MIPS汇编语言实现选择排序算法
1.流程图
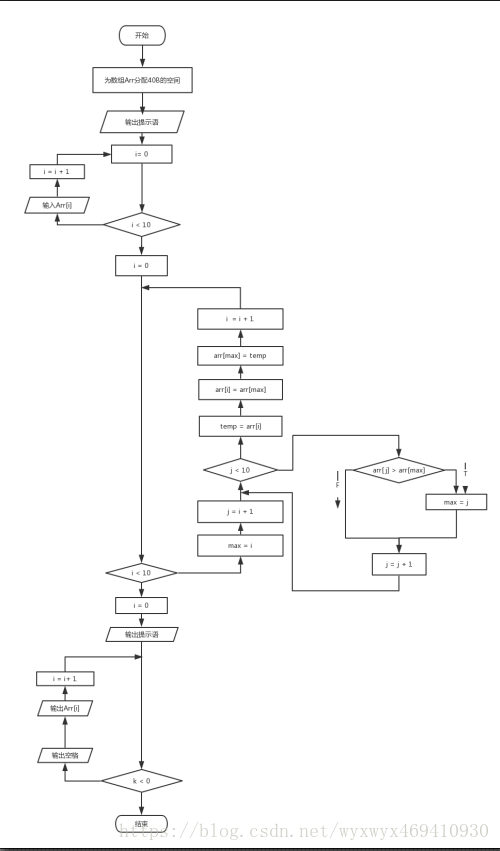
2.C代码
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
int main()
{
uint32_t num = 10 ;
uint32_t *arr = malloc(sizeof(uint32_t)*num);
printf("Please input:");
printf("%u",num);
printf(" unsigned integer, with one integer in one line:\n");
for(uint32_t i = 0 ; i < num ; i ++ )
{
scanf("%u",&arr[i] );
}
for(uint32_t i = 0 ; i < num ; i ++ )
{
uint32_t max = i ;
for( uint32_t j = i + 1 ; j < num ; j ++ )
{
if(arr[j] > arr[max])
{
max = j ;
}
}
uint32_t temp = arr[i] ;
arr[i] = arr[max];
arr[max] = temp ;
}
printf("Result: ");
for(uint32_t i = 0 ; i < num ; i ++ )
{
printf(" ");
printf("%u",arr[i]);
}
printf("\n");
free(arr);
}
3.MIPS代码(附注释)
.text
.globl main
main:
la $a0, prompt1
li $v0, 4
syscall
la $t0, num
lw $t0, ($t0)
add $a0, $t0, $0
li $v0, 1
syscall
la $a0, prompt2
li $v0, 4
syscall
sll $a0, $t0, 2
li $v0, 9
syscall
add $t1, $v0, $0
add $t2, $0, $0
inputLoop:
slt $t3, $t2, $t0
beq $t3, $0, inputLoopBreak
li $v0, 5
syscall
sll $t3, $t2, 2
add $t3, $t1, $t3
sw $v0, ($t3)
j inputLoopCont
inputLoopCont:
addi $t2, $t2, 1
j inputLoop
inputLoopBreak:
add $t2, $0, $0
outerLoop:
slt $t3, $t2, $t0
beq $t3, $0, outerLoopBreak
add $t4, $t2, $0
addi $t5, $t2, 1
innerLoop:
slt $t3, $t5, $t0
beq $t3, $0, innerLoopBreak
sll $t3, $t5, 2
add $t3, $t1, $t3
lw $t3, ($t3)
sll $t6, $t4, 2
add $t6, $t1, $t6
lw $t6, ($t6)
slt $t3, $t3, $t6
bne $t3, $0, afterReplace
replace:
add $t4, $t5, $0
j afterReplace
afterReplace:
j innerLoopCont
innerLoopCont:
addi $t5, $t5, 1
j innerLoop
innerLoopBreak:
beq $t2, $t4, afterSwap
swap:
sll $t3, $t2, 2
add $t3, $t1, $t3
sll $t5, $t4, 2
add $t5, $t1, $t5
lw $t6, ($t3)
lw $t7, ($t5)
sw $t7, ($t3)
sw $t6, ($t5)
j afterSwap
afterSwap:
j outerLoopCont
outerLoopCont:
addi $t2, $t2, 1
j outerLoop
outerLoopBreak:
la $a0, result
li $v0, 4
syscall
add $t2, $0, $0
outputLoop:
slt $t3, $t2, $t0
beq $t3, $0, outputLoopBreak
la $a0, space
li $v0, 4
syscall
sll $t3, $t2, 2
add $t3, $t1, $t3
lw $a0, ($t3)
li $v0, 1
syscall
j outputLoopCont
outputLoopCont:
addi $t2, $t2, 1
j outputLoop
outputLoopBreak:
la $a0, newline
li $v0, 4
syscall
li $v0, 10
syscall
.data
prompt1:
.asciiz "Please input "
prompt2:
.asciiz " unsigned integers, with one integer in one line:\n"
result:
.asciiz "Result:"
space:
.asciiz " "
newline:
.asciiz "\n"
num:
.word 0x0000000a