版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/YIDBoy/article/details/80376745
react+webpack4+typescript搭建项目
公司项目打算从vue框架转成react,所以自学了下如何用webpack4搭建react+typescript的项目,虽说有脚本架create-react-app直接搭建,公司大佬也正在自己封装属于自己的脚本架,但是这里我想从0到1的自己搭建学习搭建一下(适合新手学习下):
一:首先安装最新的webpack
npm install -D webpack
这里我们还需要安装webpack-cli,
(这是webpack4.+的,这里不做详解,有兴趣的可以到webpack官网学习https://webpack.docschina.org/)
npm install -D webpack-cli
接着安装react和相关的依赖react,react-dom
npm install --save-dev react react-dom @types/react @types/react-dom
npm install --save-dev ts-loader
npm link typescript
npm install --save-dev webpack-dev-server
npm install -D "babel-loader@^8.0.0-beta" @babel/core @babel/preset-react
这个时候,我们已经把该装的依赖的都装了(简单版),接下来我们写相关的配置
用命令行输入:
tsc --init
生成typescript的配置文件:tsconfig.json, 这里进行简单的修改
{
"compilerOptions": {
"sourceMap": true,
"noImplicitAny": true,
"module": "commonjs",
"target": "es5",
"jsx": "react"
}
}
接着我们创建src目录,以及在src目录下创建components目录,并且创建文件:
- src/index.tsx 和 src/components/Ant.tsx
index.tsx代码如下:
import * as React from "react"
import * as ReactDOM from "react-dom"
import { Ant } from "./components/Ant"
ReactDOM.render(
<Ant name="EDong" company="ydj" />,
document.getElementById("app")
)
Ant.tsx代码如下:
import * as React from "react"
export interface AntProps {
name: string
company: string
}
export class Ant extends React.Component<AntProps, {}> {
render() {
return (
<h1>
Hello, I am {this.props.name}, I in {this.props.company} now!
</h1>
)
}
}
接着我们在根目录下创建webpack.config.js和index.html:
webpack.config.js配置如下:
const path = require('path')
const CleanWebpackPlugin = require('clean-webpack-plugin')
const HtmlWebpackPlugin = require('html-webpack-plugin')
module.exports = {
mode: 'development',
//入口文件的路径
entry: "./src/index.tsx",
output: {
//打包的输出路径
path: path.resolve(__dirname, "dist"),
filename: "bundle.js"
},
// 添加需要解析的文件格式
resolve: {
extensions: ['.ts', '.tsx', '.js', '.json']
},
plugins: [
new CleanWebpackPlugin(['dist']),
new HtmlWebpackPlugin({
title: '蚂蚁',
template: './index.html',
})
],
module: {
rules: [
{
test: /\.js$/,
include: [
path.resolve(__dirname, 'src')
],
loader: 'babel-loader',
options: {
presets: ['@babel/preset-react'],
plugins: ['@babel/plugin-proposal-class-properties']
}
},
{
test: /\.tsx?$/,
use: ['ts-loader']
}
]
},
devServer: {
contentBase: path.resolve(__dirname, "dist"),
},
// 启用sourceMap
devtool: "source-map",
}
index.html代码如下:
扫描二维码关注公众号,回复:
3242002 查看本文章
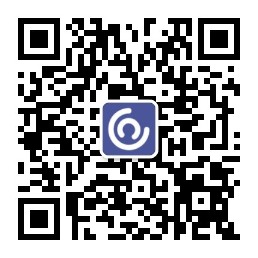
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Hello Ant</title>
</head>
<body>
<div id="app"></div>
</body>
</html>
接着直接运行webpack-dev-server, 在浏览器直接访问localhost:8080,这里已经配置了实时更新了。
简单版的我已经上传到我的github:
有兴趣的可以自己配置react-router, redux等等全家桶。