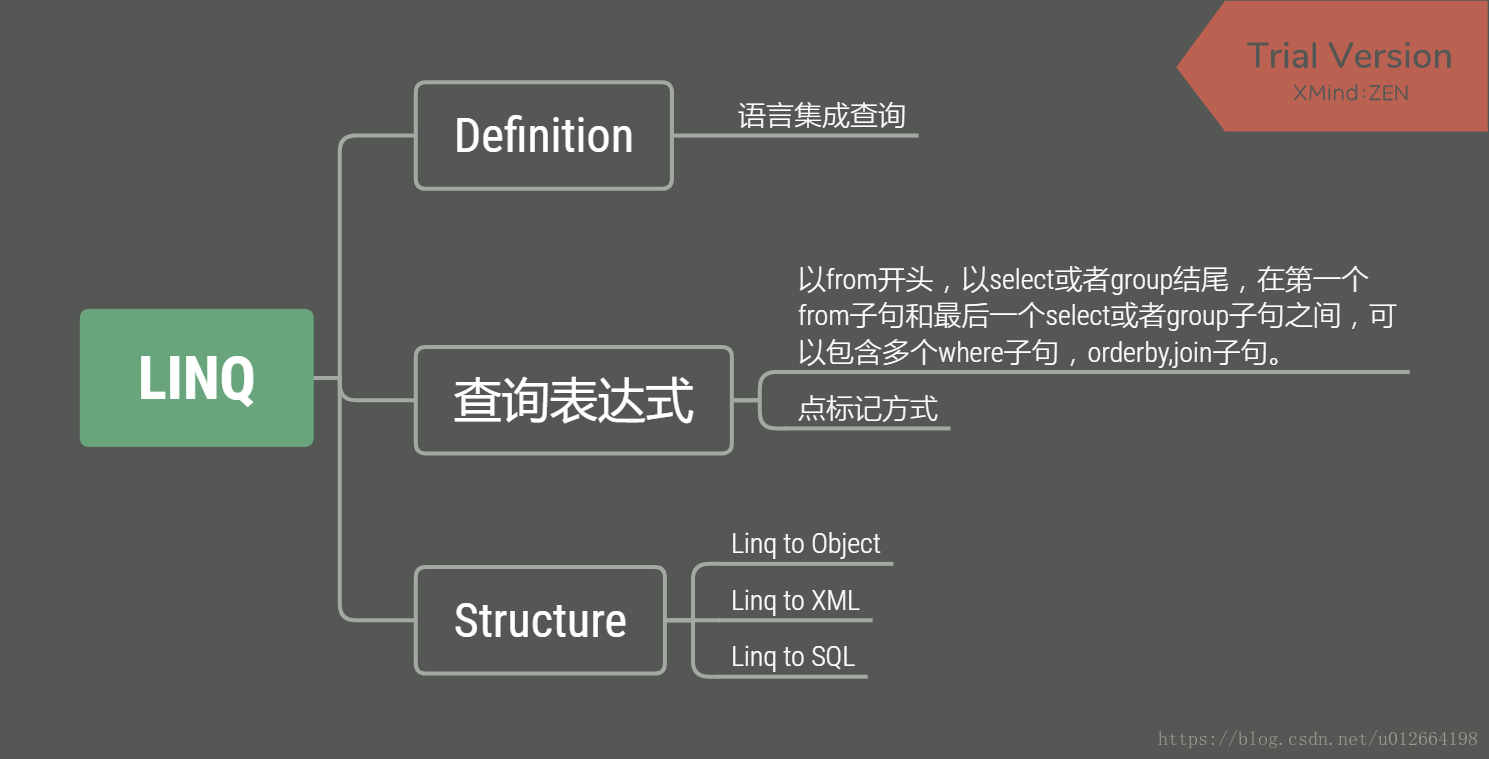
Demo
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml.Linq;
namespace LinqDemo
{
class Program
{
static void Main(string[] args)
{
var InputArray = new List<int>();
for(int i=1; i < 10; i++)
{
InputArray.Add(i);
}
Console.WriteLine("使用 Linq to Object 查询结果:");
LinqToObjectQuery(InputArray);
Console.WriteLine("使用 Linq to XML 查询结果:");
LinqToXML();
Console.Read();
}
/// <summary>
/// Linq Object 查询
/// </summary>
/// <param name="collection"></param>
private static void LinqToObjectQuery(List<int> collection)
{
var queryResult = from item in collection
where item % 2 == 0
select item;
foreach(var item in queryResult)
{
Console.Write(item + " ");
}
Console.WriteLine();
}
private static void LinqToXML()
{
//导入XML
XElement xmlDoc = XElement.Parse(xmlString);
var queryResult = from element in xmlDoc.Elements("Person")
where element.Element("Name").Value == "Allen"
select element;
foreach(var element in queryResult)
{
Console.WriteLine("Name:" + element.Element("Name").Value + " id为:" + element.Attribute("id").Value);
}
}
private static string xmlString =
"<Persons>" +
"<Person id='1'>" +
"<Name>Allen</Name>" +
"<Age>18</Age>" +
"</Person>" +
"<Person id='2'>" +
"<Name>Bill</Name>" +
"<Age>20</Age>" +
"</Person>" +
"</Persons>";
}
}