一、认识
FastDFS是一个开源的轻量级分布式文件系统,它对文件进行管理,功能包括:文件存储、文件同步、文件访问(文件上传、文件下载)等,解决了大容量存储和负载均衡的问题。特别适合以文件为载体的在线服务,如相册网站、视频网站等等。
FastDFS为互联网量身定制,充分考虑了冗余备份、负载均衡、线性扩容等机制,并注重高可用、高性能等指标,使用FastDFS很容易搭建一套高性能的文件服务器集群提供文件上传、下载等服务。
二、不建议搭
建议直接拿搭好的,搭一次至少一两天
三、不搭就至少得懂下原理
上传文件流程:
下载文件流程:
四、fastDFS在项目中的使用方法
(1)开启FastDFS服务器,我的ip地址为192.168.25.133
(2)导入包
<dependency>
<groupId>fastdfs_client</groupId>
<artifactId>fastdfs_client</artifactId>
<version>1.25</version>
</dependency>
(3)新建一个test文件测试一下:
在resources/resource/下面创建一个配置文件:client.conf
里面写入:22122默认的
tracker_server=192.168.25.133:22122
测试一下:上传一个文件到服务器里面:
public class TestFastDFS {
@Test
public void uploadFile() throws Exception {
//1、向工程中添加jar包 fastdfs_client-1.25.jar
//2、创建一个配置文件。配置tracker服务器地址
//3、加载配置文件
ClientGlobal.init("C:/Users/qinhua/Desktop/shop/taotao-manager-web/src/main/resources/resource/client.conf");
//4、创建一个TrackerClient对象。
TrackerClient trackerClient = new TrackerClient();
//5、使用TrackerClient对象获得trackerserver对象。
TrackerServer trackerServer = trackerClient.getConnection();
//6、创建一个StorageServer的引用null就可以。
StorageServer storageServer = null;
//7、创建一个StorageClient对象。trackerserver、 StorageServer两个参数。
StorageClient storageClient = new StorageClient(trackerServer, storageServer);
//8、使用StorageClient对象上传文件。
String[] strings = storageClient.upload_file("D:/1.jpg", "jpg", null);
for (String string : strings) {
System.out.println(string);
}
}
}
test后的结果为
(3)我们去服务器看看有没有:
访问网站:192.168.25.133/group1/M00/00/00/wKgZhVu-77qAcQKYAADW-NO57MA572.jpg
五、优化一下
把上面代码封装到util中,你们直接复制过去就可以了
package com.utils;
import org.csource.common.NameValuePair;
import org.csource.fastdfs.ClientGlobal;
import org.csource.fastdfs.StorageClient1;
import org.csource.fastdfs.StorageServer;
import org.csource.fastdfs.TrackerClient;
import org.csource.fastdfs.TrackerServer;
public class FastDFSClient {
private TrackerClient trackerClient = null;
private TrackerServer trackerServer = null;
private StorageServer storageServer = null;
private StorageClient1 storageClient = null;
public FastDFSClient(String conf) throws Exception {
if (conf.contains("classpath:")) {
conf = conf.replace("classpath:", this.getClass().getResource("/").getPath());
}
ClientGlobal.init(conf);
trackerClient = new TrackerClient();
trackerServer = trackerClient.getConnection();
storageServer = null;
storageClient = new StorageClient1(trackerServer, storageServer);
}
/**
* 上传文件方法
* <p>Title: uploadFile</p>
* <p>Description: </p>
* @param fileName 文件全路径
* @param extName 文件扩展名,不包含(.)
* @param metas 文件扩展信息
* @return
* @throws Exception
*/
public String uploadFile(String fileName, String extName, NameValuePair[] metas) throws Exception {
String result = storageClient.upload_file1(fileName, extName, metas);
return result;
}
public String uploadFile(String fileName) throws Exception {
return uploadFile(fileName, null, null);
}
public String uploadFile(String fileName, String extName) throws Exception {
return uploadFile(fileName, extName, null);
}
/**
* 上传文件方法
* <p>Title: uploadFile</p>
* <p>Description: </p>
* @param fileContent 文件的内容,字节数组
* @param extName 文件扩展名
* @param metas 文件扩展信息
* @return
* @throws Exception
*/
public String uploadFile(byte[] fileContent, String extName, NameValuePair[] metas) throws Exception {
String result = storageClient.upload_file1(fileContent, extName, metas);
return result;
}
public String uploadFile(byte[] fileContent) throws Exception {
return uploadFile(fileContent, null, null);
}
public String uploadFile(byte[] fileContent, String extName) throws Exception {
return uploadFile(fileContent, extName, null);
}
}
再测试一下:
@Test
public void testFastDfsClient() throws Exception {
FastDFSClient fastDFSClient = new FastDFSClient("C:/Users/qinhua/Desktop/shop/taotao-manager-web/src/main/resources/resource/client.conf");
String string = fastDFSClient.uploadFile("D:/2.jpg");
System.out.println(string);
}
六、把功能(上传图片到服务器)写到controller中
(1)由于我这里用的是kindeditor来上传图片,所以首先要导入图片上传的相关包:
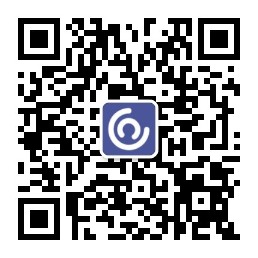
<!-- 文件上传组件 -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
</dependency>
(2)在springmvc.xml配置文件里面加上
<!-- 多媒体解析器 -->
<!-- 配置文件上传解析器 -->
<bean id="multipartResolver"
class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<!-- 设定默认编码 -->
<property name="defaultEncoding" value="UTF-8"></property>
<!-- 设定文件上传的最大值5MB,5*1024*1024 -->
<property name="maxUploadSize" value="5242880"></property>
</bean>
(3)由于我们是把图片上传到FastDFS服务器的,所以建立一个配置文件resource.properties,里面写上服务器地址:
IMAGE_SERVER_URL=http://192.168.25.133/
(4)开始编写controller
注意:由于我这里面用的是kindEditor来上传图片,所以返回的数据是固定格式的,如下图,我用map把后台返回给前端的数据封装起来,然后为浏览器兼容性,又把map转换为string:
http://kindeditor.net/docs/upload.html
controller类如下:
@Controller
public class PictureController {
@Value("${IMAGE_SERVER_URL}")
private String IMAGE_SERVER_URL;
@RequestMapping("/pic/upload")
@ResponseBody
public String picUpload(MultipartFile uploadFile) {
try {
//接收上传的文件
//取扩展名
String originalFilename = uploadFile.getOriginalFilename();
String extName = originalFilename.substring(originalFilename.lastIndexOf(".") + 1);
//上传到图片服务器
FastDFSClient fastDFSClient = new FastDFSClient("classpath:resource/client.conf");
String url = fastDFSClient.uploadFile(uploadFile.getBytes(), extName);
url = IMAGE_SERVER_URL + url;
//响应上传图片的url
Map result = new HashMap<>();
result.put("error", 0);
result.put("url", url);
return JsonUtils.objectToJson(result);
} catch (Exception e) {
e.printStackTrace();
Map result = new HashMap<>();
result.put("error", 1);
result.put("message", "图片上传失败哈");
return JsonUtils.objectToJson(result);
}
}
}
我这里的JsonUtils是一个封装好的数据转换类,你们直接拿就可以了:
import java.util.List;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonUtils {
// 定义jackson对象
private static final ObjectMapper MAPPER = new ObjectMapper();
/**
* 将对象转换成json字符串。
* <p>Title: pojoToJson</p>
* <p>Description: </p>
* @param data
* @return
*/
public static String objectToJson(Object data) {
try {
String string = MAPPER.writeValueAsString(data);
return string;
} catch (JsonProcessingException e) {
e.printStackTrace();
}
return null;
}
/**
* 将json结果集转化为对象
*
*/
public static <T> T jsonToPojo(String jsonData, Class<T> beanType) {
try {
T t = MAPPER.readValue(jsonData, beanType);
return t;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
/**
* 将json数据转换成pojo对象list
* <p>Title: jsonToList</p>
* <p>Description: </p>
* @param jsonData
* @param beanType
* @return
*/
public static <T>List<T> jsonToList(String jsonData, Class<T> beanType) {
JavaType javaType = MAPPER.getTypeFactory().constructParametricType(List.class, beanType);
try {
List<T> list = MAPPER.readValue(jsonData, javaType);
return list;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}