版权声明:本文为博主原创文章,未经博主允许不得转载,博客地址:http://blog.csdn.net/mars_xiaolei。 https://blog.csdn.net/mars_xiaolei/article/details/82877160
C++程序在存储器的全局存储区(堆-Heap)分配和释放动态存储块,new负责从堆中分配内存,delete负责把内存还给堆(释放内存)。
new和delete的简单使用
new可以跟数据类型、类、结构体、数组的名字一起使用,new分配内存之后,必须要使用delete释放内存,否则的话,可能会导致内存泄漏(memory leak),小程序还好,大程序长时间运行就会停止运行。
#include "iostream"
using namespace std;
struct Date
{
int month;
int day;
int year;
};
void main()
{
Date* birthday=new Date;
birthday->month=6;
birthday->day=24;
birthday->year=2012;
cout<<"This day is:"<<birthday->month<<"/"<<birthday->day<<"/"<<birthday->year<<endl;
delete birthday;
system("pause");
}
输出结果:
This day is:6/24/2012
请按任意键继续. . .
一般使用流程:
1、使用new运算符分配内存
2、对变量或者对象进行初始化操作
3、执行其它操作
4、在程序结束之前使用delete释放内存(如果return,则放在return之前)
new和delete固定维数数组
使用new为数组分配内存和前面差不多,但是使用delete为数组释放内存就有点不一样了,delete之后添加了“ []”,意思是将要删除的内存空间是数组。
#include "iostream"
using namespace std;
int main()
{
int *birthday=new int[3];
birthday[0]=2018;
birthday[1]=9;
birthday[2]=28;
cout<<"Today is:"<<birthday[0]<<"/"<<birthday[1]<<"/"<<birthday[2]<<endl;
delete [] birthday;
system("pause");
return 0;
}
输出结果:
Today is:2018/9/28
请按任意键继续. . .
new和delete动态内存数组
数组的大小不确定,由屏幕输入时,new将根据输入的变量值精确分配内存。
扫描二维码关注公众号,回复:
3406964 查看本文章
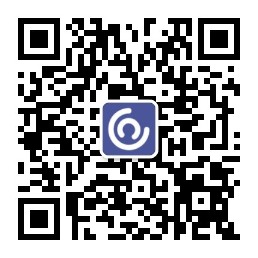
#include "iostream"
#include "cstdlib"
using namespace std;
void main()
{
int size;
cout<<"Enter the array size:";
cin>>size;
int* array=new int[size];
for(int i=0;i<size;i++)
{
array[i]=rand();
}
for(int i=0;i<size;i++)
{
cout<<"\n"<<array[i];
}
cout<<std::endl;
delete [] array;
system("pause");
return;
}
输出结果:
Enter the array size:6
41
18467
6334
26500
19169
15724
请按任意键继续. . .