Given an integer, write an algorithm to convert it to hexadecimal. For negative integer, two’s complementmethod is used.
Note:
- All letters in hexadecimal (
a-f
) must be in lowercase. - The hexadecimal string must not contain extra leading
0
s. If the number is zero, it is represented by a single zero character'0'
; otherwise, the first character in the hexadecimal string will not be the zero character. - The given number is guaranteed to fit within the range of a 32-bit signed integer.
- You must not use any method provided by the library which converts/formats the number to hex directly.
Example 1:
Input: 26 Output: "1a"
Example 2:
Input: -1 Output: "ffffffff"
给定一个整数,编写一个算法将这个数转换为十六进制数。对于负整数,我们通常使用 补码运算 方法。
注意:
- 十六进制中所有字母(
a-f
)都必须是小写。 - 十六进制字符串中不能包含多余的前导零。如果要转化的数为0,那么以单个字符
'0'
来表示;对于其他情况,十六进制字符串中的第一个字符将不会是0字符。 - 给定的数确保在32位有符号整数范围内。
- 不能使用任何由库提供的将数字直接转换或格式化为十六进制的方法。
示例 1:
输入: 26 输出: "1a"
示例 2:
输入: -1 输出: "ffffffff"
8ms
1 class Solution { 2 var hex:String = "0123456789abcdef" 3 func toHex(_ num: Int) -> String { 4 if num == 0 {return "0"} 5 var number:Int = num 6 var result:String = "" 7 var count:Int = 0 8 while(number != 0 && count < 8) 9 { 10 var index = hex.index(hex.startIndex,offsetBy: (number & 0xf)) 11 result.insert(hex[index],at: result.startIndex) 12 //注意符号:>>= 13 number >>= 4 14 count += 1 15 } 16 return result 17 } 18 }
8ms
1 class Solution { 2 func toHex(_ num: Int) -> String { 3 var result = "" 4 var map = [10:"a", 11:"b", 12:"c", 13:"d", 14:"e", 15:"f"] 5 6 let base = 16 7 var tmp = num 8 9 if tmp < 0 { 10 tmp += 2 << 31 11 } else if tmp == 0 { 12 return "0" 13 } 14 15 while tmp != 0 { 16 let mod = tmp % base 17 tmp = tmp / base 18 19 if mod < 10 { 20 result = String(mod) + result 21 } else { 22 if let value = map[mod] { 23 result = value + result 24 } 25 } 26 } 27 28 return result 29 } 30 }
12ms
1 class Solution { 2 func toHex(_ num: Int) -> String { 3 guard num != 0 else { 4 return "0" 5 } 6 7 let map = ["0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d", "e", "f"] 8 var result = "" 9 var num = num < 0 ? Int(UInt32.max) + num + 1 : num 10 while num > 0 { 11 result = map[num % 16] + result 12 num /= 16 13 } 14 15 return result 16 } 17 }
12ms
扫描二维码关注公众号,回复:
3560277 查看本文章
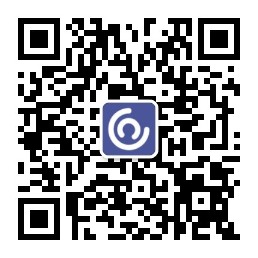
1 class Solution { 2 func toHex(_ num: Int) -> String { 3 let map = ["0","1","2","3","4","5","6","7","8","9","a","b","c","d","e","f"] 4 if num > 0 { 5 var temp = num 6 var res = "" 7 while temp != 0 { 8 let v = temp & 15 9 res = map[v] + res 10 temp >>= 4 11 } 12 return res 13 } else if num == 0 { 14 return "0" 15 } else { 16 var res = "" 17 for i in 0..<8 { 18 let v = num & (15 << (i*4)) 19 res = map[v>>(i*4)] + res 20 } 21 return res 22 } 23 } 24 }
16ms
1 class Solution { 2 func toHex(_ num: Int) -> String { 3 if num == 0 { 4 return "0" 5 } 6 7 let map = ["0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d", "e", "f"] 8 var num = num 9 var res = "" 10 var i = 0 11 12 while num != 0 && i < 8 { 13 res = map[num & 0b1111] + res 14 num >>= 4 15 i += 1 16 } 17 18 return res 19 } 20 }