1. Jewels and Stones
- String的大小和访问string中的第i个char: s.size() ; s[i] . 其中s为string
- sort函数的用法:
- 必须声明头文件#include <algorithm>
- 三个input,第一个是排序的第一个元素的地址,第二个是排序的第二个的地址,第三个是排序方法 -- 通过bool的一个函数确定是从大到小还是从小到大。sort函数每次会把两个数值放进这个bool函数进去比大小。
- bool函数中写成const能避免不小心改动了a和b的值。 &在这里表引用。
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool cmp(const int &a, const int &b){
return a<b;
}
int main()
{
vector<int> myvec{1,2,7,6,1,7};
sort(myvec.begin(), myvec.end(), cmp);
cout << "The order is:" << endl;
for (int item:myvec)
cout << item << ' ';
cout << endl;
return 0;
}
- Lambda 表达式 -- C++11的新功能
- [capture list] (params list) mutable exception -> return type {function body}
- 注意单纯捕获一个值a在lambda里面也无法改变它的值,除非引用它(&a),或者加上mutable
- https://www.cnblogs.com/DswCnblog/p/5629165.html
sort(myvec2.begin(), myvec2.end(), [](int a, int b) -> bool { return a < b; });
- vector的用法
- 要包括头文件#include <vector>
- https://www.cnblogs.com/youyoui/p/5779965.html
- insert的用法: http://www.cplusplus.com/reference/vector/vector/insert/
myvec.push_back(3); myvec.pop_back();
- 几种常见容器
- list支持快速的插入和删除,但查找费时;vector支持快速查找,但插入费时;map查找的时间复杂度是对数,最快。hash也是对数。
- Hash表
- 二叉树和红黑树
- 二叉树:对比于有序数组,便于插入新的数据项;对比于链表,便于查找数据项。
- https://blog.csdn.net/eson_15/article/details/51138663
- 红黑树: http://www.cnblogs.com/skywang12345/p/3245399.html
- set和unordered_set
- unordered_set 的底层是hashtable,所以是无序的;set 的底层是RB-Tree,所以是有序的。
- https://blog.csdn.net/zhangxiao93/article/details/74357101
- count函数
- 返回统计容器中等于value的元素的个数
- https://blog.csdn.net/lyj2014211626/article/details/69615514
- 更好的解决方法:
2. To lower Case
3. Big Countries
- SQL的题目。用UNION两个索引同时进行,所以会比直接用OR快一些
- http://www.bubuko.com/infodetail-2253174.html
- SQL教程:http://www.w3school.com.cn/sql/index.asp
4. Unique Morse Code Words
- set用于存储不同的transformations.
5. Hamming Distance
- vector的几种初始化方式https://blog.csdn.net/yjunyu/article/details/77728410?locationNum=10&fps=1
- 位运算https://blog.csdn.net/fox64194167/article/details/20692645
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int main()
{
vector<int> v(32);
for(vector<int>::iterator i = v.begin();i!= v.end();++i)
{
cout << *i << " ";
}
cout << endl;
return 0 ;
}
6. Flipping an Image
- 遍历方法:for(vector<int> &vi:A) - vi为A中每项的遍历 https://zhidao.baidu.com/question/303137003.html
- 将vector vi反转: reverse(vi.begin(),vi.end());
- i^=1; - 对于i取反 (相当于if (i==0) {i = 1;} else {i=0;})
- 更好的解法:https://www.cnblogs.com/zhuangbijingdeboke/p/9192104.html
7. Judge Route Circle
8. Projection Area of 3D Shapes
- vector赋值
vector< vector<int> > grid(2, vector<int>(2));
int b[2][2]={{1,2},{3,4}};
for(int i=0; i<2;i++){
for(int j=0;j<2;j++){
grid[i][j] = b[i][j];
}
}
9. Merge Two Binary Trees
- TreeNode *newNode = new TreeNode(t1->val + t2->val);
10. Self Dividing Numbers
- 关键在于求余后判断是否余数能整除
11. Array Partition 1
扫描二维码关注公众号,回复:
3998906 查看本文章
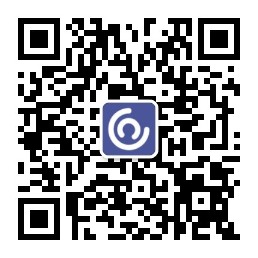
- 要使min的和最大就要按从小到大的顺序排列后两两作为一对,取前一个数字。(否则如果不这样取,就会导致大数被浪费)
- 第一个解法,直接sort完隔一个数取值相加
- 第二个解法把数值映射到一个reference table中,记录每个数出现的次数;然后从小到大通过flag来决定是要加还是不管。
12. Middle of the Linked List
- 关键在于设置两个指针同时跑,p2每次跑两格,p1每次跑一格,所以当p2跑完的时候p1就刚好在一半的位置。
13. Transpose Matrix
- 建一个新的向量矩阵,然后把A中的值一一拷贝到B内
14. Leaf-Similar Trees
- 关键在于写一个函数来收集全部的叶子 - 递归的方法,终止条件是左右指针都指向nullptr,然后每次都先找左边后找右边
- nullptr vs null: null是旧的已经被弃用的,它会被当作一个int;而nullptr是新的,它还是会被当成一个pointer。
- 树节点和一个vector被当作input放进函数里面的时候的表达方式如下,此时调用函数的时候直接输入树根和vector的名字就好,在函数里面也一样。
void leaves(TreeNode* root, vector<int>& leaf)
15. Number Complement
- ******位运算:https://blog.csdn.net/morewindows/article/details/7354571
- 更好的解法: https://www.cnblogs.com/grandyang/p/6275742.html
16. Uncommon Words
- unordered_map<string, int> 可以用来统计每个词出现的数量
- 用iter来做map的for循环
- istringstream的用法:https://blog.csdn.net/longzaitianya1989/article/details/52909786
class Solution {
public:
vector<string> uncommonFromSentences(string A, string B) {
istringstream wrdA(A);
istringstream wrdB(B);
vector<string> output;
unordered_map<string, int> words;
string word;
while(wrdA>>word){
words[word]++;
}
while(wrdB>>word){
words[word]++;
}
for(auto iter = words.begin(); iter!=words.end();iter++){
if(iter->second==1){
output.push_back(iter->first);
}
}
return output;
}
};
17. Reverse Words in a String |||
- istringstream读入每一个数,然后用reverse(tmp.begin(), tmp.end())实现反转,再重新存入一个string类型的result