前言
本文是dubbo系列的第一篇,在正式讲解dubbo的源码之前,需要搭建一套dubbo的样例,用于我们阅读源码以及理解dubbo的核心功能,本文是以springboot为基础的,其他的通过xml配置的太麻烦了。
版本说明
springboot starter : 0.1.1
dubbo版本: 2.6.2
新建项目
新建项目dubbo-provider, dubbo-service,dubbo-interface,dubbo-consumer这四个项目,项目结构如下
pom配置
dubbo-provider pom.xml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.4.RELEASE</version>
</parent>
<properties>
<dubbo-spring-boot>1.0.0</dubbo-spring-boot>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.14.8</version>
</dependency>
</dependencies>
</dependencyManagement>
dubbo-service 和dubbo-consumer的pom文件相同 pom.xml
<dependencies>
<!-- Spring Boot Dubbo 依赖 -->
<dependency>
<groupId>com.alibaba.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>0.1.1</version>
</dependency>
<!-- Spring Boot Web 依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Test 依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<dependency>
<artifactId>dubbo-interface</artifactId>
<version>1.0-SNAPSHOT</version>
<groupId>com.dubbo</groupId>
</dependency>
</dependencies>
dubbo-interface pom文件
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.5.1</version>
<configuration>
<source>${java.version}</source>
<target>${java.version}</target>
<testSource>${java.version}</testSource>
<testTarget>${java.version}</testTarget>
</configuration>
</plugin>
</plugins>
</build>
编码
dubbo-service
启动类
@SpringBootApplication
@DubboComponentScan("com.dubbo.provider.facade")
public class ProviderApplication {
public static void main(String[] args) {
SpringApplication.run(ProviderApplication.class);
}
}
application.yml文件
spring:
application:
name: dubbo-service
profiles:
active: dev
server:
port: 7100
dubbo:
application:
name: dubbo-service
registry:
address: zookeeper://localhost:2181
protocol:
name: dubbo
port: 20880
装饰层
@Service 注解是**com.alibaba.dubbo.config.annotation.Service ** 的,不是spring提供的
扫描二维码关注公众号,回复:
3900144 查看本文章
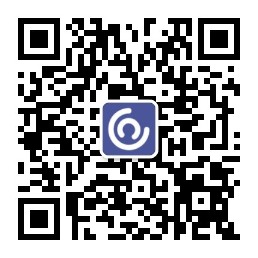
/**
* @Author 张云和
* @Date 2018/9/11
* @Time 14:48
*/
@Service
public class UserFacade implements UserFacadeService {
@Resource
private UserService userService;
@Override
public UserDTO getUser(Integer userId) {
UserPO userPO = userService.getUser(userId);
if(null==userPO){
return null;
}
UserDTO userDTO = new UserDTO();
userDTO.setUserId(userPO.getUserId());
userDTO.setUserName(userPO.getUserName());
return userDTO;
}
}
UserFacadeService 为暴露出去的接口服务,在dubbo-interface中进行定义,方便消费者和生产者都能引用到
内部service层
@Service // 这个注解是spring的注解,不是dubbo的
public class UserServiceImpl implements UserService {
@Override
public UserPO getUser(Integer userId) {
// 此处没有从数据库查询
UserPO userPO = new UserPO();
userPO.setUserId(userId);
userPO.setUserName("测试用户:"+userId);
userPO.setLastLoginTime(new Date());
userPO.setPassword("password");
return userPO;
}
}
dubbo-consumer
启动类
@SpringBootApplication
@DubboComponentScan("com.dubbo.consumer.service")
public class ConsumerApplication {
public static void main(String[] args) {
SpringApplication.run(ConsumerApplication.class);
}
}
建立一个service类,用来引用dubbo-service中提供的接口服务
@Service // spring的
public class UserService implements UserFacadeService{
@Reference // dubbo的接口引用注解
private UserFacadeService userFacadeService;
@Override
public UserDTO getUser(Integer userId) {
return userFacadeService.getUser(userId);
}
}
建立controller用来测试接口
@RestController
public class TestController {
@Resource
private UserService userService;
@RequestMapping("getUser")
public UserDTO getUser(Integer userId){
return userService.getUser(userId);
}
}
application.yml
spring:
application:
name: dubbo-consumer
profiles:
active: dev
server:
port: 7101
dubbo:
application:
name: dubbo-consumer
registry:
address: zookeeper://localhost:2181
protocol:
name: dubbo
port: 20880
测试
打开浏览器,访问http://localhost:7101/getUser?userId=1 ,得到结果
{"userId":1,"userName":"测试用户:1"}
本文搭建demo的项目结构是按照我正式生产环境进行搭的,所以跟其他博客上的项目结构有些区别,不是那么简单,有需要demo的可以关注公众号私信我! 一起交流学习!