今天做一个安卓项目是涉及到了在安卓平台中读取以及编辑word文档。开始搞了很久没怎么弄清楚,不过,最后,还是搞出来了。
首先,需要导入必要的第三方jar包:
第三方poi jar包下载
下载了jar包之后将jar包导入进项目中,如图:
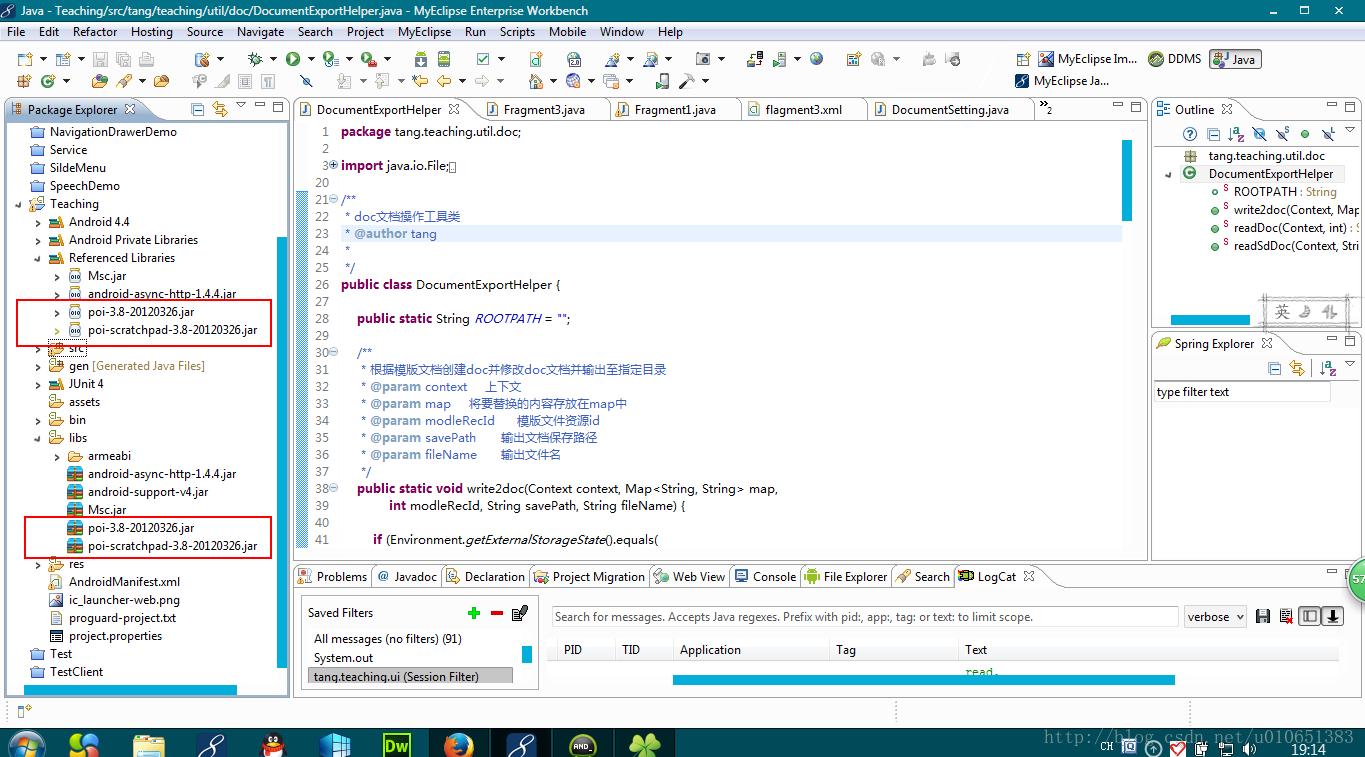
首先将如上图两个poi包复制到libs目录下,然后【项目——>右键——>build path——>Add to build path】;
接下来便是怎么使用这些工具类操作word文档了:
这个word文档模版需要放在rec目录下的raw文件夹下(默认是没有这个文件夹的,可以自己新建)。这个word文档模版的格式如下图:
大家可以看到,这个模版中出现了一堆看不懂的东西,例如${title},是一个占位符,用于在程序中替换文档的内容,在${}之中没有固定的格式,有一定的意义就行了,起到的就是想sql语句中的占位符的作用。
那么我们要如何使用这个工具类呢?
首先
这些是一些用来表示上面所说的占位符的常量。
然后
在以上代码中,通过map将需要插入word文档的内容封装在里面,在这个map集合中,键为以上所说的占位符,而值便是你要插入wod文档的内容。然后调用一下上面给出的工具类中的write2doc方法就搞定了!
首先,需要导入必要的第三方jar包:
第三方poi jar包下载
下载了jar包之后将jar包导入进项目中,如图:
首先将如上图两个poi包复制到libs目录下,然后【项目——>右键——>build path——>Add to build path】;
接下来便是怎么使用这些工具类操作word文档了:
package tang.teaching.util.doc;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Map;
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.usermodel.Range;
import tang.teaching.setting.DocumentSetting;
import android.content.Context;
import android.content.res.Resources;
import android.os.Environment;
import android.widget.Toast;
/**
* doc文档操作工具类
* @author tang
*
*/
public class DocumentExportHelper {
public static String ROOTPATH = "";
/**
* 根据模版文档创建doc并修改doc文档并输出至指定目录
* @param context 上下文
* @param map 将要替换的内容存放在map中
* @param modleRecId 模版文件资源id
* @param savePath 输出文档保存路径
* @param fileName 输出文件名
*/
public static void write2doc(Context context, Map<String, String> map,
int modleRecId, String savePath, String fileName) {
if (Environment.getExternalStorageState().equals(
Environment.MEDIA_MOUNTED)) {
//获取内存卡路径
ROOTPATH = Environment.getExternalStorageDirectory()
.getAbsolutePath()+ File.separator;
Resources res = context.getResources();
//获取doc文档模版输入流
InputStream is = res.openRawResource(modleRecId);
try {
//创建doc文档管理对象
HWPFDocument hDocument = new HWPFDocument(is);
//获取文档主体内容
Range range = hDocument.getRange();
//获取页眉区主体内容
Range headerRange = hDocument.getHeaderStoryRange();
//替换页眉区指定文本内容
headerRange.replaceText(DocumentSetting.DOC_NAME, map.get(DocumentSetting.DOC_NAME));
//替换文档内容区主体内容指定区域文本
for (Map.Entry<String, String> entry : map.entrySet()) {
range.replaceText(entry.getKey(), entry.getValue());
}
//创建并输出文档到指定目录
fileName += "_" + map.get(DocumentSetting.TIME) + ".doc";
File file = new File(ROOTPATH + savePath);
if (!file.exists()) {
file.mkdirs();
}
file = new File(ROOTPATH + File.separator + savePath + fileName);
if (!file.exists()) {
file.createNewFile();
}
FileOutputStream fis = new FileOutputStream(file);
hDocument.write(fis);
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println("SDCard不可用");
}
}
/**
* 根据资源id读取模版文档的主体内容
* @param context 上下文
* @param modleRecId 模版文件资源id
* @return
*/
public static String readDoc(Context context, int modleRecId) {
String docContent = "";
Resources res = context.getResources();
InputStream is = res.openRawResource(modleRecId);
try {
HWPFDocument hDocument = new HWPFDocument(is);
Range range = hDocument.getRange();
docContent = range.text();
} catch (IOException e) {
e.printStackTrace();
}
return docContent;
}
/**
* 根据指定路径读取sd卡中的doc文档中的文本内容
* @param context 上下文
* @param path doc文档存储路径
* @return
* @throws FileNotFoundException
*/
public static String readSdDoc(Context context, String path)
throws FileNotFoundException {
String docContent = "";
File file = new File(path);
if (file.exists()) {
InputStream is = new FileInputStream(file);
try {
HWPFDocument hDocument = new HWPFDocument(is);
Range range = hDocument.getRange();
docContent = range.text();
} catch (IOException e) {
e.printStackTrace();
}
} else {
Toast.makeText(context, "文件不存在", Toast.LENGTH_LONG).show();
}
return docContent;
}
}
以上为我写的一个对word文档操作的一个工具类,若要对word文档进行操作(我所说的是修改所给word文档模版,并输出修改后的新的word文档),首先需要给出一个word文档的模版,如下图:
这个word文档模版需要放在rec目录下的raw文件夹下(默认是没有这个文件夹的,可以自己新建)。这个word文档模版的格式如下图:
大家可以看到,这个模版中出现了一堆看不懂的东西,例如${title},是一个占位符,用于在程序中替换文档的内容,在${}之中没有固定的格式,有一定的意义就行了,起到的就是想sql语句中的占位符的作用。
那么我们要如何使用这个工具类呢?
首先
这些是一些用来表示上面所说的占位符的常量。
然后
在以上代码中,通过map将需要插入word文档的内容封装在里面,在这个map集合中,键为以上所说的占位符,而值便是你要插入wod文档的内容。然后调用一下上面给出的工具类中的write2doc方法就搞定了!