关键:找一个容器存储可换位置的字母
1. 自己
使用数组存储
class Solution {
public String reverseVowels(String s) {
int[] map= new int[256];
map['a']= map['A']= 1;
map['e']= map['E']= 1;
map['i']= map['I']= 1;
map['o']= map['O']= 1;
map['u']= map['U']= 1;
char[] tmp= s.toCharArray();
int st= 0, e= tmp.length-1;
while(st< e){
if(map[tmp[st]]==1 && map[tmp[e]]==1){
char ch= tmp[st];
tmp[st]= tmp[e];
tmp[e]= ch;
st++;e--;
}if(map[tmp[st]]==0){
st++;
}if(map[tmp[e]]==0){
e--;
}
}
return new String(tmp);
}
}
2. 参考
用set存贮
public class Solution {
public String reverseVowels(String s) {
char[] list=s.toCharArray();
Set<Character> set=new HashSet<>();
set.add('a');
set.add('e');
set.add('i');
set.add('o');
set.add('u');
set.add('A');
set.add('E');
set.add('I');
set.add('O');
set.add('U');
for (int i=0, j=list.length-1; i<j; ) {
if (!set.contains(list[i])) {
i++;
continue;
}
if (!set.contains(list[j])) {
j--;
continue;
}
char temp=list[i];
list[i]=list[j];
list[j]=temp;
i++;
j--;
}
return String.valueOf(list);
}
}
或用另一种方式创建含值的set:
Set<Character> vowels = new HashSet<>(Arrays.asList(new Character[]{'a','e','i','o','u','A','E','I','O','U'}));
3. 参考
使用string存储,contains判断
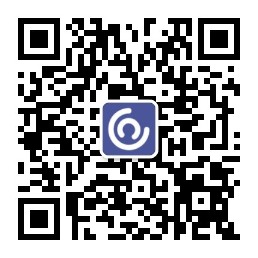
public class Solution {
public String reverseVowels(String s) {
if(s == null || s.length()==0) return s;
String vowels = "aeiouAEIOU";
char[] chars = s.toCharArray();
int start = 0;
int end = s.length()-1;
while(start<end){
while(start<end && !vowels.contains(chars[start]+"")){
start++;
}
while(start<end && !vowels.contains(chars[end]+"")){
end--;
}
char temp = chars[start];
chars[start] = chars[end];
chars[end] = temp;
start++;
end--;
}
return new String(chars);
}