版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/qq_28484355/article/details/79181899
上一篇博客中,讲解了如何通过python+youtube-dl+ffmpeg去下载YouTube上的1080p及以上视频
python——下载youtube上的1080p或4k视频(python+youtube-dl+ffmpeg)
这一篇,我们通过编写一段简单的代码,来完成批下载视频的操作。
代码如下:
# coding:utf8
import subprocess
import time
import os
# list.txt路径
PATH = os.path.dirname(os.path.abspath(__file__))+'/list.txt'
# 查询视频信息语句
COMMAND_PREFIX_CHECK = 'youtube-dl -F '
# 下载1080p视频语句
COMMAND_PREFIX_DOWNLOAD = 'youtube-dl -f 137+140 '
# 传入url,加载相应的1080p视频
def download_by_url(url):
p = subprocess.Popen(COMMAND_PREFIX_DOWNLOAD + url, shell=True, stdout=subprocess.PIPE, stderr=subprocess.STDOUT)
start = time.time()
print "********\tStart download:" + url + "\t" + time.strftime('%Y-%m-%d %H:%M:%S', time.localtime(start))
while True:
line = p.stdout.readline()
if not line == '':
print line.strip('\n')
else:
break
p.wait()
end = time.time()
print "********\tEnd\t"+time.strftime('%Y-%m-%d %H:%M:%S', time.localtime(end)),
print "taking:"+str(int(end-start))+" seconds"
mark_downloaded_url(url)
# 从list.txt中读取一条未下载过的url
def get_url_from_list():
print "\n********\tGet url"
f = open(PATH, 'r+')
for line in f.readlines():
if not line == '\n':
if line[0] == 'h':
return line.strip('\n')
return ''
# 标记已经下载过的url(url前面加上*)
def mark_downloaded_url(url):
output = []
f = open(PATH, 'r+')
i = 0
for line in f.readlines():
line = line.strip('\n')
url = url.strip('\n')
if line == url:
line = "*" + line
output.append(line+"\n")
i = i + 1
f.close()
f = open(PATH, 'w+')
f.writelines(output)
f.close()
if __name__ == '__main__':
# 检查list.txt文件是否存在
if not os.path.exists(PATH):
print "can't find "+PATH
exit(0)
while True:
# 若想暂停下载,在同级目录下建一个stop.txt
if os.path.exists(os.path.dirname(os.path.abspath(__file__))+'/stop.txt'):
exit(0)
url = get_url_from_list()
print "\t\t\tRead:" + url
if url == '':
print "FINISH DOWNLOAD"
exit(0)
download_by_url(url)
注:基于python2.7
代码很简单,稍微了解python的应该都能够看懂。
进行批下载视频操作的话,我们只需要把youtube_mv_download.py下载到本地,在同级目录下新建一个list.txt,将我们需要下载的YouTube的视频网址拷贝进去即可,如下:
https://www.youtube.com/watch?v=ppOWR7ZLl7Q
https://www.youtube.com/watch?v=kRj4toENrnA
https://www.youtube.com/watch?v=4tBnF46ybZk
然后,执行:python youtube_mv_downlaod.py 即可,下载完成后,视频文件就放在同级目录下。
注:1、命令行有实时日志输出,供我们查看下载的进度;
2、此外,每下载好一个视频,程序会将list.txt标记为*,即为已下载。
扫描二维码关注公众号,回复:
3383177 查看本文章
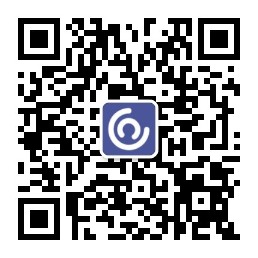
3、若想终止下载,可以在同级目录下新建一个stop.txt,程序便会在下载完当前视频后停止下载。