版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/zhaohaibo_/article/details/81809783
这篇文章所述内容均为瞎弄! 仅仅为了简单地熟悉像素处理的基本方法方法
做的是14年的建模A题嫦娥登陆。
其中一个问题是这样的
如何选择下面这个图(月球表面高度2400米处的照片)的嫦娥三号着陆点?
其实官方给的数据里有图像中每个点的高度,但我不想使用。(好吧其实是懒得下载matlab所以打不开)。如果有每个点的水平高度的话,通过高度分析一波坡度值应该不是什么难事。
这里仅考虑图像的处理方面。
1. 读取图像
from scipy import misc
from scipy.misc import imread, imresize, imsave
import os
import seaborn as sns
import matplotlib.pyplot as plt
os.chdir('/Users/zhaohaibo/Desktop')
# 读取图像
pic = imread('pic2400.jpg')
pic.shape # (2300,2300)
pic.max() # 218
pic.min() # 0
pic.dtype # dtype('uint8')
# 使用sbs的palette变成一个带有颜色的图(目的?没目的)
sns.set()
sns.set_style("white")
pic2 = plt.imshow(pic)
plt.savefig('24003.jpg')
2.使用K-Means聚类
- img = cv2.imread(‘图片’)之后,直接就可以查看每个点的像素值
- 聚为8类,想象中大致就可以把平地那一类选出来了吧
import numpy as np
import cv2
from scipy import misc
from scipy.misc import imread, imresize, imsave
import os
import seaborn as sns
import matplotlib.pyplot as pl
os.chdir('/Users/zhaohaibo/Desktop')
#对rgb图像kmeans聚类
img = cv2.imread('24003.jpg')
Z = img.reshape((-1,3))
# convert to np.float32
Z = np.float32(Z)
# define criteria, number of clusters(K) and apply kmeans()
criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 10, 1.0)
K = 8
ret,label,center=cv2.kmeans(Z,K,None,criteria,10,cv2.KMEANS_RANDOM_CENTERS)
# Now convert back into uint8, and make original image
center = np.uint8(center)
res = center[label.flatten()]
res2 = res.reshape((img.shape))
cv2.imwrite('k=8.jpg',res2)
分别是彩色和黑白的聚类结果。
# 划出平地
from PIL import Image
i = 1
j = 1
img = Image.open("k=12.jpg")#读取系统的内照片
print (img.size)#打印图片大小
print (img.getpixel((4,4)))
# 要保留177 22 88
width = img.size[0]#长度
height = img.size[1]#宽度
for i in range(0,width):#遍历所有长度的点
for j in range(0,height):#遍历所有宽度的点
data = (img.getpixel((i,j)))#打印该图片的所有点
print (data)#打印每个像素点的颜色RGBA的值(r,g,b,alpha)
print (data[0])#打印RGBA的r值
if (data[0]>=170 and data[0]<=180):#RGBA的r值大于170,并且g值大于170,并且b值大于170
img.putpixel((i,j),(255,255,255,255))#则这些像素点的颜色改成白色
img = img.convert("RGB")#把图片强制转成RGB
img.save("1.jpg")#保存修改像素点后的图片
扫描二维码关注公众号,回复:
3324056 查看本文章
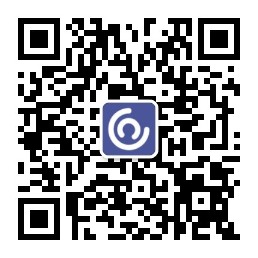
好像有点意思了,看起来平地被标成了白色。
提取rgb数值(想画3D图没成功)
法1
# 提取rgb的数值
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
from matplotlib import cm
from matplotlib.ticker import LinearLocator, FormatStrFormatter
import numpy as np
from PIL import Image
import os
os.chdir("/Users/zhaohaibo/Desktop")
i = 1
j = 1
img = Image.open("k=12.jpg")#读取系统的内照片
print (img.size)#打印图片大小
print (img.getpixel((4,4))) # 获取某点的像素值
# 要保留177 22 88
width = img.size[0]#长度
height = img.size[1]#宽度
x = []
y = []
z = []
for i in range(0,width):#遍历所有长度的点
for j in range(0,height):#遍历所有宽度的点
data = (img.getpixel((i,j)))
x.append(data[0])
y.append(data[1])
z.append(data[2])
x = np.array(x)
y = np.array(y)
z = np.array(z)
x = x.reshape(214,215)
y = y.reshape(214,215)
z = z.reshape(214,215)
网上找了另一个简单的方法
image_path = "HOPE.png"
from mpl_toolkits.mplot3d import Axes3D
from matplotlib import pyplot
from PIL import Image
fig = pyplot.figure()
axis = fig.add_subplot(1, 1, 1, projection="3d") # 3D plot with scalar values in each axis
im = Image.open(image_path)
r, g, b = list(im.getdata(0)), list(im.getdata(1)), list(im.getdata(2))
axis.scatter(r, g, b, c="#ff0000", marker="o")
axis.set_xlabel("Red")
axis.set_ylabel("Green")
axis.set_zlabel("Blue")
pyplot.show()
选择最佳着陆点
# 提取rgb的数值
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
from matplotlib import cm
from matplotlib.ticker import LinearLocator, FormatStrFormatter
import numpy as np
from PIL import Image
import os
os.chdir("/Users/zhaohaibo/Desktop")
img = Image.open("face.jpg")
width = img.size[0]#长度
height = img.size[1]#宽度
def pi(i,j):
return img.getpixel((i,j))[0]
for i in range(6, height-6):
for j in range(6, width-6):
if(pi(i,j)==255 and pi(i+5,j+5)==255 and pi(i-5,j-5)==255 and pi(i-5,j+5)==255 and pi(i+5,j-5)==255):
img.putpixel((i,j),(0,255,0,255))
img = img.convert("RGB")
def check_pi(i,j):
if(img.getpixel((i,j))==(0,255,0)):
return True
else:
return False
for i in range(2, height-2):
for j in range(2, width-2):
if(check_pi(i,j) and check_pi(i-2,j-2) and check_pi(i-2,j+2) and check_pi(i+2,j-2) and check_pi(i+2,j+2)):
img.putpixel((i,j),(0,0,255,255))
area = []
for i in range(2,height-2):
for j in range(2,width-2):
if(img.getpixel((i,j))==(0,0,255)):
area.append((i,j))
for i in range(len(area)-6):
if(area[i-7][0]==area[i+6][0]):
print((area[i]))
(167, 48)
所以一些绿色点是好的着陆区域?绿色点里面蓝色点是好的着陆点?