Conditionals
Conditional expressions allow your programs to make decisions and take different forks in the road,depending on the value of variables or user input.
C provides a few different ways to implement conditional expressions (also know as branches ) in your programs,some of which likely look familiar from Scratch.
if (and if-else,and if-else..else)
Use Boolean expressions to make descisions.
if (boolean-expression)
{
statements;
}
if the boolean-expression evaluates to true,all lines of code between the curly braces will execute in order from top-to-bottom.
if the boolean-expression evaluates to false,those lines of code will not execute.
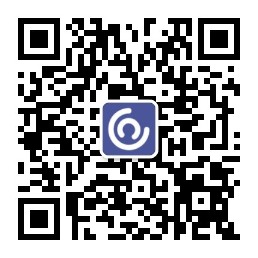
if (boolean-expression)
{
statements;
}
else
{
statements;
}
if the boolean-expression evaluates to true,all lines of code between the first set of curly braces will execute in order from top-to-bottom.
if the boolean-expression evaluates to false,all lines of code between the second set of curly braces will execute in order from top-to-bottom.
switch statement
Use discrete cases to make decisions
int x = GetInt();
switch(x)
{
case 1;
printf("One!\n");
break;
case 2:
printf("Two!\n");
break;
case 3:
printf("Three!\n");
break;
default:
printf("Sorry!\n");
}
C's switch() statement is a conditional statement that permits enumeration of discrete cases,instead of relying on Boolean expressions.
It's important to break;between each case,or you will "fall throug" each case (unless that is desired behavior).
The ternary operator (?:)
Use to replace a very simple if-else to make your code look fancy
cool but not a good idea
int x;
if (expr)
{
x = 5;
}
else
{
x = 5;
}
Next
int x = (expr) ? 5 : 6;
These two snippets of code act identically. // 这两段代码片段作用相同
The ternary operator (?:) is mostly a cute trick,but is useful for writing tricially short conditional branches.Be familiar with it,but know that you won't need to write it if you don't want to. // 三元操作符(?:)是一个不错的技巧