版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/qq_41026740/article/details/83099786
HTTP网络通信编码流程
短连接:即非持久连接,指客户端和服务器进行一次HTTP请求/响应之后就关闭连接。所以下一次的HTTP请求/响应操作需要重新建立连接。
长连接:即持久连接,指客户端和服务器建立一次连接之后可以在这条连接上进行多次请求/响应操作。长连接可以设置过期时间,也可以不设置。
一、简单输出文本信息,如“你好,秋天!”
myhttp.c
1 #include <string.h>
2 #include <stdio.h>
3 #include <stdlib.h>
4 #include <unistd.h>
5 #include <assert.h>
6 #include <sys/socket.h>
7 #include <netinet/in.h>
8 #include <arpa/inet.h>
9
10 //服务器与客户端HTTP
11 //简单输出你好,秋天!
12 int main()
13 {
14 int sockfd = socket(AF_INET, SOCK_STREAM, 0);//套接字
15 assert(sockfd != -1);
16
17 struct sockaddr_in saddr, caddr;
18 memset(&saddr,0, sizeof(saddr));
19 saddr.sin_family = AF_INET;
20 saddr.sin_port = htons(80);
21 saddr.sin_addr.s_addr = inet_addr("127.0.0.1");
22
23 int res = bind(sockfd, (struct sockaddr*)&saddr, sizeof(saddr));
24 assert(res != -1);
25
26 listen(sockfd,5);
27
28 while(1)
29 {
30 int len = sizeof(caddr);
31 int c = accept(sockfd,(struct sockaddr*)&caddr,&len);
32 if(c < 0)
33 {
34 continue;
35 }
36
37 char buff[1024] = {0};
38 recv(c,buff,1000,0);
39 printf("recv:\n%s\n",buff);
40
41 char *s ="你好,秋天!";
42 send(c,s,strlen(s),0);
43
44 close(c);
45 }
46 }
需要管理员权限才能运行。
切换管理员的方法:(1)命令行输入su;(2)输入密码,即切换成功。
退出管理员的方法:命令行输入exit。
运行代码,在浏览器输入127.0.0.1,即可显示“你好,秋天!”。
若出现乱码,可通过改变编码为Unicode(UTF-8)模式。
扫描二维码关注公众号,回复:
3648594 查看本文章
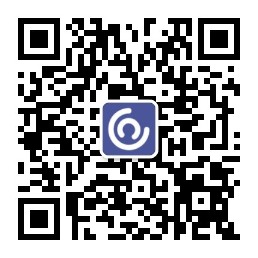
二、HTTP应答模式
myhttp2.c
1 #include <string.h>
2 #include <stdio.h>
3 #include <stdlib.h>
4 #include <unistd.h>
5 #include <assert.h>
6 #include <sys/socket.h>
7 #include <netinet/in.h>
8 #include <arpa/inet.h>
9
10 //服务器与客户端HTTP
11 //HTTP应答部分
12 int main()
13 {
14 int sockfd = socket(AF_INET, SOCK_STREAM, 0);//套接字
15 assert(sockfd != -1);
16
17 struct sockaddr_in saddr, caddr;
18 memset(&saddr,0, sizeof(saddr));
19 saddr.sin_family = AF_INET;
20 saddr.sin_port = htons(80);
21 saddr.sin_addr.s_addr = inet_addr("127.0.0.1");
22
23 int res = bind(sockfd, (struct sockaddr*)&saddr, sizeof(saddr));
24 assert(res != -1);
25
26 listen(sockfd,5);
27
28 while(1)
29 {
30 int len = sizeof(caddr);
31 int c = accept(sockfd,(struct sockaddr*)&caddr,&len);
32 if(c < 0)
33 {
34 continue;
35 }
36
37 char buff[1024] = {0};
38 recv(c,buff,1000,0);
39 printf("recv:\n%s\n",buff);
40
41 char *s ="你好,秋天!";
42 char sendbuff[256] = {0};
43 strcpy(sendbuff,"http/1.1 200 OK\r\n");
44 strcat(sendbuff,"Server:myhttp\r\n");
45 strcat(sendbuff,"Content-Length:5\r\n");
46 strcat(sendbuff,"\r\n");
47 strcat(sendbuff,"hello");
48
49 printf("send:\n%s\n",sendbuff);
50 send(c,sendbuff,strlen(sendbuff),0);
51 close(c);
52 }
53 }
结果:
三、建立文件index.html,进而访问文件内容
myhttp3.c
1 #include <string.h>
2 #include <stdio.h>
3 #include <stdlib.h>
4 #include <unistd.h>
5 #include <assert.h>
6 #include <sys/socket.h>
7 #include <netinet/in.h>
8 #include <arpa/inet.h>
9 #include <fcntl.h>
10
11 //服务器与客户端HTTP
12 //读文件index.html里的内容
13 int main()
14 {
15 int sockfd = socket(AF_INET, SOCK_STREAM, 0);//套接字
16 assert(sockfd != -1);
17
18 struct sockaddr_in saddr, caddr;
19 memset(&saddr,0, sizeof(saddr));
20 saddr.sin_family = AF_INET;
21 saddr.sin_port = htons(80);
22 saddr.sin_addr.s_addr = inet_addr("127.0.0.1");
23
24 int res = bind(sockfd, (struct sockaddr*)&saddr, sizeof(saddr));
25 assert(res != -1);
26
27 listen(sockfd,5);
28
29 while(1)
30 {
31 int len = sizeof(caddr);
32 int c = accept(sockfd,(struct sockaddr*)&caddr,&len);
33 if(c < 0)
34 {
35 continue;
36 }
37
38 char buff[1024] = {0};
39 recv(c,buff,1000,0);
40 printf("recv:\n%s\n",buff);
41
42 int fd = open("index.html",O_RDONLY);
43 if(fd == -1)
44 {
45 close(c);
46 continue;
47 }
48
49 int size = lseek(fd,0,SEEK_END);
50 lseek(fd,0,SEEK_SET);
51 char sendbuff[256] = {0};
52 strcpy(sendbuff,"http/1.1 200 OK\r\n");
53 strcat(sendbuff,"Server:myhttp\r\n");
54 sprintf(sendbuff + strlen(sendbuff),"Content-Length:%d\r\n",size);
55 strcat(sendbuff,"\r\n");
56
57 printf("send:\n%s\n",sendbuff);
58 send(c,sendbuff,strlen(sendbuff),0);
59
60 char readbuff[512] = {0};
61 int n = 0;
62 while((n = read(fd,readbuff,512)) > 0)
63 {
64 send(c,readbuff,n,0);
65 }
66
67 close(fd);
68 close(c);
69 }
70 }
index.html
<html>
<head>
<title>主页</title>
</head>
<body>
<center>
床前明月光,</br>
疑是地上霜。</br>
举头望明月,</br>
低头思故乡。</br>
</center>
</body>
</html>
结果:
前提:需要在与myhttp3.c同目录下建立一个文件名为index.html文件,里面写上HTML语言的代码,进而运行查看结果。