先上图吧!
代码实现其实很简单:
先创建一个Transform类(用来作为为进制转换的工具)
using System;
namespace Conversion
{
class Transform
{
internal string TenToBinary(long value)//将十进制转换为二进制
{
return Convert.ToString(value, 2);
}
internal string TenToEight(long value)//将十进制转换为八进制
{
return Convert.ToString(value, 8);
}
internal string TenToSixteen(long value)//将十进制转换为十六进制
{
return Convert.ToString(value, 16);
}
internal string BinaryToEight(long value)//将二进制转换为八进制
{
return Convert.ToString(
Convert.ToInt64(value.ToString(), 2), 8);
}
internal string BinaryToTen(long value)//将二进制转换为十进制
{
return Convert.ToInt64(
value.ToString(), 2).ToString();
}
internal string BinaryToSixteen(long value)//将二进制转换为十六进制
{
return Convert.ToString(
Convert.ToInt64(value.ToString(), 2), 16);
}
internal string EightToBinary(long value)//将八进制转换为二进制
{
return Convert.ToString(
Convert.ToInt64(value.ToString(), 8), 2);
}
internal string EightToTen(long value)//将八进制转换为十进制
{
return Convert.ToInt64(
value.ToString(), 8).ToString();
}
internal string EightToSixteen(long value)//将八进制转换为十六进制
{
return Convert.ToString(
Convert.ToInt64(value.ToString(), 8), 16);
}
internal string SixteenToBinary(string value)//将十六进制转换为二进制
{
return Convert.ToString(
Convert.ToInt64(value, 16), 2);
}
internal string SixteenToEight(string value)//将十六进制转换为八进制
{
return Convert.ToString(
Convert.ToInt64(value, 16), 8);
}
internal string SixteenToTen(string value)//将十六进制转换为十进制
{
return Convert.ToUInt64(value, 16).ToString();
}
}
}
然后在MainActivity中实现功能:
using Android.App;
using Android.Widget;
using Android.OS;
using System;
namespace Conversion
{
[Activity(Label = "Conversion", MainLauncher = true,Icon ="@drawable/book")]
public class MainActivity : Activity
{
Button btnTransform;
private EditText edtInput,edtOutput;
RadioButton twoC,eightC,sixteenC, twoC2, eightC2, sixteenC2,tenC,tenC2;
Spinner spwhere;
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.Main);
edtInput = FindViewById<EditText>(Resource.Id.edtinput);
edtOutput = FindViewById<EditText>(Resource.Id.edtoutput);
btnTransform = FindViewById<Button>(Resource.Id.btnTransform);
twoC = FindViewById<RadioButton>(Resource.Id.twoC);
eightC = FindViewById<RadioButton>(Resource.Id.eightC);
sixteenC = FindViewById<RadioButton>(Resource.Id.sixteenC);
twoC2 = FindViewById<RadioButton>(Resource.Id.twoC2);
eightC2 = FindViewById<RadioButton>(Resource.Id.eightC2);
sixteenC2 = FindViewById<RadioButton>(Resource.Id.sixteenC2);
tenC = FindViewById<RadioButton>(Resource.Id.tenC);
tenC2 = FindViewById<RadioButton>(Resource.Id.tenC2);
var comments = FindViewById<EditText>(Resource.Id.edtinput);
var comments2 = FindViewById<EditText>(Resource.Id.edtoutput);
//选择条
btnTransform.Click += ButtenTransform;
comments.Click += delegate
{
comments.Text = "";
};
comments2.Click += delegate
{
comments2.Text = "";
};
}
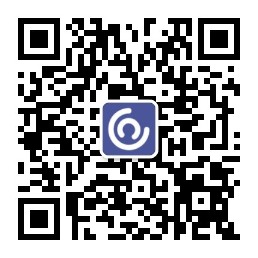
private void ButtenTransform(object sender,EventArgs args)
{
try
{
Action();//调用Action方法进行转换操作
}
catch (Exception)
{
Toast.MakeText(this, "输入错误请重试,错误", ToastLength.Short).Show();
}
}
/// <summary>
/// 进制转换
/// </summary>
private void Action()
{
if (!sixteenC.Checked)
{
long p_line_value;
if (long.TryParse(edtInput.Text, out p_line_value))//判断输入的是否是正确的赋值
{
if (tenC.Checked)
{
if (tenC2.Checked)
{
edtOutput.Text = edtInput.Text;
}
else if (twoC2.Checked)
{
edtOutput.Text = new Transform().TenToBinary(long.Parse(edtInput.Text));
}
else if (eightC2.Checked)
{
edtOutput.Text = new Transform().TenToEight(long.Parse(edtInput.Text));
}
else if(sixteenC2.Checked)
{
edtOutput.Text = new Transform().TenToSixteen(long.Parse(edtInput.Text));
}
else
{
Toast.MakeText(this, "输入错误,重试!", ToastLength.Short).Show();
}
}
else if (twoC.Checked)
{
if (tenC2.Checked)
{
edtOutput.Text = new Transform().BinaryToTen(long.Parse(edtInput.Text));
}
else if (twoC2.Checked)
{
edtOutput.Text = edtInput.Text;
}
else if (eightC2.Checked)
{
edtOutput.Text = new Transform().BinaryToEight(long.Parse(edtInput.Text));
}
else if (sixteenC2.Checked)
{
edtOutput.Text = new Transform().BinaryToSixteen(long.Parse(edtInput.Text));
}
else
{
Toast.MakeText(this, "输入错误,重试!", ToastLength.Short).Show();
}
}
else if(eightC.Checked)
{
if (tenC2.Checked)
{
edtOutput.Text = new Transform().EightToTen(long.Parse(edtInput.Text));
}
else if (twoC2.Checked)
{
edtOutput.Text = new Transform().EightToBinary(long.Parse(edtInput.Text));
}
else if (eightC2.Checked)
{
edtOutput.Text = edtInput.Text;
}
else if (sixteenC2.Checked)
{
edtOutput.Text = new Transform().EightToSixteen(long.Parse(edtInput.Text));
}
else
{
Toast.MakeText(this, "输入错误,重试!", ToastLength.Short).Show();
}
}
}
}
else
{
if (tenC2.Checked)
{
edtOutput.Text = new Transform().SixteenToTen(edtInput.Text);
}
else if (twoC2.Checked)
{
edtOutput.Text = new Transform().SixteenToBinary(edtInput.Text);
}
else if (eightC2.Checked)
{
edtOutput.Text = new Transform().SixteenToEight(edtInput.Text);
}
else if (sixteenC2.Checked)
{
edtOutput.Text = edtInput.Text;
}
else
{
Toast.MakeText(this, "输入错误,重试!", ToastLength.Short).Show();
}
}
}
}
}
布局代码送上:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:orientation="horizontal"
android:minWidth="25px"
android:minHeight="80px"
android:layout_marginTop="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/linearLayoutForName">
<TextView
android:text="输入:"
android:layout_width="81.5dp"
android:layout_height="match_parent"
android:id="@+id/textViewNme"
android:textAllCaps="true"
android:textSize="20dp"
android:gravity="center" />
<EditText
android:layout_width="291.0dp"
android:layout_height="match_parent"
android:id="@+id/edtinput" />
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/linearLayout1"
android:paddingTop="10dp"
android:paddingEnd="20dp"
android:gravity="center">
<RadioGroup
android:minWidth="25px"
android:minHeight="25px"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:id="@+id/radioGroup1"
android:orientation="horizontal">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="十进制"
android:textSize="15dp"
android:id="@+id/tenC" />
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checked="true"
android:text="二进制"
android:textSize="15dp"
android:id="@+id/twoC" />
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="八进制"
android:textSize="15dp"
android:id="@+id/eightC" />
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="十六进制"
android:textSize="15dp"
android:id="@+id/sixteenC" />
</RadioGroup>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:minWidth="25px"
android:minHeight="25px"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/linearLayout2">
<TextView
android:text="输出:"
android:layout_width="81.5dp"
android:layout_height="match_parent"
android:id="@+id/textViewName"
android:textAllCaps="true"
android:textSize="20dp"
android:gravity="center" />
<EditText
android:layout_width="291.0dp"
android:layout_height="match_parent"
android:id="@+id/edtoutput" />
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/linearLayout2"
android:paddingTop="10dp"
android:paddingEnd="20dp"
android:gravity="center">
<RadioGroup
android:minWidth="25px"
android:minHeight="25px"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:id="@+id/radioGroup2"
android:orientation="horizontal">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="十进制"
android:textSize="15dp"
android:id="@+id/tenC2" />
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checked="true"
android:text="二进制"
android:textSize="15dp"
android:id="@+id/twoC2" />
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="八进制"
android:textSize="15dp"
android:id="@+id/eightC2" />
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="十六进制"
android:textSize="15dp"
android:id="@+id/sixteenC2" />
</RadioGroup>
</LinearLayout>
<Button
android:text="转 换"
android:layout_width="match_parent"
android:layout_height="75.0dp"
android:id="@+id/btnTransform"
android:textSize="25dp"
android:layout_marginTop="13.0dp" />
</LinearLayout>