版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/majinlei121/article/details/83867361
首先导入import模块
import numpy as np
创建list和matrix
vector = np.array([5, 10, 15, 20]) # list
matrix = np.array([[5, 10, 15], [20, 25, 30], [35, 40, 45]]) # matrix
print vector
print matrix
#打印输出
[ 5 10 15 20]
[[ 5 10 15]
[20 25 30]
[35 40 45]]
求取矩阵的各种属性
print(vector.shape) #打印list形状
print(matrix.shape) #打印矩阵形状
#打印输出
(4L,)
(3L, 3L)
numbers = np.array([1, 2, 3, 4])
numbers.dtype #输出list的数据类型
#打印输出
dtype('int32')
提取矩阵元素
vector = numpy.array([5, 10, 15, 20])
print(vector[0:3])
#打印输出
[ 5 10 15]
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
print(matrix[:,1])
#打印输出
[10 25 40]
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
print(matrix[:,0:2])
[[ 5 10]
[20 25]
[35 40]]
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
print(matrix[1:3,0:2])
[[20 25]
[35 40]]
==操作
vector = numpy.array([5, 10, 15, 20])
vector == 10
#打印输出
array([False, True, False, False], dtype=bool)
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
matrix == 25
array([[False, False, False],
[False, True, False],
[False, False, False]], dtype=bool)
vector = numpy.array([5, 10, 15, 20])
equal_to_ten = (vector == 10)
print equal_to_ten
print(vector[equal_to_ten])
#打印输出
[False True False False]
[10]
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
second_column_25 = (matrix[:,1] == 25)
print second_column_25
print(matrix[second_column_25, :])
[False True False]
[[20 25 30]]
vector = numpy.array([5, 10, 15, 20])
equal_to_ten_and_five = (vector == 10) & (vector == 5)
print equal_to_ten_and_five
[False False False False]
vector = numpy.array([5, 10, 15, 20])
equal_to_ten_or_five = (vector == 10) | (vector == 5)
print equal_to_ten_or_five
[True True False False]
vector = numpy.array([5, 10, 15, 20])
equal_to_ten_or_five = (vector == 10) | (vector == 5)
vector[equal_to_ten_or_five] = 50
print(vector)
[50 50 15 20]
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
second_column_25 = matrix[:,1] == 25
print second_column_25
matrix[second_column_25, 1] = 10
print matrix
[False True False]
[[ 5 10 15]
[20 10 30]
[35 40 45]]
改变矩阵元素数据类型 astype
vector = numpy.array(["1", "2", "3"])
print vector.dtype
print vector
vector = vector.astype(float)
print vector.dtype
print vector
#打印输出
|S1
['1' '2' '3']
float64
[ 1. 2. 3.]
sum, axis = 0 列相加, axis = 1 行相加
vector = numpy.array([5, 10, 15, 20])
vector.sum()
50
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
matrix.sum(axis=1)
array([ 30, 75, 120])
matrix = numpy.array([
[5, 10, 15],
[20, 25, 30],
[35, 40, 45]
])
matrix.sum(axis=0)
array([60, 75, 90])
reshape(变形),shape(查看数组形状),ndim(维度),dtype(查看数据类型),size(总的元素个数),zeros(全0矩阵数组),ones(全1矩阵数组)
a = np.arange(15).reshape(3, 5) #arange生成0-14间隔为1的数组
a
array([[ 0, 1, 2, 3, 4],
[ 5, 6, 7, 8, 9],
[10, 11, 12, 13, 14]])
a.shape
(3, 5)
a.ndim
2
a.dtype.name
'int32'
a.size
15
np.zeros ((3,4))
array([[ 0., 0., 0., 0.],
[ 0., 0., 0., 0.],
[ 0., 0., 0., 0.]])
np.ones( (2,3,4), dtype=np.int32 )
array([[[1, 1, 1, 1],
[1, 1, 1, 1],
[1, 1, 1, 1]],
[[1, 1, 1, 1],
[1, 1, 1, 1],
[1, 1, 1, 1]]])
np.arange(a,b,n)生成间隔为n,数据范围为[a,b)的序列
np.arange( 10, 30, 5 )
array([10, 15, 20, 25])
np.arange( 0, 2, 0.3 )
array([ 0. , 0.3, 0.6, 0.9, 1.2, 1.5, 1.8])
np.arange(12).reshape(4,3)
array([[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8],
[ 9, 10, 11]])
np.random.random(a,b)(生成0-1之间矩阵大小为(a,b)随机数)
np.linspace(a,b,num)(生成a-b之间等间隔的num个数据)
np.random.random((2,3))
array([[ 0.40130659, 0.45452825, 0.79776512],
[ 0.63220592, 0.74591134, 0.64130737]])
from numpy import pi
np.linspace( 0, 2*pi, 100 )
array([ 0. , 0.06346652, 0.12693304, 0.19039955, 0.25386607,
0.31733259, 0.38079911, 0.44426563, 0.50773215, 0.57119866,
0.63466518, 0.6981317 , 0.76159822, 0.82506474, 0.88853126,
0.95199777, 1.01546429, 1.07893081, 1.14239733, 1.20586385,
1.26933037, 1.33279688, 1.3962634 , 1.45972992, 1.52319644,
1.58666296, 1.65012947, 1.71359599, 1.77706251, 1.84052903,
1.90399555, 1.96746207, 2.03092858, 2.0943951 , 2.15786162,
2.22132814, 2.28479466, 2.34826118, 2.41172769, 2.47519421,
2.53866073, 2.60212725, 2.66559377, 2.72906028, 2.7925268 ,
2.85599332, 2.91945984, 2.98292636, 3.04639288, 3.10985939,
3.17332591, 3.23679243, 3.30025895, 3.36372547, 3.42719199,
3.4906585 , 3.55412502, 3.61759154, 3.68105806, 3.74452458,
3.8079911 , 3.87145761, 3.93492413, 3.99839065, 4.06185717,
4.12532369, 4.1887902 , 4.25225672, 4.31572324, 4.37918976,
4.44265628, 4.5061228 , 4.56958931, 4.63305583, 4.69652235,
4.75998887, 4.82345539, 4.88692191, 4.95038842, 5.01385494,
5.07732146, 5.14078798, 5.2042545 , 5.26772102, 5.33118753,
5.39465405, 5.45812057, 5.52158709, 5.58505361, 5.64852012,
5.71198664, 5.77545316, 5.83891968, 5.9023862 , 5.96585272,
6.02931923, 6.09278575, 6.15625227, 6.21971879, 6.28318531])
numpy数学运算
扫描二维码关注公众号,回复:
4074040 查看本文章
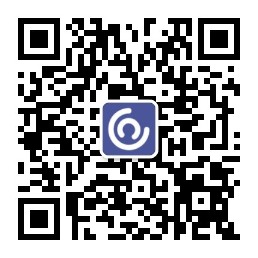
a = np.array( [20,30,40,50] )
b = np.arange( 4 )
print a
print b
c = a-b
print c
b**2
print b**2
print a<35
[20 30 40 50]
[0 1 2 3]
[20 29 38 47]
[0 1 4 9]
[True True False False]
A = np.array( [[1,1],
[0,1]] )
B = np.array( [[2,0],
[3,4]] )
print A
[[1 1]
[0 1]]
print B
[[2 0]
[3 4]]
print A*B # 矩阵对应元素相乘
[[2 0]
[0 4]]
print A.dot(B) # 矩阵相乘
[[5 4]
[3 4]]
print np.dot(A, B)
[[5 4]
[3 4]]
np.sqrt (开根号),np.floor(向下取整),ravel(拉伸为一维),.T(转置)
a = 10*np.random.random((3,4))
print a
[[ 2.82298425 7.14369753 8.69695365 3.25215523]
[ 4.05086915 6.52158344 7.14745109 3.37805305]
[ 5.08484091 7.04078148 7.7110627 4.13750478]]
a = np.floor(a)
print a
[[ 2. 7. 8. 3.]
[ 4. 6. 7. 3.]
[ 5. 7. 7. 4.]]
print a.ravel()
[ 2. 7. 8. 3. 4. 6. 7. 3. 5. 7. 7. 4.]
a.shape = (6, 2)
print a
[[ 2. 7.]
[ 8. 3.]
[ 4. 6.]
[ 7. 3.]
[ 5. 7.]
[ 7. 4.]]
print a.T
[[ 2. 8. 4. 7. 5. 7.]
[ 7. 3. 6. 3. 7. 4.]]
np.hstack (沿水平堆叠,增加列数)
np.vstack (沿竖直堆叠,增加行数)
a = np.floor(10*np.random.random((2,2)))
b = np.floor(10*np.random.random((2,2)))
print a
print '---'
print b
print '---'
print np.hstack((a,b))
print np.vstack((a,b))
[[ 0. 3.]
[ 2. 8.]]
---
[[ 3. 8.]
[ 5. 4.]]
---
[[ 0. 3. 3. 8.]
[ 2. 8. 5. 4.]]
[[ 0. 3.]
[ 2. 8.]
[ 3. 8.]
[ 5. 4.]]
np.hsplit (沿水平方向切分)
np.vsplit (沿竖直方向切分)
a = np.floor(10*np.random.random((2,12)))
print a
[[ 7. 3. 6. 8. 6. 0. 7. 6. 5. 4. 4. 5.]
[ 5. 6. 7. 8. 6. 9. 8. 8. 2. 3. 1. 5.]]
print np.hsplit(a,3) #水平方向切分,将12列切分为3份,每份4列
[array([[ 7., 3., 6., 8.],
[ 5., 6., 7., 8.]]), array([[ 6., 0., 7., 6.],
[ 6., 9., 8., 8.]]), array([[ 5., 4., 4., 5.],
[ 2., 3., 1., 5.]])]
print np.hsplit(a,(3,4)) # 在第3列后面切一刀,第4列后面切一刀
[array([[ 7., 3., 6.],
[ 5., 6., 7.]]), array([[ 8.],
[ 8.]]), array([[ 6., 0., 7., 6., 5., 4., 4., 5.],
[ 6., 9., 8., 8., 2., 3., 1., 5.]])]
a = np.floor(10*np.random.random((12,2)))
print a
[[ 2. 2.]
[ 4. 6.]
[ 1. 1.]
[ 5. 9.]
[ 5. 9.]
[ 0. 0.]
[ 6. 2.]
[ 6. 0.]
[ 0. 0.]
[ 7. 6.]
[ 7. 6.]
[ 0. 3.]]
print np.vsplit(a,3) #竖直方向切分,将12行切分为3份,每份4行
[array([[ 2., 2.],
[ 4., 6.],
[ 1., 1.],
[ 5., 9.]]), array([[ 5., 9.],
[ 0., 0.],
[ 6., 2.],
[ 6., 0.]]), array([[ 0., 0.],
[ 7., 6.],
[ 7., 6.],
[ 0., 3.]])]
复制操作
= 使用等号复制后两个数组完全相同,占用同样的地址空间
view 复制后两个数组不相同,但是,占用同样的地址空间
copy 复制一个和原数组不相关新数组,占用不同的地址空间
################# 等号复制 ############################
a = np.arange(12)
b = a
print b is a
b.shape = 3,4
print a.shape
print id(a)
print id(b)
True
(3L, 4L)
99466176
99466176
################# view复制 ############################
a = np.arange(12).reshape(3,4)
c = a.view()
print c is a
c.shape = 2,6
print a.shape
c[0,4] = 1234
print a
print id(a)
print id(c)
False
(3L, 4L)
[[ 0 1 2 3]
[1234 5 6 7]
[ 8 9 10 11]]
99466816
99464176
################# copy复制 ############################
a = np.arange(12).reshape(3,4)
print a
d = a.copy()
print d is a
d[0,0] = 9999
print d
print a
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]]
False
[[9999 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]]
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]]
argmax(axis=0)返回每一列最大位置的索引
data = np.sin(np.arange(20)).reshape(5,4)
print data
[[ 0. 0.84147098 0.90929743 0.14112001]
[-0.7568025 -0.95892427 -0.2794155 0.6569866 ]
[ 0.98935825 0.41211849 -0.54402111 -0.99999021]
[-0.53657292 0.42016704 0.99060736 0.65028784]
[-0.28790332 -0.96139749 -0.75098725 0.14987721]]
ind = data.argmax(axis=0)
print ind
[2 0 3 1]
data_max = data[ind, xrange(data.shape[1])]
print data_max
[ 0.98935825 0.84147098 0.99060736 0.6569866 ]
np.argsort(返回数组中从小到大的元素索引位置)
a = np.array([4, 3, 1, 2])
j = np.argsort(a)
print j
[2 3 1 0] #第一个元素2代表最小值1的索引,第二个元素3代表倒数第二小值2的索引
print a[j]
[1 2 3 4]
np.sort(a, axis=1) 对数组a进行排序,1是对每一行元素分别进行排序,0是对每一列元素分别进行排序
a = np.array([[4, 3, 5], [1, 2, 1]])
print a
[[4 3 5]
[1 2 1]]
print np.sort(a, axis=1)
[[3 4 5]
[1 1 2]]
print np.sort(a, axis=0)
[[1 2 1]
[4 3 5]]
np.title(复制多个输入数组)
a = np.arange(0, 40, 10)
print a
[ 0 10 20 30]
b = np.tile(a, (3, 5))
print b
[[ 0 10 20 30 0 10 20 30 0 10 20 30 0 10 20 30 0 10 20 30]
[ 0 10 20 30 0 10 20 30 0 10 20 30 0 10 20 30 0 10 20 30]
[ 0 10 20 30 0 10 20 30 0 10 20 30 0 10 20 30 0 10 20 30]]