版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/Jacky_Ponder/article/details/53991298
一个C语言的工程中如何调用C++写成的代码呢?最简单的方法当然是使用g++编译C工程,但有时候可能因为一些限制不能使用g++编译,这时候可以考虑把C++代码Make成一个动态库或静态库,再在C代码中调用。
1.动态库的创建
如下有一个调用opencv的程序:
facedetect.h:
#include "cv.h"
#include "highgui.h"
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <assert.h>
#include <math.h>
#include <float.h>
#include <limits.h>
#include <time.h>
#include <ctype.h>
#ifdef __cplusplus
extern "C"{
#endif
int facedetect(char* file_name);
#ifdef __cplusplus
}
#endif
facedetect.cpp:
#include"facedetect.h"
#ifdef __cplusplus
extern "C"{
#endif
int facedetect(char* file_name)
{
CvMemStorage* storage = 0;
CvHaarClassifierCascade* cascade = 0;
static const char* cascade_name;
cascade_name = "haarcascade_frontalface_alt2.xml";
if(cascade_name){
printf("cascade_name:%s\n", cascade_name);
}
else{
printf("cascade_name:NULL\n");
}
if(file_name){
printf("%s\n", file_name);
}
cascade = (CvHaarClassifierCascade*)cvLoad( cascade_name, 0, 0, 0 );
storage = cvCreateMemStorage(0);
IplImage* image = cvLoadImage( file_name, 1 );
if( image ){
double scale = 1.3;
IplImage* gray = cvCreateImage( cvSize(image->width,image->height), 8,
1 );
IplImage* small_img = cvCreateImage( cvSize( cvRound (image->width/scale),
cvRound (image->height/scale)),
8, 1 );
cvCvtColor( image, gray, CV_BGR2GRAY );
cvResize( gray, small_img, CV_INTER_LINEAR );
cvEqualizeHist( small_img, small_img );
cvClearMemStorage( storage );
if( cascade ){
double t = (double)cvGetTickCount();
CvSeq* faces = cvHaarDetectObjects( small_img, cascade, storage,
1.1, 2, 0/*
CV_HAAR_DO_CANNY_PRUNING*/,
cvSize(30, 30) );
t = (double)cvGetTickCount() - t;
printf( "detection time = %gms\n", t/((double)cvGetTickFrequency()*
1000.) );
printf("%d\n", faces ? faces->total : 0);
}
cvReleaseImage( &gray );
cvReleaseImage( &small_img );
cvReleaseImage( &image );
}
}
#ifdef __cplusplus
}
#endif
首先通过facedetect.cpp编译出libfacedetect.so
扫描二维码关注公众号,回复:
3811575 查看本文章
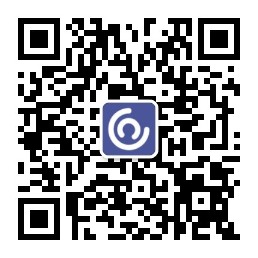
#g++ facedetect.cpp -shared -fPIC -o libfacedetect.so `pkg-config --cflags --libs opencv`
如果需要进行交叉编译则
#arm-hisiv100nptl-linux-g++ facedetect.cpp -shared -fPIC -o libfacedetect-arm.so `pkg-config --cflags --libs opencv`
2.动态库的调用示例
test.c:
#include "facedetect.h"
int main()
{
facedetect("lena.jpg");
return 0;
}
然后编译
#gcc test.c -o test ./libfacedetect.so `pkg-config --cflags --libs opencv` -lstdc++
其中-lstdc++ 所对应的是标准C++库
执行
./test