package saas.framework.cache;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import saas.framework.cache.redis.cmds.RedisCmdsFactory;
import saas.framework.service.SpringContextHolder;
public class RedisCmdHelper {
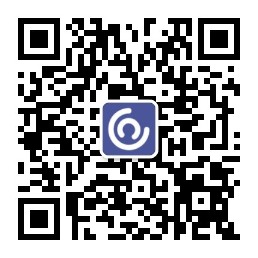
private int db = 3;
private static ThreadLocal<Jedis> jedisPoolCache = new ThreadLocal<Jedis>();
private static final Logger logger = LoggerFactory.getLogger(RedisCmdHelper.class);
private JedisPool jedisPool;
private RedisCmdsFactory redisCmdsFactory;
public RedisCmdHelper() {
this.redisCmdsFactory = SpringContextHolder.getBean("redisCmdsFactory");
}
public void setJedisPool(JedisPool jedisPool) {
this.jedisPool = jedisPool;
}
public void setDb(int db) {
this.db = db;
}
public Object executeCallBack(JedisCallback jedisCallback) {
Jedis jedis = null;
boolean isError = false;
try {
jedis = getJedis();
Object obj = jedisCallback.executCmd(jedis);
return obj;
} catch (Exception ex) {
isError = true;
logger.error("execute cmd error", ex);
return null;
} finally {
if (jedis != null){
returnConnection(jedis, isError);
}
jedisPoolCache.remove();
}
}
public Object doRedisCmd(String cmd, Object key, Object[] params, Object[] extraParam) {
if (redisCmdsFactory == null) {
redisCmdsFactory = SpringContextHolder.getBean("redisCmdsFactory");
}
if (redisCmdsFactory == null) {
logger.error("redisCmdsFactory cant be null");
}
Object result = null;
Jedis jedis = null;
boolean isError = false;
try {
jedis = getJedis();
jedisPoolCache.set(jedis);
IRedisCmd redisCmd = redisCmdsFactory.getRedisCmdByKey(cmd);
if (redisCmd != null) {
result = redisCmd.exeute(key, params, extraParam);
}
} catch (Exception ex) {
logger.error("execute cmd error", ex);
isError = true;
} finally {
if (jedis != null) {
returnConnection(jedis, isError);
}
jedisPoolCache.remove();
}
return result;
}
public Object doRedisCmd(String cmd, Object key, Object[] params) {
return doRedisCmd(cmd, key, params, null);
}
public Object doRedisCmd(String cmd, Object key) {
return doRedisCmd(cmd, key, null, null);
}
private Jedis getJedis() {
Jedis jedis = jedisPool.getResource();
jedis.select(db);
return jedis;
}
private void returnConnection(Jedis jedis, Boolean error) {
if (error) {
jedisPool.returnBrokenResource(jedis);
} else {
jedisPool.returnResource(jedis);
}
}
public static Jedis getCurrentJedis() {
return jedisPoolCache.get();
}
}
----------------------------------------------------------------------------------
/**
* Copyright (c) 2005-2010 springside.org.cn
*
* Licensed under the Apache License, Version 2.0 (the "License");
*
* $Id: SpringContextHolder.java 1211 2010-09-10 16:20:45Z calvinxiu $
*/
package saas.framework.service;
/**
* 以静态变量保存Spring ApplicationContext, 可在任何代码任何地方任何时候中取出ApplicaitonContext.
*
*/
public class SpringContextHolder {
/**
* 从静态变量applicationContext中取得Bean, 自动转型为所赋值对象的类型.
*/
@SuppressWarnings("unchecked")
public static <T> T getBean(String name) {
return (T) ServiceLocator.getService(name);
}
public static <T> T getBean(Class<T> interfaceClass) {
return (T) ServiceLocator.getService(interfaceClass);
}
}
----------------------------------------------------------------------------------
package saas.framework.service;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Component;
/**
*star
*/
@Component
public class ServiceLocatorInitializer implements ApplicationContextAware {
/* (non-Javadoc)
* @see org.springframework.context.ApplicationContextAware#setApplicationContext(org.springframework.context.ApplicationContext)
*/
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
ServiceLocator.setApplicationContext(applicationContext);
}
}
-----------------------------------------------------------------------------------------------
package saas.framework.service;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.NoSuchBeanDefinitionException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.MessageSource;
public class ServiceLocator {
private static final Logger logger = LoggerFactory.getLogger(ServiceLocator.class);
private static ApplicationContext applicationContext;
/**
* @return Returns the applicationContext.
*/
public static synchronized ApplicationContext getApplicationContext() {
return applicationContext;
}
/**
* @param applicationContext
* The applicationContext to set.
*/
public static synchronized void setApplicationContext(ApplicationContext appContext) {
applicationContext = appContext;
}
/**
* Returns the service that is associated with the interface whose class you
* provide. For right now, that's going to be the bean in the Spring context
* whose name is the class name with the first letter lowercased (e.g.
* SecurityDAO.class looks up a bean called "securityDAO"). Changed this so
* that it just caches the name of the service rather than the service
* itself.
*
* @param interfaceClass
* the class of the interface you wish to return.
* @return the appropriate object, or null if it doesn't exist.
*/
public static <T> T getService(Class<T> interfaceClass) {
if (interfaceClass == null) {
return null;
}
return applicationContext.getBean(interfaceClass);
}
/**
* Returns the service corresponding to the name you request. This is
* private now because we want to force people to get beans according to the
* interface, and not let them ask for any old bean.
*
* @param serviceName
* the name of the service to obtain.
* @return the appropriate service object, or null if none can be found.
*/
public static Object getService(String serviceName) {
try {
if (applicationContext == null) {
return null;
}
Object o = applicationContext.getBean(serviceName);
return o;
} catch (NoSuchBeanDefinitionException e) {
logger.warn("No bean found with name:" + serviceName);
return null;
}
}
/**
*
* Returns the service of particular type
*
* @param serviceName
* @param requiredType
* @return
*/
public static <T> T getService(String serviceName, Class<T> requiredType) {
if (applicationContext == null) {
return null;
}
return applicationContext.getBean(serviceName, requiredType);
}
public static MessageSource getMessageSource() {
return getApplicationContext();
}
}
-------------------------------------------------------------------------------------
package saas.framework.cache.redis.cmds;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import org.springframework.stereotype.Component;
import saas.framework.cache.IRedisCmd;
@Component
public class RedisCmdsFactory {
private Map<String, IRedisCmd> cmdMaps=new ConcurrentHashMap<String, IRedisCmd>();
public IRedisCmd getRedisCmdByKey(String key){
return cmdMaps.get(key);
}
public void registCmd(IRedisCmd redisCmd){
cmdMaps.put(redisCmd.getCmdId(), redisCmd);
}
}