版权声明:《==study hard and make progress every day==》 https://blog.csdn.net/qq_38225558/article/details/83053508
文件的上传
servlet3.0本身具有文件上传功能
这里我们使用第三方开源 fileupload 实现文件的上传
后台实现上传功能类:(当文件上传的时候,后台需要去解析请求对象【数据仍然在请求对象中】)
/**
* fileupload实现文件上传功能步骤:
* ①导入jar包,创建上传页面 commons-fileupload-1.2.2.jar,commons-io-1.4.jar
* ②解析请求类型,获取请求中的文件项
* ③遍历文件项,判断普通项和文件项
* ④获取上传的文件名称
* ⑤存储数据:将文件写入到服务器本地
* @author 郑清
*/
@WebServlet("/upload")
public class FileUploadServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
try {
FileItemFactory factory = new DiskFileItemFactory();//创建文件磁盘工厂对象
ServletFileUpload upload = new ServletFileUpload(factory);//根据文件磁盘工厂对象创建文件解析器对象
List<FileItem> items = upload.parseRequest(req);//解析请求类型,获得文件项,进行文件上传时,分为普通项和文件项 List<FileItem>:前端页面提交的数据被封装到FileItem
// 遍历 ==> 判断数据类型
for (FileItem fileItem : items) {
if (fileItem.isFormField()) { //普通项 isFormFiled:true->普通数据,false->文件数据
System.out.println(fileItem.getString("UTF-8"));//获取普通项
} else { //文件项
ServletContext context = getServletContext();
String name = fileItem.getName();//获取文件上传的名称
//判读上传的文件是否是一个图片
String mimeType = context.getMimeType(name);
if (mimeType==null||!mimeType.startsWith("image/")) {
System.out.println("===不属于图片类型...===");
return;
}
String extension = FilenameUtils.getExtension(name);//获取文件后缀名
String newName = UUID.randomUUID().toString() + "." + extension;//防止上传的文件同名
//servlet中 获取到本地绝对路径
String realPath = context.getRealPath("/upload");
File f = new File(realPath, newName);
fileItem.write(f);//将文件写入本地
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
前端上传页面:
注意: ①需要使用post提交
②form表单添加属性:enctype="multipart/form-data" (form表单默认不会将文件的数据上传,只会上传普通的数据设置了该属性之后,浏览器会将文件数据提交给服务器)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>首页</title>
</head>
<body>
<h1>文件上传</h1>
<form action="/zq/upload" method="post" enctype="multipart/form-data" >
用户名:<input type="text" name="username" ><br/>
上传头像:<input type="file" name="imgupload" ><br/>
<input type="submit" >
</form>
</body>
</html>
文件的下载
超链接下载
注意:如果是浏览器支持的文件类型,浏览器会直接打开文件,注意:需要准备一个文件夹存放下载文件
也就是说如果超链接指向的资源【文档、图片、视频】,浏览器支持的文件类型默认可以打开,那么浏览器会选择直接打开 ,当浏览器打不开资源时,就执行下载
<a href="${pageContext.request.contextPath }/download/1.txt" >下载文本文档</a><br/>
<a href="${pageContext.request.contextPath }/download/1.jpg" >下载图片</a><br/>
程序下载
注意:前台还是使用超链接,超链接转到后台servlet,在servlet通过流的方式把文件复制到前台
<a href="${pageContext.request.contextPath }/download?filename=1.txt" >下载文本文档</a><br/>
<a href="${pageContext.request.contextPath }/download?filename=1.jpg" >下载图片</a><br/>
后台实现下载功能类:
扫描二维码关注公众号,回复:
3610037 查看本文章
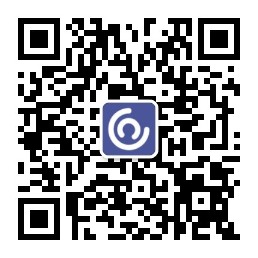
/**
* 文件下载:
* ①获取前台要下载的文件名称
* ②设置响应类型
* ③设置下载页显示的文件名
* ④获取下载文件夹的绝对路径和文件名合并为File类型
* ⑤将文件复制到浏览器
* @author 郑清
*/
@WebServlet("/download")
public class DownLoadServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws IOException {
String realPath = getServletContext().getRealPath("/download");//获取下载文件路径
String filename = req.getParameter("filename");//获取前台传过来的名称
File file = new File(realPath,filename);//把下载文件构成一个文件处理
//设置响应类型 ==》 告诉浏览器当前是下载操作,我要下载东西
resp.setContentType("application/x-msdownload");
//设置下载时文件的显示类型(即文件名称-后缀) ex:txt为文本类型
resp.setHeader("Content-Disposition","attachment;filename="+filename);
//下载文件:将一个路径下的文件数据转到一个输出流中,也就是把服务器文件通过流写(复制)到浏览器端
Files.copy(file.toPath(), resp.getOutputStream());//Files.copy(要下载的文件路径,响应的输出流)
}
}
点击下载项目资源 密码:8wf2