一.页面展示时间类型数据
1.引入头文件:<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt"%>
2.将从后台取出的时间类型数据按yyyy-MM-dd格式,格式化;
<td><fmt:formatDate value="${u.birthday }" pattern="yyyy-MM-dd"/> </td>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
function deleteUser(userId,userName){
if(window.confirm("您确定要删除用户["+userName+"]吗?")){
location.href="${pageContext.request.contextPath }/user/"+userId+"/delete.action";
}
}
</script>
</head>
<body>
<h1>用户列表</h1>
<table border="1" cellpadding="0" cellspacing="0">
<tr>
<th><input type="checkbox"/></th>
<th>编号</th>
<th>姓名</th>
<th>生日</th>
<th>性别</th>
<th>地址</th>
<th>操作</th>
</tr>
<c:forEach items="${users }" var="u" varStatus="status">
<c:if test="${status.index%2==0 }">
<tr style="background-color: #ccc">
</c:if>
<c:if test="${status.index%2!=0 }">
<tr>
</c:if>
<td><input type="checkbox" value="${u.id }" name="uid"/></td>
<td>${u.id }</td>
<td>${u.username }</td>
<td><fmt:formatDate value="${u.birthday }" pattern="yyyy-MM-dd"/> </td>
<td>${u.sex==1?'男':'女' }</td>
<td>${u.address }</td>
<td>
<a href="">查看</a>/
<a href="${pageContext.request.contextPath }/editUser.action?id=${u.id }">修改</a>/
<a onclick="deleteUser(${u.id},'${u.username} }')" href="javascript:void(0)">删除</a>
</td>
</tr>
</c:forEach>
</table>
</body>
</html>
二.后台时间类型字段接收前台传来的时间字符串
1.自定义时间字符串转换类DateConverter继承 Converter<String, Date>
注明:Converter这个类可以转换任何类型,用的最多的是时间转换;
package com.igeek.ssm.ex.converters;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.springframework.core.convert.converter.Converter;
/**
* @author www.igeehome.com
*
* TODO
*
* 2018年10月24日下午7:57:37
*/
public class DateConverter implements Converter<String, Date> {
@Override
public Date convert(String dateStr) {
Date date = null;
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
date = dateFormat.parse(dateStr);
} catch (ParseException e) {
//e.printStackTrace();
dateFormat = new SimpleDateFormat("yyyy-MM-dd");
try {
date = dateFormat.parse(dateStr);
} catch (ParseException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
return date;
}
}
2.springMVC.xml中配置:
<!-- 配置注解驱动 -->
<!-- 如果配置此标签,可以不用配置... -->
<mvc:annotation-driven conversion-service="conversionService" />
<!-- 配置类型转换器 -->
<bean id="conversionService"
class="org.springframework.format.support.FormattingConversionServiceFactoryBean">
<property name="converters">
<set>
<bean class="com.igeek.ssm.ex.converters.DateConverter" />
</set>
</property>
</bean>
3.页面:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>添加用户</title>
</head>
<body>
<form action="${pageContext.request.contextPath }/addUser.action" method="post">
<p>用户名:<input type="text" name="username"/></p>
<p>生日:<input type="date" name="birthday"/></p>
<p>性别:<input type="radio" name="sex" value="1"/>男
<input type="radio" name="sex" value="2"/>女</p>
<p>地址:<input type="text" name="address"/></p>
<p><input type="submit" value="添加"/></p>
</form>
</body>
</html>
4.后台接收:
@RequestMapping("/addUser")
public String addUser(User user) {
userService.saveUser(user);
// 无论是否成功,进入列表页面
// 重定向进入url:userList.action
return "redirect:userList.action";
}
5.实体类:
扫描二维码关注公众号,回复:
3896437 查看本文章
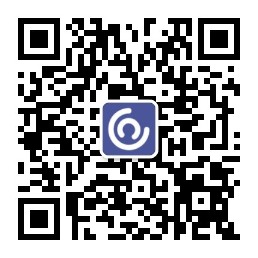
package com.igeek.ssm.ex.pojo;
import java.util.Date;
/**
* @author www.igeehome.com
*
* TODO
*
* 2018年10月29日下午7:48:30
*/
public class User implements java.io.Serializable{
private int id;
private String username;
private Date birthday;
private int sex;
private String address;
/**
* @return the id
*/
public int getId() {
return id;
}
/**
* @param id the id to set
*/
public void setId(int id) {
this.id = id;
}
/**
* @return the username
*/
public String getUsername() {
return username;
}
/**
* @param username the username to set
*/
public void setUsername(String username) {
this.username = username;
}
/**
* @return the birthday
*/
public Date getBirthday() {
return birthday;
}
/**
* @param birthday the birthday to set
*/
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
/**
* @return the sex
*/
public int getSex() {
return sex;
}
/**
* @param sex the sex to set
*/
public void setSex(int sex) {
this.sex = sex;
}
/**
* @return the address
*/
public String getAddress() {
return address;
}
/**
* @param address the address to set
*/
public void setAddress(String address) {
this.address = address;
}
}