create database student
go
--创建表
--创建教师表
use student
go
create table teacher
(
tno char(4) constraint pk_tno primary key,
tname char(10) not null,
tsex char(2) constraint uk_xb check(tsex='男' or tsex='女'),
tbirthday datetime,
ttitle char(10)
)
go
insert into teacher
(tno,tname,tsex,tbirthday,ttitle)
values('2011','张华','男','1982-8-2','教授')
insert into teacher
(tno,tname,tsex,tbirthday,ttitle)
values('2012','潘强','男','1978-9-5','教授')
insert into teacher
(tno,tname,tsex,tbirthday,ttitle)
values('2013','王楠','男','1972-1-7','助教')
insert into teacher
(tno,tname,tsex,tbirthday,ttitle)
values('2014','马建','男','1989-12-3','教授')
insert into teacher
(tno,tname,tsex,tbirthday,ttitle)
values('2015','秦徐斌','男','1988-3-2','讲师')
--创建学生表
use student
go
create table student
(
sno char(10) constraint pk_sno primary key,
sname char(10) not null,
ssex char(2) constraint uk_stu check(ssex='男' or ssex='女'),
sbirthday datetime,
sscore numeric(18,0),
classno char(8),
st_mnt int
)
go
insert into student
values('0701011101','严金','男','1992-3-1',518,'07010211',0)
insert into student
values('0701011102','孙晓龙','女','1993-7-2',423,'07010211',0)
insert into student
values('0701011103','许盼盼','女','1992-8-1',432,'07010211',0)
insert into student
values('0701011104','贾思凡','女','1992-5-8',467,'07010211',0)
insert into student
values('0701011105','王赢','男','1994-3-8',398,'07010111',0)
insert into student
values('0701011106','东方红','男','1995-9-2',240,'07010111',0)
insert into student
values('0701011107','李莉','女','1992-10-2',420,'07010111',0)
insert into student
values('0601011101','张劲','男','1987-11-12',486,'06010111',0)
insert into student
values('0601011102','金伟','男','1988-4-14',487,'06010111',0)
insert into student
values('0601011103','李健','男','1987-6-29',476,'06010111',0)
insert into student
values('0601011104','王红青','女','1988-8-24',478,'06010111',0)
insert into student
values('0601011105','谭辉','男','1988-6-16',465,'06010111',0)
insert into student
values('0601011106','陆凤英','女','1988-1-7',480,'06010111',0)
insert into student
values('0601011107','胡涛','男','1987-7-15',462,'06010111',0)
--创建选修课程表
use student
go
create table choice
(
sno char(10) not null,
cno char(7) not null,
grade real
)
go
alter table choice
add constraint fk_choice
foreign key(sno) references student(sno)
insert into choice
values('0701011101','1001',90)
insert into choice
values('0701011101','1002',50)
insert into choice
values('0701011101','1003',70)
insert into choice
values('0701011101','1004',70)
insert into choice
values('0701011102','1001',93)
insert into choice
values('0701011102','1002',53)
insert into choice
values('0701011103','1001',90)
insert into choice
values('0701011104','1003',72)
insert into choice
values('0701011105','1003',60)
insert into choice
values('0701011106','1003',78)
insert into choice
values('0701011107','1003',50)
insert into choice
values('0701011107','1004',70)
insert into choice
values('0601011101','1002','')
--创建课程表
use student
create table course
(
cno char(7) constraint fk_co primary key,
cname char(30) not null,
credits real not null
)
insert into course
values('1001','Illustrator平面设计',90)
insert into course
values('1002','Photoshop图像处理',80)
insert into course
values('1003','Dreamweaver网页制作',70)
insert into course
values('1004','数据结构',60)
CREATE TABLE department
(
deptno char(2) constraint pk_dept primary key,
deptname char(20) not null
)
CREATE TABLE professional
(
pno char(4) constraint pk_pro primary key,
pname char(30) not null,
deptno char(2)
)
INSERT INTO department
VALUES('01','计算机工程系')
INSERT INTO department
VALUES('02','商贸管理系')
INSERT INTO department
VALUES('03','外语系')
INSERT INTO department
VALUES('04','机电工程系')
INSERT INTO department
VALUES('05','化学工程系')
INSERT INTO department
VALUES('06','物理系')
INSERT INTO department
VALUES('11','信息系')
INSERT INTO professional
VALUES('0101','计算机应用技术','01')
INSERT INTO professional
VALUES('0102','计算机网络技术','01')
INSERT INTO professional
VALUES('0201','物流管理','02')
INSERT INTO professional
VALUES('0202','会计','02')
INSERT INTO professional
VALUES('0301','德语','03')
INSERT INTO professional
VALUES('0302','商务英语','03')
INSERT INTO professional
VALUES('0401','模具设计与制造','04')
INSERT INTO professional
VALUES('0402','机电一体化技术','04')
INSERT INTO professional
VALUES('0501','有机化工生产技术','11')
INSERT INTO professional
VALUES('0502','精细化学品生产技术','11')
CREATE TABLE class
(
classno char(8) constraint pk_class primary key,
classname char(16) not null,
pno char(4) constraint fk_class references professional(pno)
)
INSERT INTO class
VALUES('07010211','网络0711','0102')
INSERT INTO class
VALUES('06010111','计应0611','0101')
INSERT INTO class
VALUES('06020111','物流0611','0201')
INSERT INTO class
VALUES('06020211','会计0611','0202')
INSERT INTO class
VALUES('07010111','计应0711','0501')
--实验7 数据查询(3)——查询排序与查询结果存储
--1. 查询课程信息,按课程名称降序排序
select *
from course
order by cname desc
--2. 查询选修了1001号课程成绩非空的学生学号和成绩,并按成绩降序排序
select student.sno,choice.grade
from student join choice
on student.sno=choice.sno
where cno='1001' and grade<>''
order by grade desc
--3. 查询11系学生学号、姓名和年龄,按年龄升序排序
select student.sno,sname,YEAR(getdate())-YEAR(sbirthday)
from student join class
on student.classno=class.classno
join professional
on class.pno=professional.pno
where deptno='11'
--实验8 数据查询(4)——查询统计与汇总
--4. 查询课程总数
select COUNT(*)
from course
--5. 查询选修1001号课程的学生人数
select COUNT(*)
from student
where sno in (select sno
from choice
where cno='1001')
--6. 查询被选修课程的数量
select COUNT(*)
from course
where cno in (select cno
from choice)
--7. 查询选修070101班学生的平均入学成绩
select AVG(sscore)
from student
where classno='07010211'
--8. 查询070101001号学生选修课程的数量、总分以及平均分
select COUNT(*),SUM(grade),AVG(grade)
from student join choice
on student.sno=choice.sno
where student.sno='0701011101'
--9. 查询选修1001号课程的学生人数、最高分、最低分和平均分
select COUNT(*),max(grade),MIN(grade)
from student join choice
on student.sno=choice.sno
where cno='1001'
--10. 求各个课程号和相应的选课人数
select cno,COUNT(*)
from choice join student
on choice.sno=student.sno
group by cno
--11. 依次按班级、系号对学生进行分类统计人数、入学平均分
select classno,COUNT(*),AVG(sscore)
from student
group by classno
select deptno,COUNT(*),AVG(sscore)
from student join class
on student.classno=class.classno
join professional
on class.pno=professional.pno
group by deptno
--12. 查询选修了均分在75以上的课程号及均分
select *
from
(select sno,AVG(grade)as score
from choice
group by sno)as a
where score>'75'
--13. 查询选修了2门以上课程的学生学号
select sno
from
(select student.sno,COUNT(*) as count
from student join choice
on student.sno=choice.sno
group by student.sno)as aa
where count>2
--14. 明细汇总年龄<20的学生,并汇总学生数量、平均年龄
select *
from student
where YEAR(getdate())-YEAR(sbirthday)<20
select COUNT(*),AVG(YEAR(getdate())-YEAR(sbirthday))
from student
--15. 按班级明细汇总成绩<85分的学生,汇总学生数、均分
select classno,COUNT(*),AVG(grade)
from student join choice
on student.sno=choice.sno
where grade<'80'
group by classno
--实验9 数据查询(5)——连接查询
--16. 用SQL Server形式连接查询学生学号、姓名、性别及其所选课程编号
select student.sno,sname,ssex,cno
from student join choice
on student.sno=choice.sno
order by sno
--17. 用SQL Server形式连接查询学生学号、姓名及其所选课程名称及成绩
select student.sno,sname,ssex,cname,grade
from student join choice
on student.sno=choice.sno
join course
on choice.cno=course.cno
--18. 查询选修了1002课程的学生学号、姓名及1001课程成绩
select student.sno,sname,cno,grade
from student join choice
on student.sno=choice.sno
where cno in('1001','1002')
--19. 查询选修了“数据结构”课程的学生学号、姓名及课程成绩
select student.sno,sname,ssex,grade
from student join choice
on student.sno=choice.sno
join course
on choice.cno=course.cno
where cname='数据结构'
--20. 用左外连接查询没有选修任何课程的学生学号、姓名
select student.sno,sname
from student left join choice
on student.sno=choice.sno
where choice.sno is null
--21. 用右外连接查询选修各个课程的学生学号
select distinct student.sno
from student right join choice
on student.sno=choice.sno
--实验10 数据查询(6)——数据更新与子查询
--22. 用子查询对各班人数进行查询(新增列)
select classno,COUNT(*)
from student
group by classno
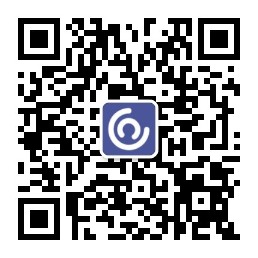
--23. 查询选修了1002课程成绩不及格的学生的学号、姓名和性别,并按姓名升序排序
select sno,sname,ssex
from student
where sno in(select sno
from choice
where grade<'60' and cno='1002')
order by sname asc
--24. 查询“东方红”同学所在班的学生信息,并按姓名降序排序
select *
from student
where classno=(select classno
from student
where sname='东方红')
order by sname desc
--25. 将有不及格成绩的学生的st_mnt值更改为3
update student
set st_mnt=3
where sno in (select sno
from choice
where grade<'60')
--26. 删除5系学生的选课信息
DELETE FROM choice WHERE sno in (select sno
from student
where classno in(select classno
from class
where pno in (select pno
from professional
where deptno='01')))
--1.自定义函数
--(1)创建自定义函数stu_sr,该函数可以变换出生日期字段的显示效果
use student
go
create function stu_sr(@stu_sb datetime) returns nvarchar(50)
as
begin
return STR(YEAR(@stu_sb))+'年'+LTRIM(STR(month(@stu_sb)))+'月'
end
go
alter function stu_sr(@stu_sb datetime) returns nvarchar(50)
as
begin
declare @sbyear nvarchar(10) =STR(YEAR(@stu_sb))
declare @sbmonth nvarchar(10) =LTRIM(STR(month(@stu_sb)))
if @sbmonth>9
return @sbyear+'年'+@sbmonth+'月'
else
return @sbyear+'年'+'0'+@sbmonth+'月'
return 0
end
go
select sno,dbo.stu_sr(sbirthday) as 出生年月,sbirthday
from student
--(2)创建自定义函数kc,要求可以查询选修了某课程的学生信息
use student
go
if exists(select *
from sysobjects
where name = 'kc'
and type='IF')
drop function kc
go
create function dbo.kc(@cname char(30)) returns table
as
return
(select student.sno
from student join choice
on student.sno=choice.sno
join course
on choice.cno=course.cno
where cname=@cname)
go
select *
from kc('Illustrator平面设计')
--2.存储过程
--创建存储过程kcgc,要求可以查询选修了某课程的学生信息
use student
go
if exists(select *
from sysobjects
where name = 'kcgc'
and type='P')
drop function kcgc
go
create proc kcgc
@cname char(30)
as
select student.sno
from student join choice
on student.sno=choice.sno
join course
on choice.cno=course.cno
where cname=@cname
go
execute kcgc'Illustrator平面设计'
--3.触发器
use student
go
--drop trigger delete_professional
create trigger delete_professional
on professional
for delete
as
if(select COUNT(*) from class join deleted
on class.pno=deleted.pno)>0
begin
print('该专业在班级表中,不可删除此记录!')
rollback transaction
end
else
print('记录已经删除')
go
delete professional
where pname='计算机应用技术'