public void updateApp() {
//as引用库 com.qianwen:okhttp-utils:3.8.0
OkHttpUtils.get()
// 服务器json地址(json文件或者接口)
.url(StringUtil.UPDATE_URL)
.build()
.execute(new StringCallback() {
@Override
public void onError(Call call, Response response, Exception e, int id) {
}
@Override
public void onResponse(String response, int id) {
//本地APP versionCode
int versionCode = BuildConfig.VERSION_CODE;
try {
JSONObject jsonObject = new JSONObject(response);
// 服务器端APP versionCode
int vesion = Integer.valueOf(jsonObject.optString("new_version").trim());
//app大小
String size = jsonObject.optString("target_size");
//更新日志
String log = jsonObject.optString("update_log");
//本地版本小于服务器版本显示更新对话框
if (versionCode < vesion) {
showDiyDialog(size, log);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
private void showDiyDialog(String targetSize, String updateLog) {
String msg = "";
if (!TextUtils.isEmpty(targetSize)) {
msg = "新版本大小:" + targetSize + "\n\n";
}
if (!TextUtils.isEmpty(updateLog)) {
msg += updateLog;
}
new AlertDialog.Builder(this)
.setTitle(String.format("是否升级到最新版本?"))
.setMessage(msg)
.setPositiveButton("升级", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
downloadApk();
dialog.dismiss();
}
})
.setNegativeButton("暂不升级", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
}
})
.create()
.show();
}
private void downloadApk() {
//显示下载进度
ProgressDialog dialog = new ProgressDialog(this);
dialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
dialog.setCancelable(false);
dialog.show();
new Thread(new DownloadApk(dialog)).start();
}
private class DownloadApk implements Runnable {
private ProgressDialog dialog;
InputStream is;
FileOutputStream fos;
public DownloadApk(ProgressDialog dialog) {
this.dialog = dialog;
}
@Override
public void run() {
OkHttpClient client = new OkHttpClient();
//下载地址
String url = StringUtil.APPDOWNLOAD_URL;
Request request = new Request.Builder().get().url(url).build();
try {
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
//获取内容总长度
long contentLength = response.body().contentLength();
//设置最大值
dialog.setMax((int) contentLength);
//保存到sd卡
File apkFile = new File("mnt/sdcard", "HomeTheaters.apk");
fos = new FileOutputStream(apkFile);
//获得输入流
is = response.body().byteStream();
//定义缓冲区大小
byte[] bys = new byte[1024];
int progress = 0;
int len = -1;
while ((len = is.read(bys)) != -1) {
try {
Thread.sleep(1);
fos.write(bys, 0, len);
fos.flush();
progress += len;
//设置进度
dialog.setProgress(progress);
} catch (InterruptedException e) {
}
}
//下载完成,提示用户安装
installApk(apkFile);
}
} catch (IOException e) {
} finally {
//关闭io流
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
is = null;
}
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
fos = null;
}
}
dialog.dismiss();
}
}
private void installApk(File file) {
//调用系统安装程序
Intent intent = new Intent();
intent.setAction("android.intent.action.VIEW");
intent.addCategory("android.intent.category.DEFAULT");
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.setDataAndType(Uri.fromFile(file), "application/vnd.android.package-archive");
startActivity(intent);
}
服务器端json格式
{
"code": 0,
"data": {
"download_url": "app下载地址",
"force": false,
"update_content": "软件更新",
"v_code": "1",
"v_name": "1.0",
"v_sha1": "7db76e18ac92bb29ff0ef012abfe178a78477534",
"v_size": 12365909
}
}
实现效果
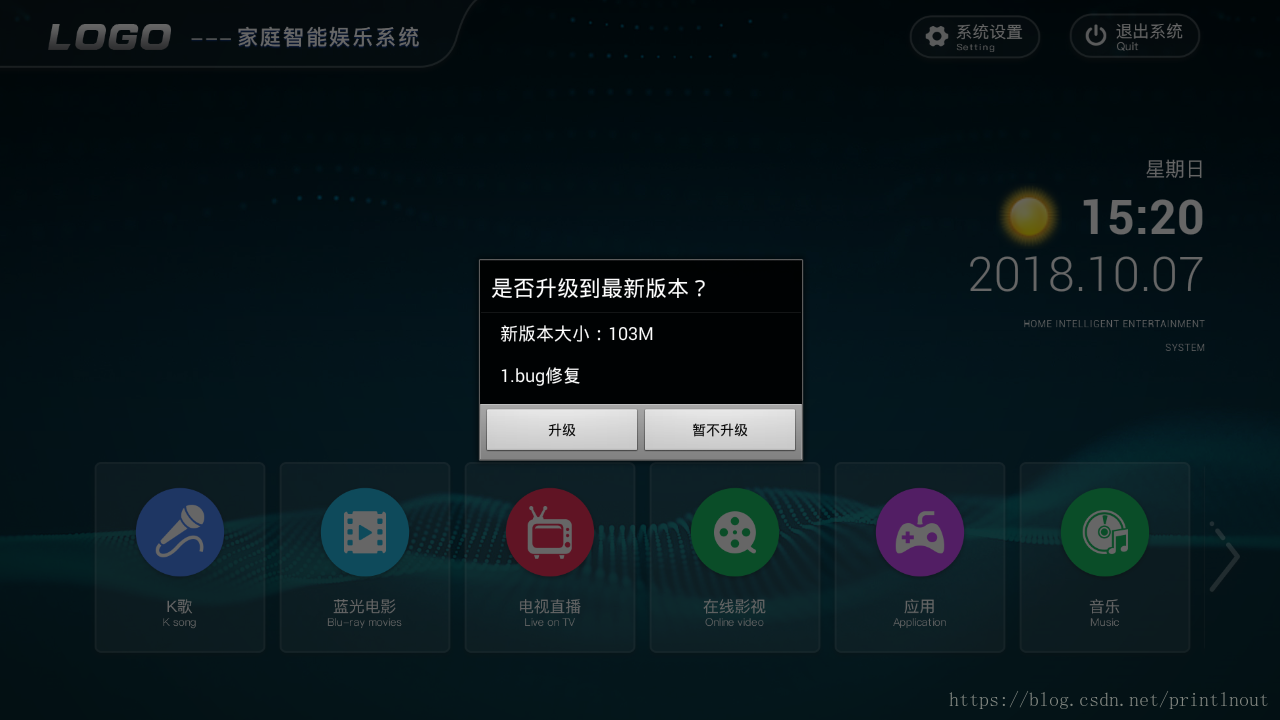
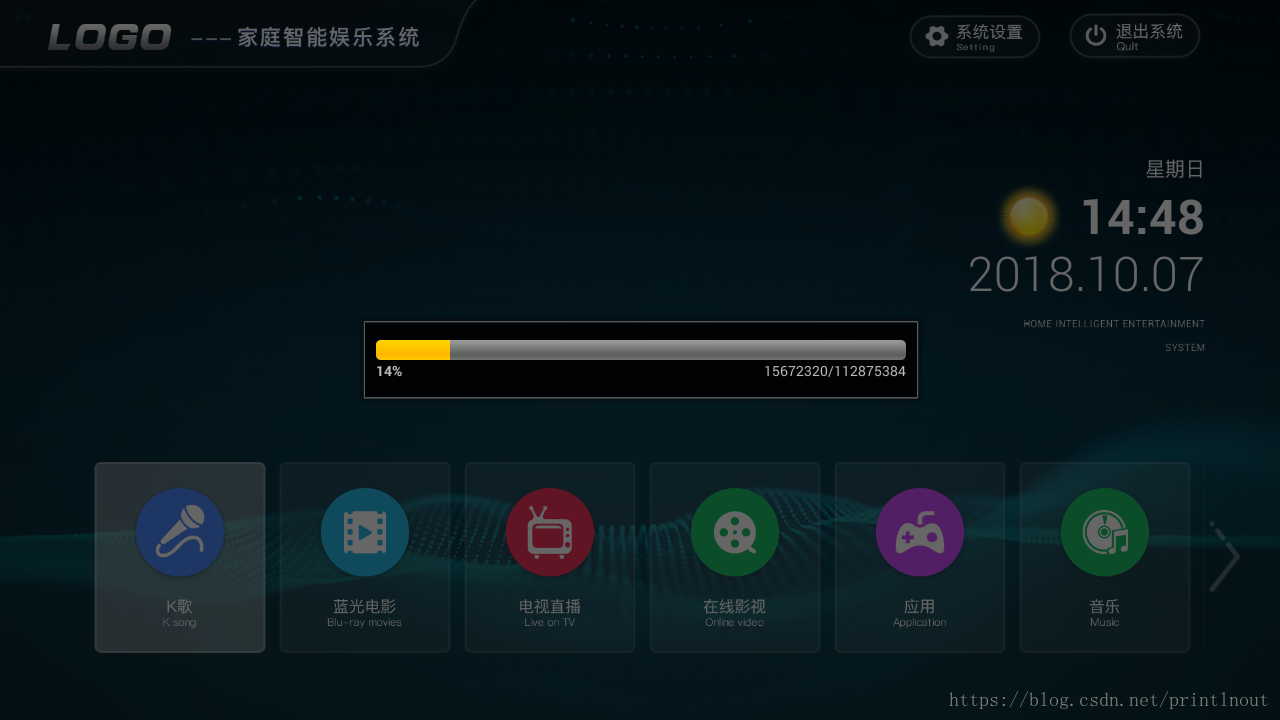