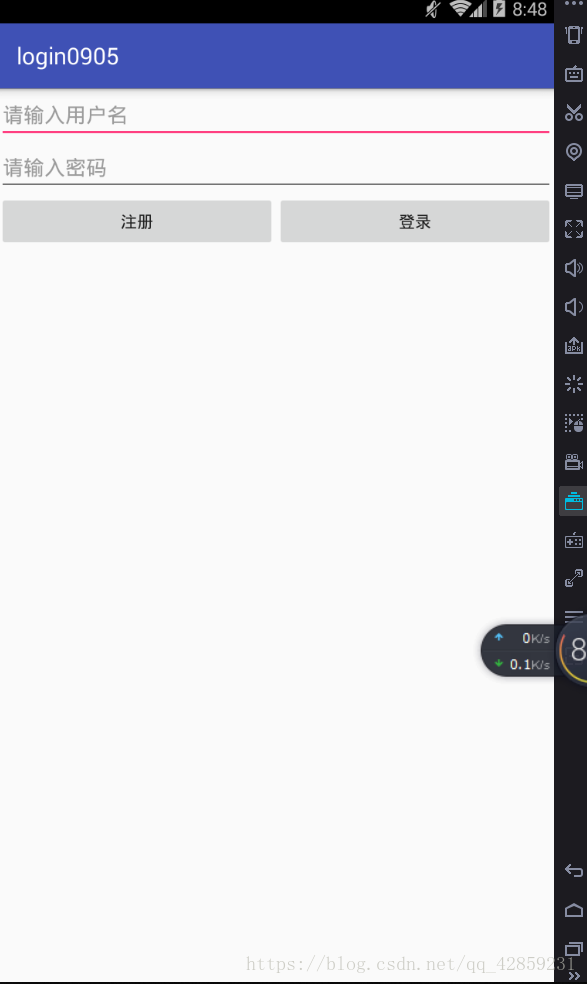
=======================MainAcitvity===================
package com.example.login0905;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import org.xutils.view.annotation.Event;
import org.xutils.view.annotation.ViewInject;
import org.xutils.x;
public class MainActivity extends AppCompatActivity implements accountView{
private accountPresenter presenter;
private String mobile,pwd;
@ViewInject(R.id.name)
private EditText name;
@ViewInject(R.id.password)
private EditText password;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
x.view().inject(this);
presenter = new accountPresenter(this);
}
@Event({R.id.register,R.id.login})
private void accountCallback(View view){
init();
switch (view.getId()){
case R.id.register:
if(TextUtils.isEmpty(mobile)||TextUtils.isEmpty(pwd)){
Toast.makeText(this,"注册失败",Toast.LENGTH_SHORT).show();
}else{
presenter.register(mobile,pwd);
}
break;
case R.id.login:
if(TextUtils.isEmpty(mobile)||TextUtils.isEmpty(pwd)){
Toast.makeText(this,"登录失败",Toast.LENGTH_SHORT).show();
Intent intent = new Intent(MainActivity.this, ShowAcitvity.class);
startActivity(intent);
}else{
presenter.login(mobile,pwd);
}
break;
}
}
private void init() {
mobile = name.getText().toString();
pwd = password.getText().toString();
}
@Override
public void ShowSuccess(final String msg) {
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(MainActivity.this,msg,Toast.LENGTH_SHORT).show();
}
});
}
@Override
public void ShowError(final String msg) {
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(MainActivity.this,msg,Toast.LENGTH_SHORT).show();
}
});
}
}
====================app-==============================
package com.example.login0905;
import android.app.Application;
import org.xutils.x;
/**
* Created by Dell on 2018/9/7.
*/
public class app extends Application{
@Override
public void onCreate() {
super.onCreate();
intie();
}
private void intie() {
x.Ext.init(this);
}
}
==================accountpresenter==============
package com.example.login0905;
import com.example.login0905.accountModel;
import com.example.login0905.accountView;
/**
* Created by Dell on 2018/9/7.
*/
public class accountPresenter {
private accountView view;
private accountModel model;
public accountPresenter(accountView view) {
this.view = view;
model = new accountModel();
}
public void register(String mobile, String pwd) {
model.register(mobile,pwd,new accountModel.accountCallback(){
@Override
public void onScuuess() {
view.ShowSuccess("注册成功");
}
@Override
public void onError(String errormsg) {
view.ShowError("注册失败");
}
});
}
public void login(String mobile, String pwd) {
model.login(mobile,pwd,new accountModel.accountCallback(){
@Override
public void onScuuess() {
view.ShowSuccess("登录成功");
}
@Override
public void onError(String errormsg) {
view.ShowError("登录失败");
}
});
}
}
==================================moudle=====================
package com.example.login0905;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
/**
* Created by Dell on 2018/9/7.
*/
public class accountModel {
private OkHttpClient client;
public accountModel() {
client = new OkHttpClient.Builder()
.connectTimeout(30, TimeUnit.SECONDS)
.readTimeout(30,TimeUnit.SECONDS)
.writeTimeout(30,TimeUnit.SECONDS)
.build();
}
public void register(String mobile, String pwd, final accountCallback callback) {
FormBody body = new FormBody.Builder()
.add("name",mobile)
.add("pwd",pwd)
.build();
Request build = new Request.Builder()
.url("https://www.zhaoapi.cn/user/reg")
.post(body)
.build();
client.newCall(build).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
callback.onError(e.getMessage());
}
@Override
public void onResponse(Call call, Response response) throws IOException {
callback.onScuuess();
}
});
/* client.newCall(build).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
callback.onError(e.getMessage());
}
@Override
public void onResponse(Call call, Response response) throws IOException {
callback.onScuuess();
}
});*/
}
public void login(String mobile, String pwd, final accountCallback callback) {
FormBody body = new FormBody.Builder()
.add("name",mobile)
.add("pwd",pwd)
.build();
Request build = new Request.Builder()
.url("https://www.zhaoapi.cn/user/login")
.post(body)
.build();
client.newCall(build).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
callback.onError(e.getMessage());
}
@Override
public void onResponse(Call call, Response response) throws IOException {
callback.onScuuess();
}
});
}
public interface accountCallback{
void onScuuess();
void onError(String errormsg);
}
}
===========================AccountVIew====================
package com.example.login0905;
/**
* Created by Dell on 2018/9/7.
*/
public interface accountView {
void ShowSuccess(String msg);
void ShowError(String msg);
}
=====================布局===============
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.login0905.MainActivity">
<EditText
android:id="@+id/name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入用户名"/>
<EditText
android:id="@+id/password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入密码"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/register"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="注册"/>
<Button
android:id="@+id/login"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="登录"/>
</LinearLayout>
</LinearLayout>
Mvp---------------------------app
猜你喜欢
转载自blog.csdn.net/qq_42859231/article/details/82562147
今日推荐
周排行