Springboot中集成了@Async注解,我们在使用的时候直接用就好了.
不需要获取到返回值
如果只是单纯的让线程去异步执行,不需要返回结果的话,如下示例,直接进行..单线程进行的话,至少需要4+3+2=9秒钟,而我们不考虑运行的结果,只是让他去运行的话,那么肯定在他们执行之前执行完成,
后来打印了一下时间,好像就用了4ms.所以,多线程异步还是挺6的....
package com.trs.idap.service;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Component;
/**
* Created by Administrator on 2018/10/11.
* 描述:
*
* @author Young
* @create 2018-10-11 19:48
*/
@Component
@Async
public class TestAsyncService {
@Async
public void execAsync1(){
try{
Thread.sleep(2000);
System.out.println("甲睡了2000ms");
}catch (Exception e){
e.printStackTrace();
}
}
@Async
public void execAsync2(){
try{
Thread.sleep(4000);
System.out.println("乙睡了4000ms");
}catch (Exception e){
e.printStackTrace();
}
}
@Async
public void execAsync3(){
try{
Thread.sleep(3000);
System.out.println("丙睡了3000ms");
}catch (Exception e){
e.printStackTrace();
}
}
}
package com.trs.idap.web.rest.controller;
import com.trs.idap.service.TestAsyncService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* Created by Administrator on 2018/10/11.
* 描述:
* @author Young
* @create 2018-10-11 19:46
*/
@RequestMapping("/api")
@RestController
public class TestAsync {
@Autowired
private TestAsyncService testAsyncService;
@RequestMapping("execAsync")
public void execAsync(){
testAsyncService.execAsync1();//2s
testAsyncService.execAsync2();//4s
testAsyncService.execAsync3();//3s
System.out.println("我执行结束了");
}
}
扫描二维码关注公众号,回复:
3846100 查看本文章
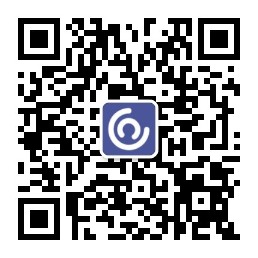
需要返回值
需要返回值的情况也差不多,可以理解为,一次性把任务分发出去.然后任务各自去执行,这时分发任务后可以返回一个Future,我们可以理解为在饭店做饭给的小票,等饭好了凭小票去拿饭...
等我们做完其他的事情的时候,再来收集这些返回值.通过Future的get()方法,如果该Future对应的方法还没有执行完,或者没有结果,此时get()会阻塞在这里,直到拿到结果值为止....
Futurn还有一个isDone()方法,只是判断该Future对应的任务有没有执行完成,和get()大同小异了..看你是想拿到结果,还是想看任务是否执行成功.
所以,我们看下,单线程同步的话,至少需要9s+其他处理时间,而现在是最大任务的时间+其他处理时间.此时很明显的看清楚,9s(所有任务的时间和)是远远比最大任务时间要多的多....所以这就是效率....也刚起步,慢慢积累,接收批评.
package com.trs.idap.web.rest.controller;
import com.trs.idap.service.TestAsyncService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
/**
* Created by Administrator on 2018/10/11.
* 描述:
* @author Young
* @create 2018-10-11 19:46
*/
@RequestMapping("/api")
@RestController
public class TestAsync {
@Autowired
private TestAsyncService testAsyncService;
@RequestMapping("execAsync")
public void execAsync() throws ExecutionException, InterruptedException {
long l = System.currentTimeMillis();
Future<String> stringFuture = testAsyncService.execAsync1();//2s
Future<String> stringFuture1 = testAsyncService.execAsync2();//4s
Future<String> stringFuture2 = testAsyncService.execAsync3();//3s
//此时这里的get方法调用了线程的阻塞,可以不用isDone进行判断.
//如果Future还没有值,线程是阻塞的,直到获取到值时结束
String s = stringFuture.get();
String s1 = stringFuture1.get();
String s2 = stringFuture2.get();
System.out.println("执行结束....."+s+s1+s2);
System.out.println("我执行结束了:"+(System.currentTimeMillis()-l));
}
}
package com.trs.idap.service;
import org.springframework.scheduling.annotation.Async;
import org.springframework.scheduling.annotation.AsyncResult;
import org.springframework.stereotype.Component;
import java.util.concurrent.Future;
/**
* Created by Administrator on 2018/10/11.
* 描述:
*
* @author Young
* @create 2018-10-11 19:48
*/
@Component
@Async
public class TestAsyncService {
@Async
public Future<String> execAsync1(){
try{
Thread.sleep(2000);
System.out.println("甲睡了2000ms");
return new AsyncResult<String>("甲执行成功了");
}catch (Exception e){
e.printStackTrace();
}
return null;
}
@Async
public Future<String> execAsync2(){
try{
Thread.sleep(4000);
System.out.println("乙睡了4000ms");
return new AsyncResult<String>("乙执行成功了");
}catch (Exception e){
e.printStackTrace();
}
return null;
}
@Async
public Future<String> execAsync3(){
try{
Thread.sleep(3000);
System.out.println("丙睡了3000ms");
return new AsyncResult<String>("丙执行结束了");
}catch (Exception e){
e.printStackTrace();
}
return null;
}
}