tensorflow中:
constant tensor不能直接赋值,否则会报错:
TypeError:
'Tensor'
object
does
not
support item assignment
Variable
tensor不能为某个特定元素赋值,只能为整个变量tensor全部赋值。
赋值命令为:new_state = tf.assign(state,new_tensor)
为了解决张量元素赋值问题,上网查看解决方法,主要是针对一维张量的,主要是用one_hot来实现,如文章:张量元素值修改,
本文参照其思想,实现2D张量元素替换,如果想实现更高维度的张量的元素赋值,可以在此基础上再进行修改。
脚本如下,亲测可行,如有纰漏,欢迎大家拍砖指教。
#-*-coding:utf-8-*-
import tensorflow as tf
sess = tf.InteractiveSession()
sess.run(tf.global_variables_initializer())
def tensor_expand(tensor_Input,Num):
'''
张量自我复制扩展,将Num个tensor_Input串联起来,生成新的张量,
新的张量的shape=[tensor_Input.shape,Num]
:param tensor_Input:
:param Num:
:return:
'''
tensor_Input = tf.expand_dims(tensor_Input,axis=0)
tensor_Output = tensor_Input
for i in range(Num-1):
tensor_Output= tf.concat([tensor_Output,tensor_Input],axis=0)
return tensor_Output
def get_one_hot_matrix(height,width,position):
'''
生成一个 one_hot矩阵,shape=【height*width】,在position处的元素为1,其余元素为0
:param height:
:param width:
:param position: 格式为【h_Index,w_Index】,h_Index,w_Index为int格式
:return:
'''
col_length = height
row_length = width
col_one_position = position[0]
row_one_position = position[1]
rows_num = height
cols_num = width
single_row_one_hot = tf.one_hot(row_one_position, row_length, dtype=tf.float32)
single_col_one_hot = tf.one_hot(col_one_position, col_length, dtype=tf.float32)
one_hot_rows = tensor_expand(single_row_one_hot, rows_num)
one_hot_cols = tensor_expand(single_col_one_hot, cols_num)
one_hot_cols = tf.transpose(one_hot_cols)
one_hot_matrx = one_hot_rows * one_hot_cols
return one_hot_matrx
def tensor_assign_2D(tensor_input,position,value):
'''
给 2D tensor的特定位置元素赋值
:param tensor_input: 输入的2D tensor,目前只支持2D
:param position: 被赋值的张量元素的坐标位置,=【h_index,w_index】
:param value:
:return:
'''
shape = tensor_input.get_shape().as_list()
height = shape[0]
width = shape[1]
h_index = position[0]
w_index = position[1]
one_hot_matrix = get_one_hot_matrix(height, width, position)
new_tensor = tensor_input - tensor_input[h_index,w_index]*one_hot_matrix +one_hot_matrix*value
return new_tensor
if __name__=="__main__":
##test
tensor_input = tf.constant([i for i in range(20)],tf.float32)
tensor_input = tf.reshape(tensor_input,[4,5])
new_tensor = tensor_assign_2D(tensor_input,[2,3],100)
print(new_tensor.eval())
本文原创,如需转载,请注明出处:https://blog.csdn.net/Strive_For_Future/article/details/82426015
扫描二维码关注公众号,回复:
3618397 查看本文章
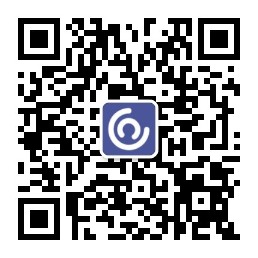