spring cloud ribbon with eureka
introduction
在上一篇中阐述了ribbon
的基本用法,但是可以发现服务列表是通过配置得来的,实际
情况通常是由负载均衡+服务发现来实现的,通过服务发现获取服务列表,负载均衡通过rule选择要调用的服务。服务发现可以通过eureka
来实现,后期会讲解利用consul
做服务发现。
eureka discovery service
eureka服务发现在前面的文章中已经提供到,这里直接给出代码
首先是 eureka server
package com.lkl.springcloud.ribbon;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableAutoConfiguration
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
new SpringApplicationBuilder(EurekaServerApplication.class).properties(
"spring.config.name:eureka", "logging.level.com.netflix.discovery:OFF")
.run(args);
}
}
对应配置文件:
server.port=8761
spring.application.name= eureka
eureka.client.registerWithEureka=false
eureka.client.fetchRegistry=false
在eureka上进行服务注册SimpleApplication.java
package com.lkl.springcloud.ribbon;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@Configuration
@EnableAutoConfiguration
@EnableDiscoveryClient
@RestController
public class SimpleApplication {
@RequestMapping("/")
public String hello() {
return "Hello";
}
public static void main(String[] args) {
new SpringApplicationBuilder(SimpleApplication.class).properties(
"spring.config.name:simple").run(args);
}
}
对应client配置
server.port= 8081
spring.application.name= simple
eureka.instance.leaseRenewalIntervalInSeconds= 10
eureka.client.registryFetchIntervalSeconds= 5
eureka.client.serviceUrl.defaultZone= http://localhost:${eureka.port:8761}/eureka/
ribbon + eureka
Application.java
package com.lkl.springcloud.ribbon;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.context.annotation.Bean;
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
/**
* Created by liaokailin on 16/5/9.
*/
@SpringBootApplication
@EnableDiscoveryClient
@RestController
public class Application {
@Autowired
RestTemplate client;
/**
* LoadBalanced 注解表明restTemplate使用LoadBalancerClient执行请求
*
* @return
*/
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
RestTemplate template = new RestTemplate();
SimpleClientHttpRequestFactory factory = (SimpleClientHttpRequestFactory) template.getRequestFactory();
factory.setConnectTimeout(3000);
factory.setReadTimeout(3000);
return template;
}
@RequestMapping("/")
public String helloWorld() {
return client.getForObject("http://simple/", String.class);
}
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
EnableDiscoveryClient
表明为eureka client 通过可以进行服务发现
RestTemplate
构建RestTemplate
对应的bean,在method上使用注解LoadBalanced
表示restTemplate使用LoadBalancerClient
执行请求
对应配置文件
spring.application.name=RibbionClient
eureka.instance.leaseRenewalIntervalInSeconds= 10
eureka.client.registryFetchIntervalSeconds= 5
eureka.client.serviceUrl.defaultZone= http://localhost:${eureka.port:8761}/eureka/
run Application.java
后访问http://localhost:8080/调用helloWorld()
方法,该方法访问http://simple/
其中的simple
为SimpleApplication.java
在eureka上注册的service id,即实际调用
扫描二维码关注公众号,回复:
3181359 查看本文章
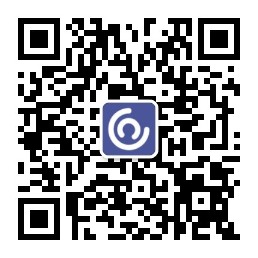
@RequestMapping("/")
public String hello() {
return "Hello";
}
因此得到返回结果Hello
.
ok ~ it’s work ! more about is here
转载请注明
http://blog.csdn.net/liaokailin/article/details/51469834
欢迎关注,您的肯定是对我最大的支持