- Android 自带的时间控件常用的有: TextClock,AnalogClock,Chronometer
TextClock( 文本时钟)
- TextClock 是在 Android 4.2(API 17) 后推出的用来替代 DigitalClock 的一个控件!
- TextClock 可以以字符串格式显示当前的日期和时间,因此推荐在 Android 4.2 以后使用 TextClock。
图 1.1
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/GridLayout1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:columnCount="1"
android:orientation="horizontal"
android:rowCount="6">
<TextClock
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="MM/dd/yy h:mmaa" />
<TextClock
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="MMM dd, yyyy h:mmaa" />
<TextClock
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="MMMM dd, yyyy h:mmaa" />
<TextClock
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="E, MMMM dd, yyyy h:mmaa" />
<TextClock
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="EEEE, MMMM dd, yyyy h:mmaa" />
<TextClock
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="Noteworthy day: 'M/d/yy" />
</GridLayout>
Chronometer(计时器)
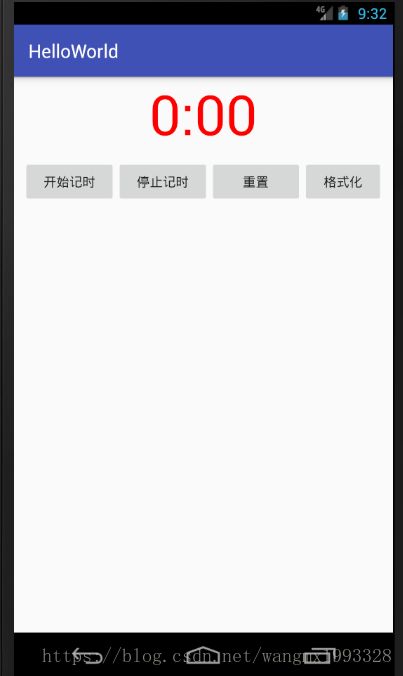
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Chronometer
android:id="@+id/chronometer"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:textColor="#ff0000"
android:textSize="60dip" />
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_margin="10dip"
android:orientation="horizontal">
<Button
android:id="@+id/btnStart"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="开始记时" />
<Button
android:id="@+id/btnStop"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="停止记时" />
<Button
android:id="@+id/btnReset"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="重置" />
<Button
android:id="@+id/btn_format"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="格式化" />
</LinearLayout>
</LinearLayout>
package com.example.administrator.helloworld;
import android.os.Bundle;
import android.os.SystemClock;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.Chronometer;
import android.widget.Toast;
/**
* 一个应用程序可以有1个或多个活动,而没有任何限制。
* 每个为应用程序所定义的活动都需要在 AndroidManifest.xml 中声明,应用的主活动的意图过滤器标签中需要包含 MAIN 动作和 LAUNCHER 类别
* 如果 MAIN 动作还是 LAUNCHER 类别没有在活动中声明,那么应用程序的图标将不会出现在主屏幕的应用列表中。
*
* implements View.OnClickListener 用于为操作按钮绑定单击事件
* implements Chronometer.OnChronometerTickListener 用于为 计时器绑定计时器事件
*/
public class MainActivity extends AppCompatActivity implements View.OnClickListener, Chronometer.OnChronometerTickListener {
/**
* 成员变量
* chronometer:计时器
* btn_start(开始计时按钮)、btn_stop(停止计时按钮)、btn_reset(重置计时按钮)、btn_format(格式化按钮)
*/
private Chronometer chronometer;
private Button btn_start, btn_stop, btn_reset, btn_format;
/**
* 当活动第一次被创建时调用
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/**
* 从项目的 res/layout 中的XML文件加载 UI 组件
* android.util.Log#d(java.lang.String, java.lang.String) 方法用于在 Logcat 窗口中打印日志
*/
setContentView(R.layout.activity_main);
Log.d("Android Message:", "The onCreate() event");
initView();
}
/**
* 初始化 计时器
* 获取布局文件中的控件,然后绑定事件
* <p>
* chronometer(计时器)绑定计时器事件
* btn_start(开始计时按钮)、btn_stop(停止计时按钮)、btn_reset(重置计时按钮)、btn_format(格式化按钮)绑定单击事件
*/
private void initView() {
chronometer = findViewById(R.id.chronometer);
btn_start = findViewById(R.id.btnStart);
btn_stop = findViewById(R.id.btnStop);
btn_reset = findViewById(R.id.btnReset);
btn_format = findViewById(R.id.btn_format);
chronometer.setOnChronometerTickListener(this);
btn_start.setOnClickListener(this);
btn_stop.setOnClickListener(this);
btn_reset.setOnClickListener(this);
btn_format.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btnStart:
chronometer.start();// 开始计时
break;
case R.id.btnStop:
chronometer.stop();// 停止计时
break;
case R.id.btnReset:
chronometer.setBase(SystemClock.elapsedRealtime());// 复位
break;
case R.id.btn_format:
chronometer.setFormat("Time:%s");// 更改时间显示格式
break;
}
}
@Override
public void onChronometerTick(Chronometer chronometer) {
String time = chronometer.getText().toString();
if (time.equals("00:00")) {
Toast.makeText(MainActivity.this, "时间到了~", Toast.LENGTH_SHORT).show();
}
}
}