RequestMapping("getPageInfo",method = RequestMethod.POST) // public List<User> getPageInfo(@ApiParam(required = true,value = "页数") @RequestParam(value = "draw" ,required = false) long drwa, // @ApiParam(required = true,value = "开始数") @RequestParam(value = "start" ,required = false) long start, // @ApiParam(required = true,value = "数量") @RequestParam(value = "length" ,required = false)long length){ // long recordsTotal = // Page pager = new Page(); // pager.setRecordsTotal(); // } }
UserMapper
package com.sofn.mapper.generator; import com.sofn.model.User; import com.sofn.util.generator.base.BaseMapper; import org.apache.ibatis.annotations.Mapper; import org.springframework.stereotype.Component; import java.util.List; @Mapper @Component public interface UserMapper extends BaseMapper { int deleteByPrimaryKey(Integer id); int insert(User record); User selectByPrimaryKey(Integer id); List<User> selectAll(); int updateByPrimaryKey(User record); /** * 根据性别查询 * @param sex * @return */ List<User> findBySex(String sex); }
UserServiceImpl
package com.sofn.service.impl; import com.sofn.mapper.generator.UserMapper; import com.sofn.mapper.sofn.UserExpandMapper; import com.sofn.model.User; import com.sofn.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import org.springframework.transaction.annotation.Transactional; import java.util.List; import java.util.Map; /** * Created by wuye on 2017/4/17. */ @Service @Transactional public class UserServiceImpl implements UserService { @Autowired private UserMapper userMapper; @Autowired private UserExpandMapper userExpandMapper; @Override public int deletByUserId(Integer id) { return userMapper.deleteByPrimaryKey(id); } @Override public int add(User record) { return userMapper.insert(record); } @Override public User findUserById(Integer id) { return userMapper.selectByPrimaryKey(id); } @Override public List<User> selectAll() { return userMapper.selectAll(); } @Override public int updateByUserId(User record) { userMapper.updateByPrimaryKey(record); return 0; } @Override public List<User> getPageInfo(Map<String, Object> map) { return userExpandMapper.getPageInfo(map); } @Override public long getCount(Map<String, Object> map) { return userExpandMapper.getCount(map); } @Override public List<User> findBySex(String sex) { return userExpandMapper.findBySex(sex); } }
扫描二维码关注公众号,回复:
408136 查看本文章
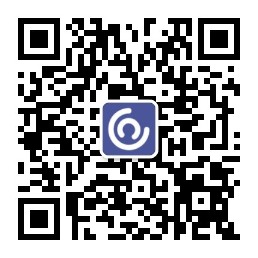