pthread_detach函数
功能:分离线程
int pthread_detach(pthred_t thread);
返回值:若成功,返回0,否则,返回错误编号
- 线程分离状态:指定该线程‘线程主动与主控线程断开关系,线程结束后,其退出状态不由其他线程获取,而直接自己主动释放,网络、多线程服务器常用。
- 进程若有该机制,将不会产生僵尸进程,僵尸进程的产生主要是由于进程死后,大部分资源被释放,一点残留资源仍然存于系统中,导致内核认为该进程仍然存在。
- 也可以用pthread_create函数参数2(线程属性)来设置线程分离。
测试代码:
#include<unistd.h>
#include<string.h>
#include<pthread.h>
#include<stdio.h>
void *tfn(void *arg)
{
int n = 3;
while(n--) {
printf("thred count %d\n", n);
sleep(1);
}
}
int main()
{
pthread_t tid;
void *tret;
int err;
pthread_create(&tid, NULL, tfn, NULL);
pthread_detach(tid);
while(1) {
err = pthread_join(tid, &tret);
printf("-----------------------err = %d\n", err);
if(err != 0)
fprintf(stderr, "thread_join error: %s\n", strerror(err));
else
fprintf(stderr, "thread_exit code %d\n", (int) tret);
sleep(1);
}
return 0;
}
输出结果:
测试代码:
#include<unistd.h>
#include<string.h>
#include<pthread.h>
#include<stdio.h>
void *tfn(void *arg)
{
int n = 3;
while(n--) {
printf("thred count %d\n", n);
sleep(1);
}
}
int main()
{
pthread_t tid;
void *tret;
int err;
pthread_create(&tid, NULL, tfn, NULL);
while(1) {
err = pthread_join(tid, &tret);
printf("-----------------------err = %d\n", err);
if(err != 0)
fprintf(stderr, "thread_join error: %s\n", strerror(err));
else
fprintf(stderr, "thread_exit code %d\n", (int) tret);
sleep(1);
}
return 0;
}
输出结果:
pthread_cancel函数
扫描二维码关注公众号,回复:
3680338 查看本文章
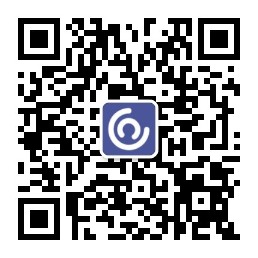
功能:杀死(取消)线程
#include<pthread.h。
int pthread_cancel(othread_t thread);
返回值:若成功,返回0, 否则,返回错误
【注意】:线程取消并不是实时的,而有一定的延时, 需要等待线程到达某个取消点(检查点)
类似玩游戏存档,必须达到指定的场所(存档点, 如:客栈、仓库、城里等)才能存储进度。杀死线程也不是立刻就能完成 ,必须到达取消点。
取消点:是线程检查是否被取消,并按请求进行工作的一个位置。通常是一个系统调用creat,open、pause、close、read、write执行命令man 7 pthreads可以查看具备这些取消点的系统调用列表。
可以粗略认为一个系统调用(进入内核)即为一个取消点。如线程中没有取消点,可以通过调用pthread_testcancl函数自行设置一个取消点。
#include<stdio.h>
#include<unistd.h>
#include<pthread.h>
#include<stdlib.h>
void *tfn1(void *arg)
{
printf("thread 1 returning\n");
return (void*) 111;
}
void *tfn2(void *arg)
{
printf("thread 2 exiting\n");
pthread_exit((void *) 222);
}
void *tfn3(void *arg)
{
while(1) {
printf("thread 3: I'm going to die in 3 seconds ....\n");
sleep(1) ;
}
return (void*) 666;
}
int main()
{
pthread_t tid;
void *tret = NULL;
pthread_create(&tid, NULL, tfn1, NULL);
pthread_join(tid, &tret);
printf(" thread 1 exit code = %d\n\n", (int)tret);
pthread_create(&tid, NULL, tfn2, NULL);
pthread_join(tid, &tret);
printf(" thread 2 exit code = %d\n\n", (int)tret);
pthread_create(&tid, NULL, tfn3, NULL);
sleep(3);
pthread_cancel(tid);
pthread_join(tid, &tret);
printf("thread 3 exit code = %d\n", (int)tret);
return 0;
}
输出结果: